Understanding Crossover and Mutation in Genetic Algorithms
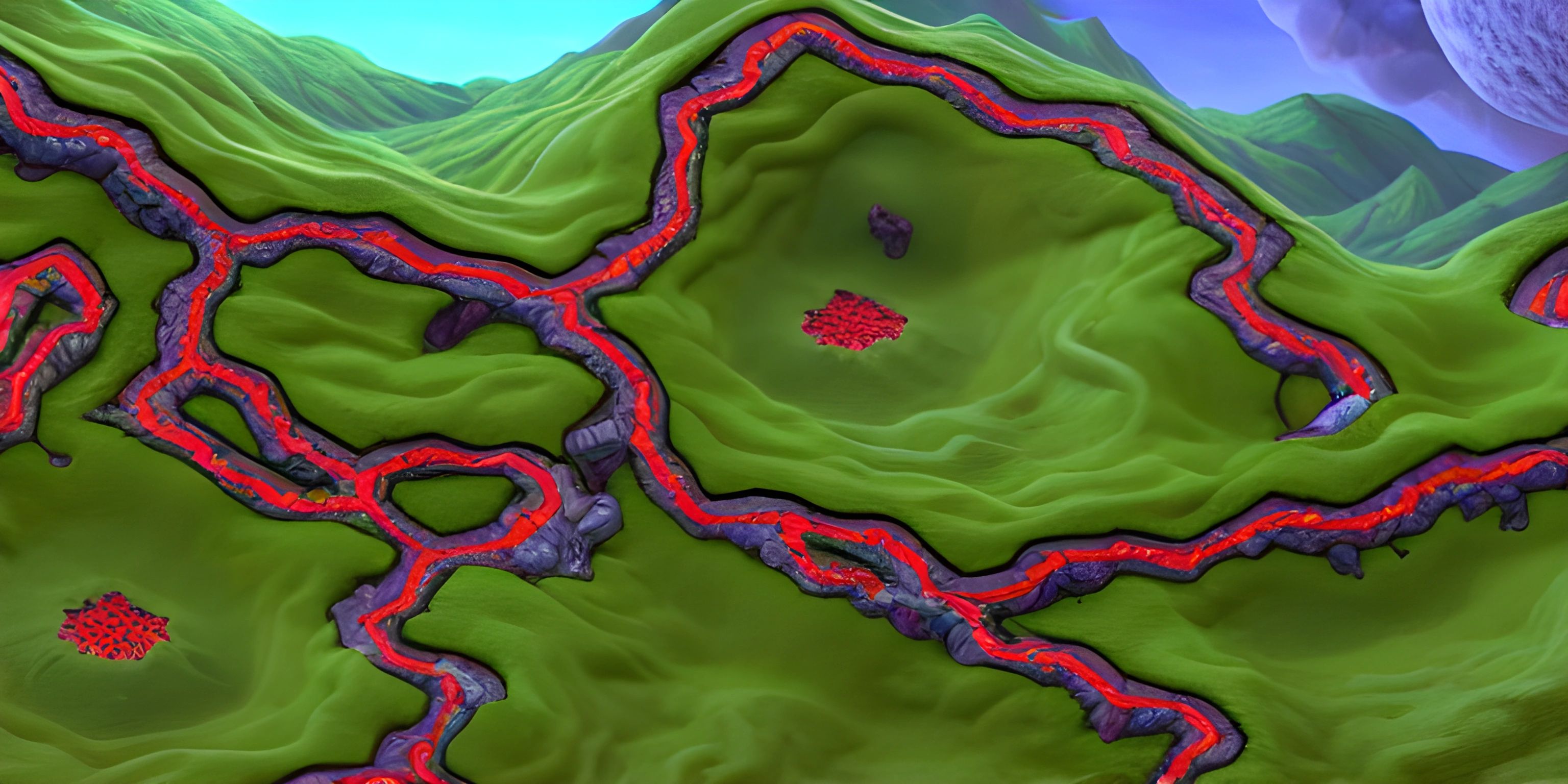
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of genetic algorithms, we take a leaf out of Mother Nature's book. Imagine trying to solve a problem by breeding solutions. Sounds wild, right? Welcome to the jungle!
Genetic Algorithms: A Quick Refresher
Genetic algorithms (GAs) are a class of optimization algorithms inspired by the process of natural selection. Think of them as a way of evolving solutions to problems over generations. Just like in nature, the fittest individuals (solutions) are more likely to survive and reproduce.
Key Components of Genetic Algorithms
- Population: A set of candidate solutions.
- Fitness Function: A way to evaluate how good a solution is.
- Selection: Choosing the best solutions to reproduce.
- Crossover: Combining parts of two solutions to create new ones.
- Mutation: Introducing random changes to solutions.
In this article, we'll focus on the funky duo: crossover and mutation.
The Magic of Crossover
Crossover is like a genetic handshake. Two parent solutions shake hands (exchange parts of their genes) to produce offspring. It’s nature’s way of saying, "Let’s mix things up a bit!"
Single-Point Crossover
The simplest form of crossover is the single-point crossover. Imagine you have two parent solutions:
Parent 1: 10101010
Parent 2: 01010101
Choose a random crossover point, say 4. Swap the tails of the parents:
Offspring 1: 10100101
Offspring 2: 01011010
Boom! We have two new solutions.
Multi-Point Crossover
Why stop at one point? Multi-point crossover uses multiple crossover points, adding more genetic diversity:
Parent 1: 10101010
Parent 2: 01010101
Choose crossover points at 3 and 6:
Offspring 1: 10110100
Offspring 2: 01001011
Uniform Crossover
Uniform crossover treats each gene independently. For each gene, flip a coin to decide which parent it comes from:
Parent 1: 10101010
Parent 2: 01010101
Coin flips: HTTHTHHT
(H for head, T for tail)
Offspring: 11010001
The Spice of Mutation
Mutation is the secret sauce of genetic algorithms. It introduces random changes to offspring, ensuring that the population doesn’t stagnate. The goal is to maintain genetic diversity and explore new parts of the solution space.
Bit-Flip Mutation
In a bit-flip mutation, we randomly choose bits to flip (0 to 1 or 1 to 0):
Original: 10101010
Mutated: 10101110
Swap Mutation
Swap mutation randomly selects two genes and swaps them:
Original: 10101010
Mutated: 10100011
Scramble Mutation
Scramble mutation randomly scrambles a subset of genes:
Original: 10101010
Subset: 0101
(positions 2 to 5)
Mutated: 10100110
(subset scrambled)
Why Crossover and Mutation Matter
Imagine a world where everyone’s the same. Boring, right? Crossover and mutation keep our genetic algorithm solutions diverse and adaptable.
Balancing Exploration and Exploitation
Crossover tends to exploit existing solutions by combining good traits. Mutation, on the other hand, explores new possibilities by introducing randomness. A good genetic algorithm strikes a balance between these two forces.
Preventing Premature Convergence
Without mutation, our population might converge too quickly on local optima (suboptimal solutions). Mutation keeps the population dynamic, helping to escape these traps.
Enhancing Diversity
Diversity is the lifeblood of genetic algorithms. Crossover and mutation ensure that the population doesn't fall into a genetic rut.
Putting It All Together
Let's see a Python example of crossover and mutation:
import random def single_point_crossover(parent1, parent2): crossover_point = random.randint(1, len(parent1) - 1) offspring1 = parent1[:crossover_point] + parent2[crossover_point:] offspring2 = parent2[:crossover_point] + parent1[crossover_point:] return offspring1, offspring2 def bit_flip_mutation(individual, mutation_rate): mutated = "" for gene in individual: if random.random() < mutation_rate: mutated += "1" if gene == "0" else "0" else: mutated += gene return mutated # Example usage parent1 = "10101010" parent2 = "01010101" offspring1, offspring2 = single_point_crossover(parent1, parent2) print("Offspring 1:", offspring1) print("Offspring 2:", offspring2) mutation_rate = 0.1 mutated_offspring1 = bit_flip_mutation(offspring1, mutation_rate) print("Mutated Offspring 1:", mutated_offspring1)
This snippet demonstrates single-point crossover and bit-flip mutation.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Async Rust (psst, it's free!).
FAQ
What is the main purpose of crossover in genetic algorithms?
The main purpose of crossover in genetic algorithms is to combine the genetic information of two parent solutions to produce new offspring. This helps to exploit existing solutions by mixing their traits, potentially leading to better solutions.
How does mutation contribute to genetic algorithms?
Mutation introduces random changes to individuals in the population. This maintains genetic diversity, helps prevent premature convergence on local optima, and ensures that the algorithm explores new areas of the solution space.
Can genetic algorithms work without crossover and mutation?
While technically possible, genetic algorithms without crossover and mutation would be much less effective. Crossover and mutation are essential for maintaining diversity and exploring the solution space, which are crucial for finding optimal solutions.
What is a fitness function in genetic algorithms?
A fitness function in genetic algorithms is a way to evaluate how good a solution is. It assigns a fitness score to each individual in the population, guiding the selection process for reproduction.
How do you balance crossover and mutation rates?
Balancing crossover and mutation rates is crucial for the effectiveness of a genetic algorithm. Typically, crossover rates are set high (around 70-90%) to exploit existing solutions, while mutation rates are set low (around 1-5%) to introduce diversity without disrupting good solutions too much. Experimentation and tuning are often necessary to find the optimal balance.