Python: Getting Started
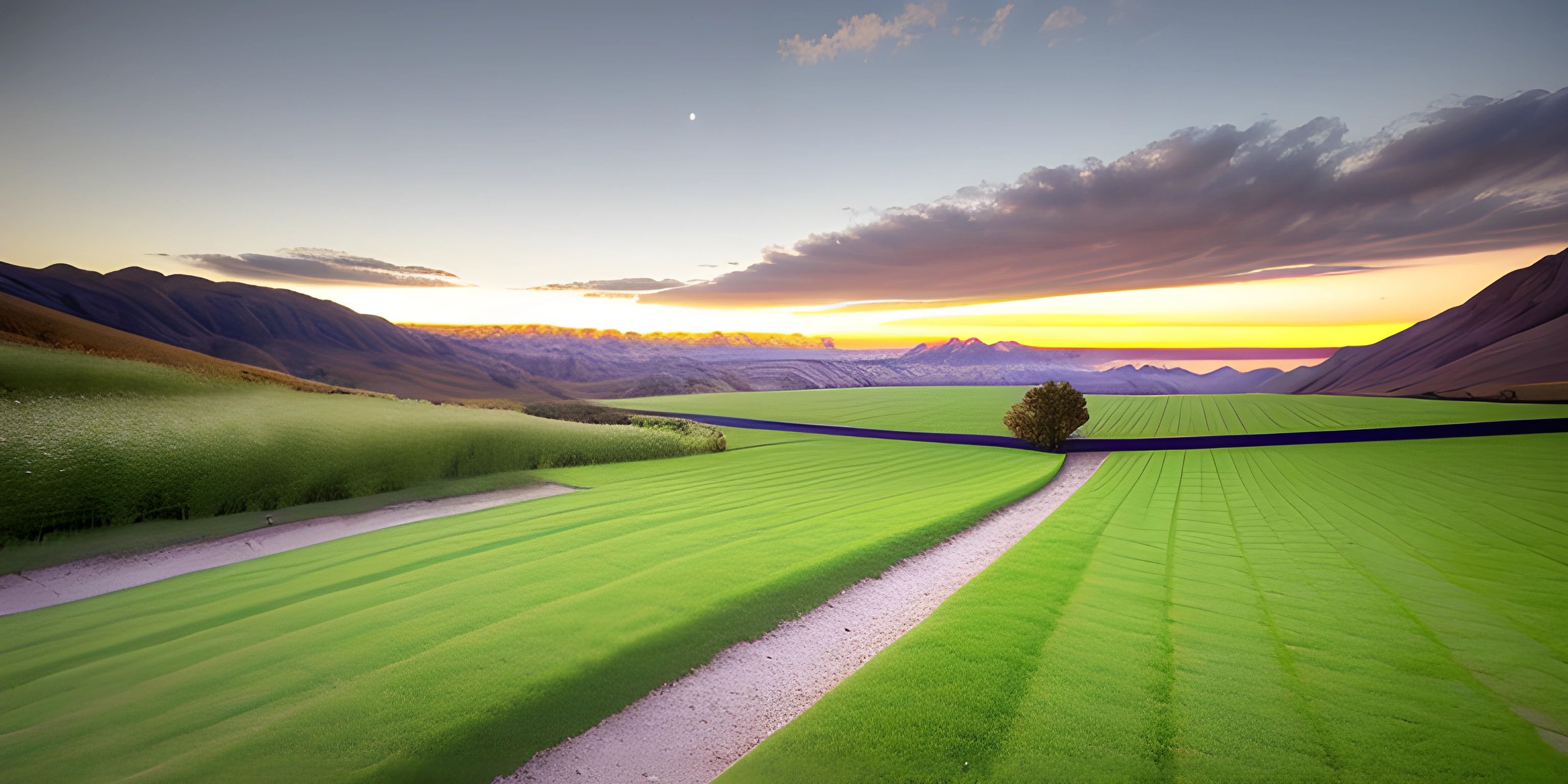
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python is a versatile and beginner-friendly programming language that has gained immense popularity due to its simplicity and readability. If you're new to programming or want to add another language to your arsenal, Python is an excellent choice. In this guide, we'll introduce you to Python, its installation process, and some essential concepts to help you get started.
Why Python?
Python is known for its easy-to-understand syntax and ability to perform complex tasks with minimal code. Its versatility spans various domains, making it a popular choice for web development, data analysis, artificial intelligence (AI), and more. Another advantage is the vast Python community, which offers extensive libraries and frameworks that make development quicker and easier.
Installing Python
Before diving into Python, you need to install it on your computer. Download the latest version of Python from the official website. Follow the installation instructions for your operating system (Windows, macOS, or Linux). To ensure Python is installed correctly, open a terminal or command prompt and type python --version
. You should see the installed version number displayed.
Your First Python Program
Now that Python is installed, let's write a simple program. Open your preferred text editor, type the following line, and save the file as hello_python.py
:
print("Hello, Python!")
To run the program, open a terminal or command prompt, navigate to the directory where you saved the file, and type python hello_python.py
. You should see the message "Hello, Python!" printed in the terminal.
Basic Python Concepts
Here are some foundational concepts you'll need to understand as you start learning Python:
Variables
Variables in Python are used to store data. To create a variable, assign a value to a name using the equal sign (=
). For example:
name = "John" age = 25
Data Types
Python has several data types, such as strings, integers, floats, lists, and dictionaries. You don't need to explicitly declare a variable's data type, as Python infers it based on the assigned value.
string_example = "Hello, World!" integer_example = 42 float_example = 3.14
Control Flow
Control flow statements, such as if, elif, and else, allow you to execute different blocks of code based on conditions.
temperature = 25 if temperature < 0: print("It's freezing!") elif temperature < 20: print("It's chilly!") else: print("It's warm!")
Loops
Python uses for and while loops to repeatedly execute a block of code.
# For loop for i in range(5): print(i) # While loop count = 0 while count < 5: print(count) count += 1
With these basic concepts under your belt, you're well on your way to learning Python. Keep exploring, practicing, and building projects to strengthen your skills.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are some reasons to learn Python?
Python is an excellent choice for beginners due to its simplicity and readability. It is versatile, making it suitable for various domains like web development, data analysis, and artificial intelligence. Python also has a vast community, ensuring extensive libraries and frameworks to aid in development.
How do I install Python?
Download the latest version of Python from the official website (https://www.python.org/downloads/) and follow the installation instructions for your operating system (Windows, macOS, or Linux). To check the installation, open a terminal or command prompt, and type python --version
.
What are the basic Python concepts I need to know?
As you start learning Python, you should understand variables, data types, control flow statements (if, elif, and else), and loops (for and while). These foundational concepts will help you build a strong foundation in Python programming.
How do I run a Python program?
Save your Python code in a file with a .py
extension (e.g., hello_python.py
). Open a terminal or command prompt, navigate to the directory where you saved the file, and type python <filename>.py
to run the program (e.g., python hello_python.py
).