Discord.js Events
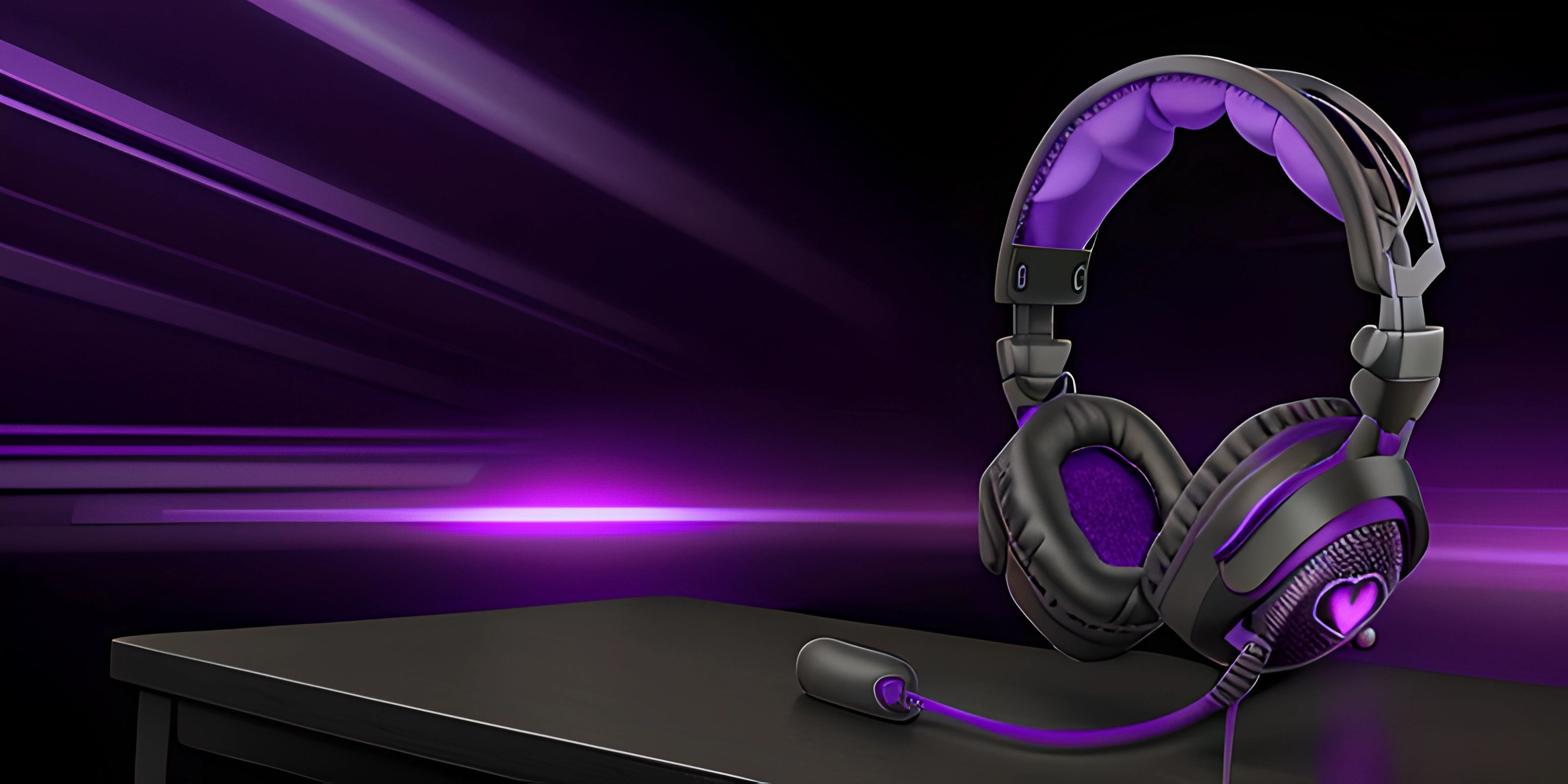
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Events are the lifeblood of any Discord bot, as they enable your bot to listen and react to things happening in the server. In this article, we'll explore how to listen and respond to Discord events using Discord.js, a powerful library for building bots and interacting with the Discord API.
Setting Up the Bot
Before diving into events, make sure you have a basic bot setup with Discord.js. If you need help setting up your bot, check out our article on creating a Discord bot with Discord.js.
Listening for Events
Once your bot is set up, you'll need to listen for events. To do this, you'll use the on
method of your Client
object. This method takes two arguments: the name of the event and a callback function that will be executed whenever the event is triggered.
Here's an example that listens for the "message" event, which is fired when any message is sent in a channel the bot has access to:
const Discord = require("discord.js"); const client = new Discord.Client(); client.on("message", (message) => { console.log(`Received message: ${message.content}`); }); client.login("your-token-here");
In this example, we're using an arrow function as the callback. The function takes a single argument, message
, which is an instance of the Message
class from Discord.js. We then log the content of the message to the console.
Responding to Events
Now that we can listen for events, let's see how we can respond to them. In the case of the "message" event, we might want our bot to reply to certain messages. Let's make the bot reply with "Hello there!" whenever someone says "Hi, bot!":
client.on("message", (message) => { if (message.content === "Hi, bot!") { message.channel.send("Hello there!"); } });
In this example, we added a conditional statement to check if the message content is "Hi, bot!". If so, the bot sends a message to the same channel with the content "Hello there!".
Other Events
Discord.js provides a wide variety of events you can listen for. Some common events include:
ready
: Emitted when the bot is logged in and ready to start working.guildMemberAdd
: Emitted when a new member joins a server.guildMemberRemove
: Emitted when a member leaves a server.voiceStateUpdate
: Emitted when a member changes their voice state, such as joining or leaving a voice channel.
You can find a comprehensive list of events in the Discord.js documentation.
Wrapping Up
Events are essential for creating responsive and interactive Discord bots. With Discord.js, listening and responding to events is straightforward and efficient. Now that you have a basic understanding of how to handle events, you can explore more advanced features and build your own unique bots to enhance your Discord experience. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What are Discord.js events and how do they work?
Discord.js events are actions or occurrences that happen within the Discord platform, such as messages being sent, users joining a server or even users changing their avatars. Discord.js, a powerful library for interacting with the Discord API, allows developers to listen and respond to these events in their custom bots or applications.
How do I listen to an event in Discord.js?
To listen to an event in Discord.js, you'll need to use the on
method on your bot's client object, passing in the event name and a callback function that will be executed when the event occurs. Here's an example of listening to the 'message' event:
const Discord = require("discord.js"); const client = new Discord.Client(); client.on("message", (message) => { // Your code to handle the message event goes here }); client.login("your-bot-token");
Can I listen to multiple events with the same callback function?
Yes, you can listen to multiple events with the same callback function by chaining additional on
methods with the same callback. For example, you can listen to both the 'message' and 'messageUpdate' events like this:
client.on("message", handleMessages) .on("messageUpdate", handleMessages); function handleMessages(message) { // Your code to handle both events goes here }
How can I remove an event listener in Discord.js?
To remove an event listener in Discord.js, you can use the off
method on your bot's client object, passing in the event name and the callback function. Here's an example of removing a listener for the 'message' event:
client.off("message", handleMessages); function handleMessages(message) { // Your code to handle the message event goes here }
Are there any important events I should always listen to in my Discord.js bot?
While the events you listen to will depend on your bot's functionality, some commonly used events include 'ready', 'message', 'guildMemberAdd', and 'guildMemberRemove'. The 'ready' event, in particular, is useful to know when your bot has successfully logged in and is ready to start processing events.