Discord.js Introduction
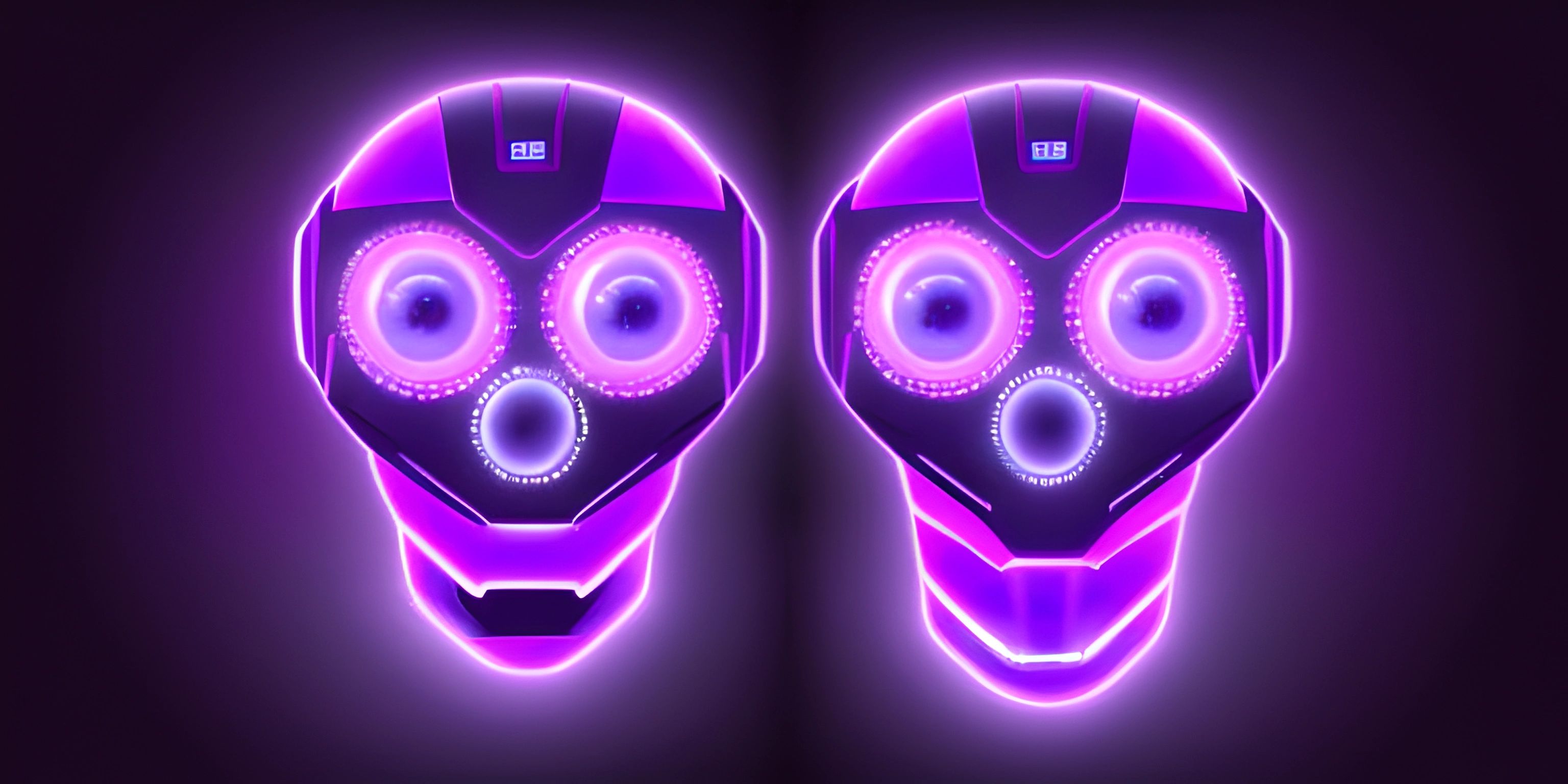
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've ever wanted to build your own Discord bot, you're in luck! The Discord.js library makes it super easy to create powerful and feature-rich bots. So grab your keyboard, and let's dive into the world of Discord bots.
What is Discord.js?
Discord.js is a powerful Node.js library that helps you interact with the Discord API. It simplifies tasks such as sending messages, managing channels, and handling user interactions. Basically, it provides you with all the tools you need to make your bot do awesome things.
Getting Started
To start using Discord.js, you'll first need to have Node.js installed on your machine. Once you have Node.js set up, you can create a new project and install Discord.js with the following command:
npm install discord.js
And that's it! You now have the power of Discord.js at your fingertips.
Creating Your First Discord Bot
Now, let's create a simple bot that greets users when they join a channel. First, you'll need to create a new file called index.js
and add the following code:
const Discord = require("discord.js"); const client = new Discord.Client(); client.on("ready", () => { console.log(`Logged in as ${client.user.tag}!`); }); client.on("message", (message) => { if (message.content === "!hello") { message.channel.send(`Hello, ${message.author}!`); } }); client.login("your-token-goes-here");
Make sure to replace "your-token-goes-here"
with your bot's token from the Discord Developer Portal. Once you've done that, start your bot by running:
node index.js
Now, your bot will reply with a greeting whenever someone sends a message with the content !hello
. Congratulations! You've just created your first Discord bot using Discord.js.
Learning More
There's so much more you can do with Discord.js, from managing roles to creating custom commands. To learn more about the possibilities, check out the official Discord.js documentation and explore various tutorials and examples available online.
Remember, creating a Discord bot is an ongoing process, and there's always room for improvement. So keep experimenting, learn from the community, and have fun building your own discord bot!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What is Discord.js and why should I use it for my bot?
Discord.js is a powerful and flexible JavaScript library that makes it easy to interact with the Discord API, allowing you to create and manage your own Discord bots. By using Discord.js, you can create bots with robust features, customize them according to your needs, and easily maintain and update them, all while leveraging the power of JavaScript.
How do I install Discord.js to start building my bot?
To install Discord.js, you'll first need to have Node.js installed on your system. Once you have Node.js set up, you can install Discord.js by running the following command in your terminal:
npm install discord.js
After the installation is complete, you can start building your bot by creating a JavaScript file and importing the Discord.js library with the require
function:
const Discord = require("discord.js");
How can I create a basic Discord bot using Discord.js?
Here's a simple example of how to create a basic Discord bot using Discord.js:
- Make sure you have Discord.js installed, as mentioned in the previous answer.
- Create a new JavaScript file (e.g.,
bot.js
) and add the following code:const Discord = require("discord.js"); const client = new Discord.Client(); client.on("ready", () => { console.log(`Logged in as ${client.user.tag}!`); }); client.on("message", (message) => { if (message.content === "ping") { message.reply("pong"); } }); client.login("your-bot-token");
- Replace
your-bot-token
with your bot's actual token, which you can obtain by creating a bot on the Discord Developer Portal. - Run your bot by executing the following command in your terminal:
node bot.js
Your bot should now be running and respond with "pong" whenever someone types "ping" in a text channel.
Can I add custom commands to my Discord.js bot?
Yes, you can add a variety of custom commands to your bot using Discord.js. To do so, you can use conditional statements within the message
event listener to check for specific commands and execute the corresponding actions. Here's an example of adding a !hello
command to your bot:
client.on("message", (message) => { if (message.content === "!hello") { message.reply("Hello there!"); } });
How can I troubleshoot common errors in my Discord.js bot?
When facing issues with your Discord.js bot, consider the following troubleshooting tips:
- Check your bot token: Ensure that you have entered the correct bot token in the
client.login()
function. - Verify your bot's permissions: Make sure your bot has the necessary permissions to read and send messages in the channels where you want it to interact.
- Examine your code: Look for syntax errors, missing variables, or incorrect function calls in your code.
- Consult the documentation: The Discord.js documentation is a valuable resource for learning more about the library and finding solutions to common issues.