Getting Started with Electron
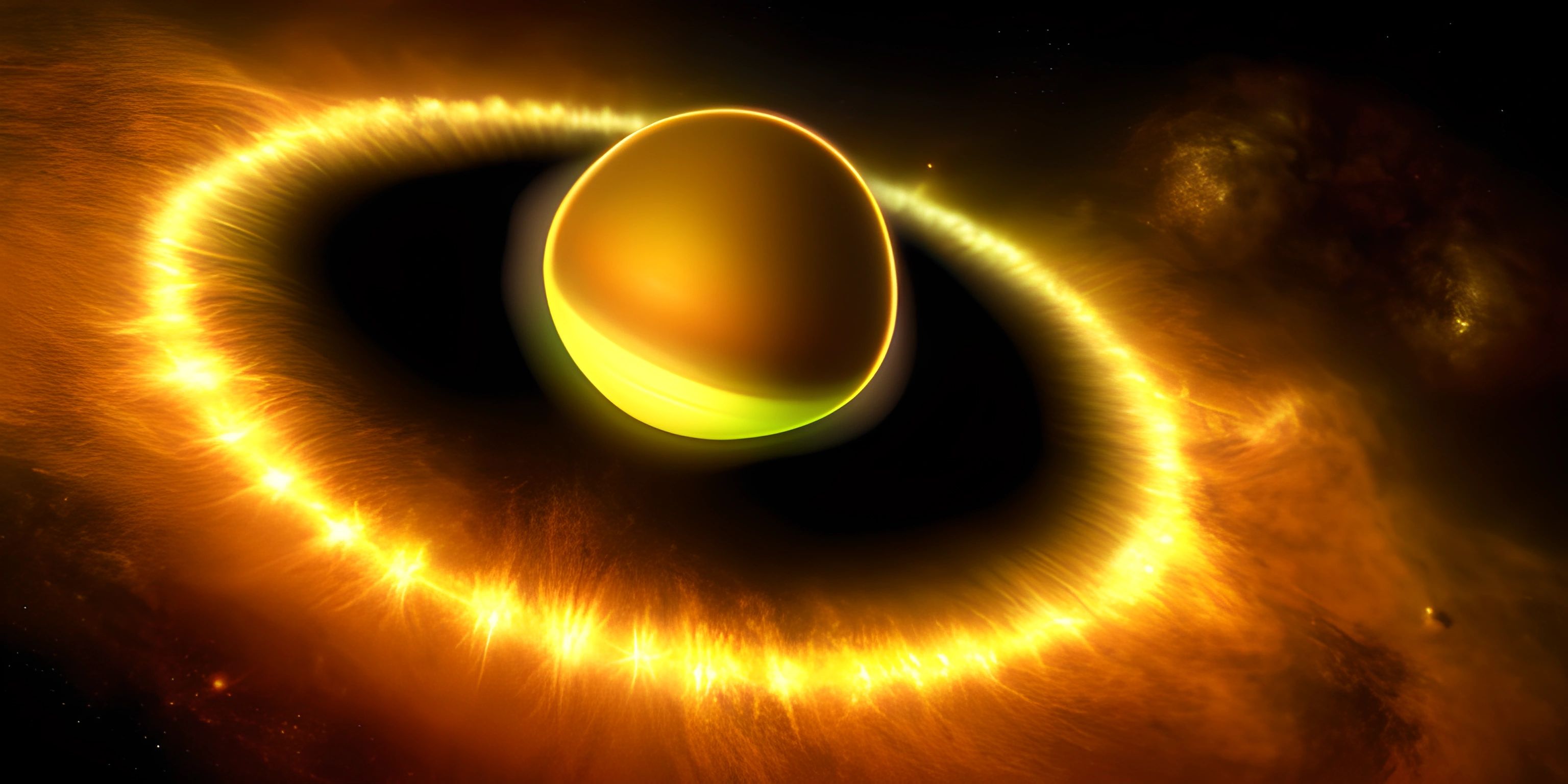
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Electron is a powerful framework for building cross-platform desktop applications using web technologies like JavaScript, HTML, and CSS. With Electron, you can create sleek and modern applications that run seamlessly on Windows, macOS, and Linux.
What is Electron?
Electron is an open-source framework that combines Node.js and Chromium to enable developers to create native desktop applications using web technologies. The magic of Electron lies in its ability to take advantage of Node.js for back-end functionality and Chromium for rendering a user interface.
Setting Up an Electron Project
To start building an Electron application, you'll need to have Node.js and npm (Node Package Manager) installed on your machine. If you don't have them installed already, follow the instructions on the official Node.js website.
With Node.js and npm in place, it's time to create a new project directory and navigate to it:
mkdir my-electron-app cd my-electron-app
Next, create a package.json
file to manage your project's dependencies:
npm init -y
Now, you can install Electron as a development dependency:
npm install electron --save-dev
Creating Your First Electron Application
With Electron installed, let's create an index.html
file in your project directory. This file will serve as the main user interface of your application:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>My Electron App</title> </head> <body> <h1>Hello Electron!</h1> </body> </html>
Now, create a main.js
file in your project directory. This file will serve as the entry point for your Electron application:
const { app, BrowserWindow } = require("electron"); function createWindow() { const win = new BrowserWindow({ width: 800, height: 600, webPreferences: { nodeIntegration: true } }); win.loadFile("index.html"); } app.whenReady().then(createWindow); app.on("window-all-closed", () => { if (process.platform !== "darwin") { app.quit(); } }); app.on("activate", () => { if (BrowserWindow.getAllWindows().length === 0) { createWindow(); } });
Finally, update the package.json
file to include a start
script that will launch your Electron application:
{ "name": "my-electron-app", "version": "1.0.0", "description": "", "main": "main.js", "scripts": { "start": "electron .", "test": "echo \"Error: no test specified\" && exit 1" }, "keywords": [], "author": "", "license": "ISC", "devDependencies": { "electron": "^15.3.3" } }
With everything in place, run your Electron application with the following command:
npm start
Congratulations! You've just created your first Electron application. From here, you can start exploring the extensive capabilities of Electron and create sophisticated desktop applications using your favorite web technologies. Don't forget to check out the official Electron documentation for more information and guidance. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is Electron and why should I use it for my desktop applications?
Electron is an open-source framework that allows developers to create cross-platform desktop applications using web technologies like JavaScript, HTML, and CSS. By using Electron, you can leverage your existing web development skills to build powerful and scalable applications that work seamlessly on Windows, macOS, and Linux.
How do I set up an Electron project?
To set up an Electron project, follow these steps:
- Ensure you have Node.js and npm installed on your system.
- Create a new directory for your project and navigate to it in your terminal.
- Run
npm init
to create apackage.json
file, and provide the required information. - Install Electron by running
npm install electron --save-dev
. - Create your main script file (e.g.,
main.js
) and the HTML file for your application's user interface. - In
package.json
, set themain
property to your main script file and add an npm start script. - Run
npm start
to launch your Electron application.
Can I use popular web frameworks like React or Angular with Electron?
Absolutely! Electron is compatible with popular web frameworks like React, Angular, and Vue.js. You can integrate these frameworks into your Electron project by following the standard setup process for each framework and then using the appropriate build process to bundle your code for use in your Electron application.
How do I package and distribute my Electron application?
To package and distribute your Electron application, you can use tools like electron-builder
or electron-packager
. These tools help you generate platform-specific executables and installers for your application, making it easy to distribute to your users. To get started, choose a packaging tool and follow the documentation to configure it for your project.
How can I access native system features in my Electron app?
Electron provides APIs that allow you to access native system features like file system operations, notifications, and more. These APIs can be accessed from both the main process (in your main script file) and the renderer process (in your application's user interface). To use these APIs, simply import the required module from the electron
package and start using the available methods and events.