Discord.js Slash Commands
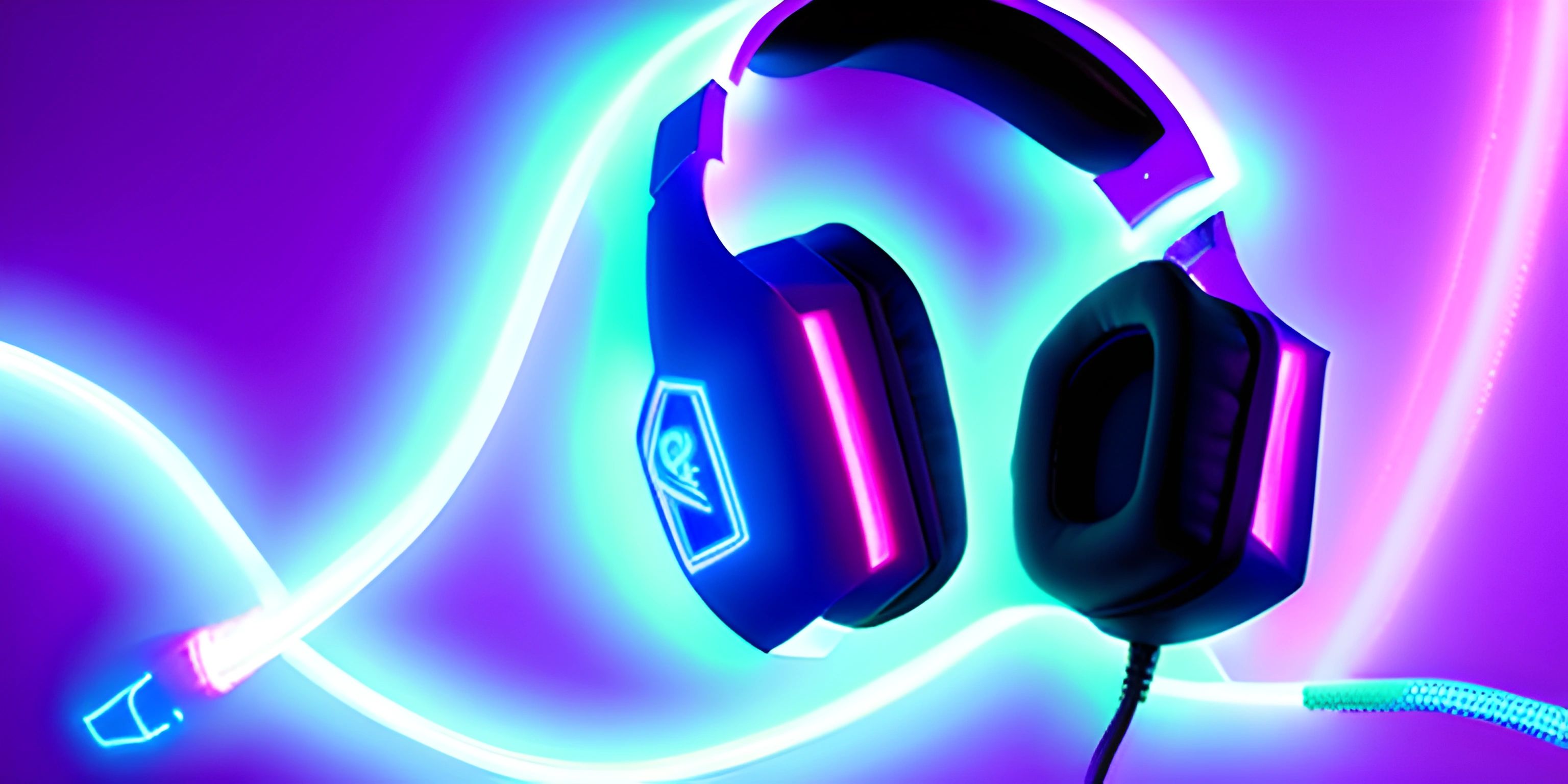
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Slash commands are a new and convenient way of interacting with Discord bots, allowing users to easily access the bot's features by typing commands preceded by a forward slash (/
). They are built using the Discord.js library, a popular choice for creating Discord bots.
Creating Slash Commands
Before we dive into responding to slash commands, let's create one first. In Discord.js, slash commands can be created using the ApplicationCommandManager
class. We'll start by creating a new command called "ping" that will return a simple "pong" response.
First, make sure you have the latest version of Discord.js installed in your project:
npm install discord.js
Next, set up your Discord bot and create an instance of the Client
class:
const { Client } = require("discord.js"); const client = new Client({ intents: ["GUILD_MESSAGES", "GUILD_SLASH_COMMANDS"] }); client.login("your-bot-token");
Now, let's create the "ping" slash command:
client.on("ready", async () => { console.log(`Logged in as ${client.user.tag}`); const pingCommand = { name: "ping", description: "Test the bot's responsiveness with a simple ping command" }; const guild = client.guilds.cache.get("your-guild-id"); await guild.commands.create(pingCommand); });
Replace your-guild-id
with the ID of the server you want to add the slash command to. The "ping" command should now be available in that server.
Responding to Slash Commands
Now that we have the "ping" command set up, let's handle the interaction and respond with a "pong" message.
To do this, we will listen for the interactionCreate
event on our client instance:
client.on("interactionCreate", async (interaction) => { if (!interaction.isCommand()) return; if (interaction.commandName === "ping") { await interaction.reply("Pong!"); } });
The interactionCreate
event is triggered whenever an interaction takes place. We check if the interaction is a command using the isCommand()
method, and then respond to the command by checking its name with the commandName
property. If it's the "ping" command, we reply with "Pong!" using the reply()
method.
That's it! Your Discord bot should now be able to respond to the "ping" slash command. You can create more complex commands and interactions by adding more options, subcommands, and even permission checks. Be sure to explore the Discord.js documentation for more information and examples. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What are slash commands in Discord.js?
Slash commands are a new way to interact with Discord bots. They are commands that start with a forward slash (/
) and provide a more user-friendly experience by displaying a list of available commands and their descriptions. Discord.js is a popular library for building Discord bots, and it allows developers to create and respond to slash commands with ease.
How do I create a slash command in Discord.js?
To create a slash command in Discord.js, you need to define the command using the ApplicationCommand
method. Here's a simple example to create a /ping
command:
const { REST } = require("@discordjs/rest"); const { Routes } = require("discord-api-types/v9"); const clientId = "YOUR_CLIENT_ID"; const guildId = "YOUR_GUILD_ID"; const token = "YOUR_BOT_TOKEN"; const commands = [ { name: "ping", description: "Replies with Pong!" } ]; const rest = new REST({ version: "9" }).setToken(token); (async () => { try { await rest.put( Routes.applicationGuildCommands(clientId, guildId), { body: commands } ); console.log("Successfully registered application commands."); } catch (error) { console.error(error); } })();
Replace YOUR_CLIENT_ID
, YOUR_GUILD_ID
, and YOUR_BOT_TOKEN
with the appropriate values.
How do I respond to a slash command in Discord.js?
To respond to a slash command in Discord.js, you need to listen for the interactionCreate
event and check if the interaction is a command. Here's an example for responding to the /ping
command:
const { Client, Intents } = require("discord.js"); const token = "YOUR_BOT_TOKEN"; const client = new Client({ intents: [Intents.FLAGS.GUILD_MESSAGES, Intents.FLAGS.GUILD_INTEGRATIONS] }); client.on("ready", () => { console.log(`Logged in as ${client.user.tag}!`); }); client.on("interactionCreate", async (interaction) => { if (!interaction.isCommand()) return; const { commandName } = interaction; if (commandName === "ping") { await interaction.reply("Pong!"); } }); client.login(token);
Replace YOUR_BOT_TOKEN
with your bot's token.
Can I create and respond to subcommands in slash commands?
Yes, you can create and respond to subcommands in slash commands using Discord.js. To create a subcommand, you need to add it to the options
array of your command. Here's an example:
{ name: "example", description: "An example command with subcommands", options: [ { name: "subcommand1", description: "The first subcommand", type: "SUB_COMMAND" }, { name: "subcommand2", description: "The second subcommand", type: "SUB_COMMAND" } ] }
To respond to a subcommand, you need to check the options
property of the interaction. Example:
client.on("interactionCreate", async (interaction) => { if (!interaction.isCommand()) return; const { commandName, options } = interaction; if (commandName === "example") { const subcommand = options.getSubcommand(); if (subcommand === "subcommand1") { await interaction.reply("You used Subcommand 1!"); } else if (subcommand === "subcommand2") { await interaction.reply("You used Subcommand 2!"); } } });
How do I update or delete existing slash commands?
To update or delete existing slash commands, you can use the methods provided by the REST
class from the @discordjs/rest
package. To update a command, use the patch
method:
await rest.patch( Routes.applicationGuildCommand(clientId, guildId, commandId), { body: updatedCommand } );
To delete a command, use the delete
method:
await rest.delete( Routes.applicationGuildCommand(clientId, guildId, commandId) );
Replace clientId
, guildId
, commandId
, and updatedCommand
with the appropriate values.