Discord.js Tutorials
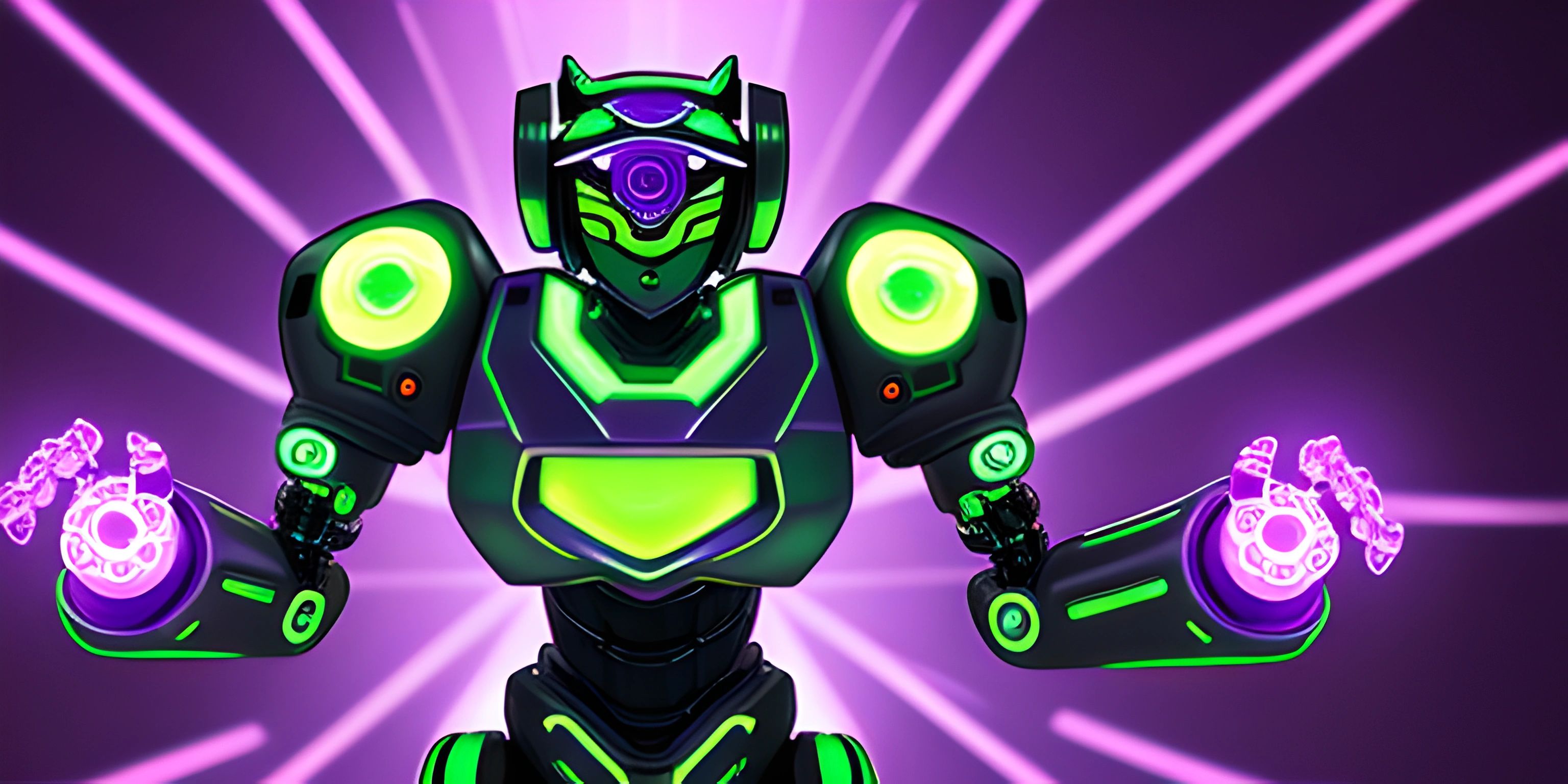
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Creating a Discord bot can be both fun and rewarding, and with Discord.js, it's easier than ever! In this series of guides, you will learn how to create, customize, and expand your very own Discord bot using the powerful Discord.js library.
Getting Started
Before diving into the exciting world of Discord bots, you'll need to set up your development environment. Here's what you'll need:
- Node.js: The runtime that will power your bot.
- npm: The package manager to install Discord.js and other dependencies.
- A code editor: We recommend Visual Studio Code.
Once you have these installed, you're ready to get started!
Setting up a Discord Application
Before you can say "Hello, world!" to your bot, you'll need to set up a new Discord application. Follow these steps:
- Visit the Discord Developer Portal.
- Click "New Application" and give it a name.
- Navigate to the "Bot" tab and click "Add Bot".
- Copy the bot token displayed under the "Build-A-Bot" section. Keep this safe, as this is the key to controlling your bot!
Creating Your Project
In your terminal, navigate to the folder where you want to create your bot project and run the following commands:
mkdir my-discord-bot cd my-discord-bot npm init -y
This will set up a basic Node.js project in the my-discord-bot
folder. Next, install the Discord.js library by running:
npm install discord.js
Now, create a bot.js
file in your project folder, and paste the following code:
const Discord = require("discord.js"); const client = new Discord.Client(); client.on("ready", () => { console.log(`Logged in as ${client.user.tag}!`); }); client.on("message", (msg) => { if (msg.content === "!ping") { msg.reply("Pong!"); } }); client.login("YOUR_BOT_TOKEN");
Replace "YOUR_BOT_TOKEN" with the token you copied earlier.
Running Your Bot
In your terminal, run:
node bot.js
Your bot should now be online and respond with "Pong!" whenever you send a message "!ping" in a server it's connected to.
Inviting Your Bot to a Server
To invite your bot to a server, follow these steps:
- Go back to the Discord Developer Portal and select your application.
- Navigate to the "OAuth2" tab.
- Check the "bot" option under "Scopes".
- Select the permissions your bot needs under "Bot Permissions".
- Copy the generated URL and visit it in your browser to invite your bot to a server.
Expanding Your Bot's Abilities
Now that you have a basic bot up and running, it's time to explore the various features Discord.js provides. Check out these guides to get started:
- Embeds: Enhance your bot's messages with rich embeds.
- Reactions: Make your bot interact with users by adding and responding to reactions.
- Command Handling: Organize your bot's commands for better maintainability and scalability.
- Events: Learn how to respond to various events in Discord using Discord.js.
Happy bot building!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making a Basic Discord Bot in JavaScript (discord.js) (psst, it's free!).
FAQ
What is Discord.js and why should I use it to build a Discord bot?
Discord.js is a powerful Node.js module that allows you to easily interact with the Discord API to create and control bots. By using Discord.js, you can build feature-rich, custom Discord bots that can do things like manage servers, send messages, and even play music. It's a popular choice for bot developers due to its ease of use and extensive documentation.
Can you provide a simple example of how to set up a Discord bot using Discord.js?
Sure! Here's a basic example to get you started. You'll need to have Node.js and Discord.js installed.
const Discord = require("discord.js"); const client = new Discord.Client(); client.on("ready", () => { console.log(`Logged in as ${client.user.tag}!`); }); client.on("message", (msg) => { if (msg.content === "ping") { msg.reply("pong"); } }); client.login("your-token-goes-here");
Replace "your-token-goes-here" with your bot's token, and your bot will respond with "pong" whenever someone sends a message with the word "ping".
How can I add more commands to my Discord bot using Discord.js?
To add more commands, you can simply extend the client.on("message", (msg) => {})
event listener, using conditionals (like if statements) to check for specific command keywords. Here's an example:
client.on("message", (msg) => { if (msg.content === "!hello") { msg.reply("Hello there!"); } else if (msg.content === "!help") { msg.reply("Here's a list of available commands: !hello, !help"); } });
In this example, the bot responds with "Hello there!" when a user sends a message with the command "!hello", and provides a list of available commands when a user sends a message with the command "!help".
How can I create more advanced commands, such as those with arguments?
You can create more advanced commands by parsing the message content and splitting it into separate parts (e.g., command name and arguments). Here's an example:
client.on("message", (msg) => { const args = msg.content.split(" "); const command = args.shift().toLowerCase(); if (command === "!say") { const textToSay = args.join(" "); msg.channel.send(textToSay); } });
In this example, the bot will respond by sending a message with the text provided after the "!say" command.
Can I use Discord.js to manage my server, such as creating channels and assigning roles?
Yes, you can! Discord.js provides many powerful features for server management. For example, to create a new text channel, you can use the following code:
client.on("message", async (msg) => { if (msg.content === "!createchannel") { try { const newChannel = await msg.guild.channels.create("new-channel", { type: "text", }); msg.reply(`Successfully created a new text channel: ${newChannel}`); } catch (error) { console.error("Error creating channel:", error); msg.reply("There was an error while creating the channel."); } } });
Similarly, you can manage roles, permissions, and more using the extensive functionality provided by the Discord.js library.