Elixir Phoenix Web Application
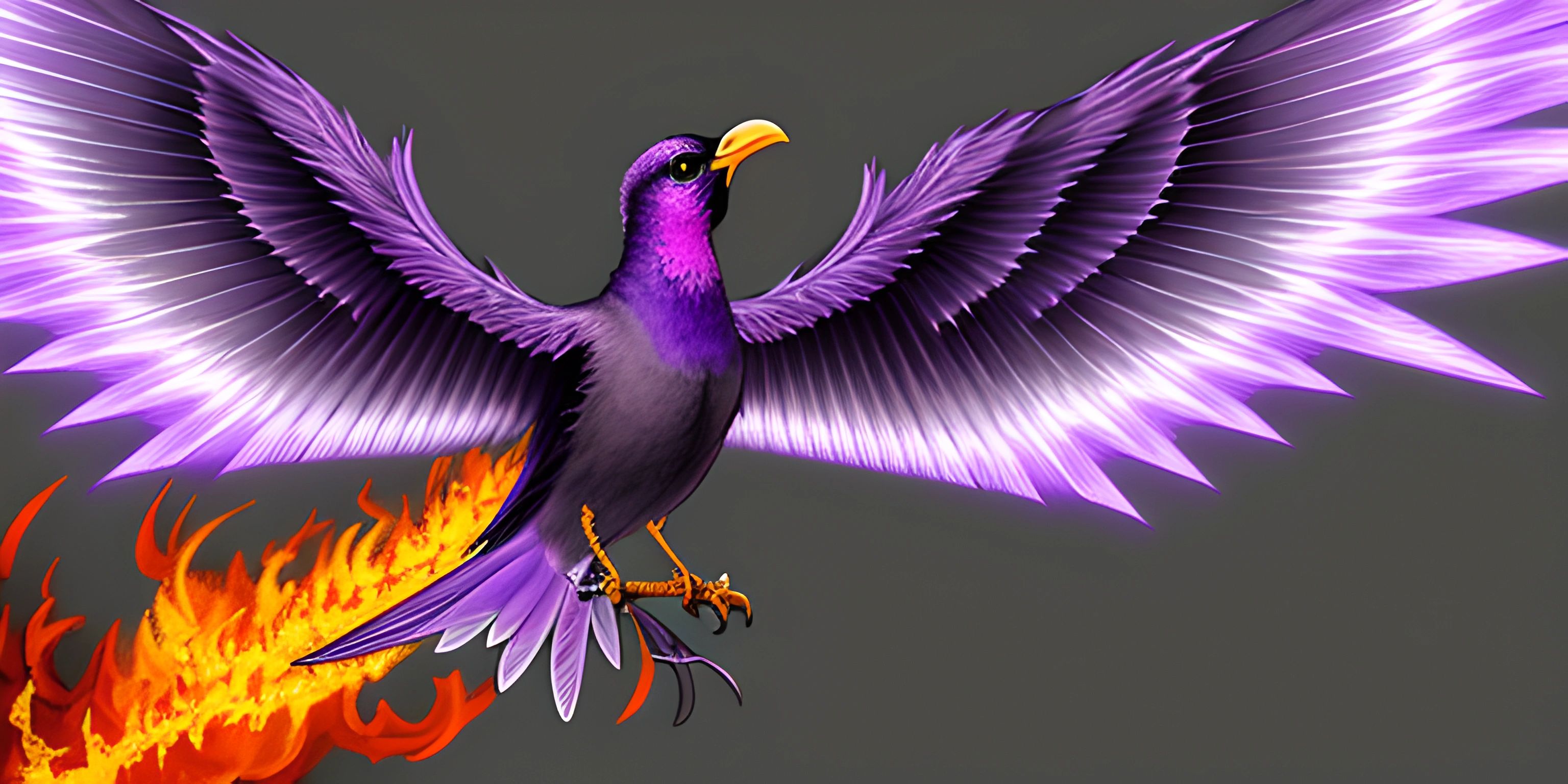
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Elixir, a functional programming language built on the Erlang VM, is known for its scalability and fault tolerance. When combined with the Phoenix Framework, a web development platform, you can create powerful and maintainable web applications. Let's dive into the magical world of Elixir and Phoenix to build a simple web app!
Setting Up Elixir and Phoenix
Before we begin, you need to have Elixir installed on your system. Head over to the official Elixir installation guide and follow the instructions for your operating system.
Next, we'll install the Phoenix Framework. Open a terminal and run the following command:
mix archive.install hex phx_new
This command installs the phx_new
Mix archive, which lets you create new Phoenix projects.
Creating a Phoenix Project
To create a new Phoenix project, run:
mix phx.new my_app
Replace my_app
with the name you want for your application. This command will generate a new Phoenix project in a directory with the same name as your app. Navigate into the newly created directory:
cd my_app
Starting the Phoenix Server
Now that we've created our project, let's start the Phoenix server. From the root directory of your project, run:
mix phx.server
This command starts the server, and you can now view your app by going to http://localhost:4000
in your browser. You should see the default Phoenix welcome page. Congratulations, you have a running Phoenix app!
Creating a Simple Page
Let's create a new page for our app. In Phoenix, a page is represented by a combination of a controller, a view, and a template. We'll start by generating a new controller:
mix phx.gen.html Pages Page pages message:string
This command generates a new Page
module with a message
attribute of type string
. It also creates the necessary controller, view, and template files. Now, we need to add a new route for our page in lib/my_app_web/router.ex
:
scope "/", MyAppWeb do pipe_through :browser get "/", PageController, :index resources "/pages", PageController, only: [:index, :new, :create, :show] end
This code adds a route for the root path ("/") that maps to the index
action of our PageController
. It also adds routes for creating and displaying pages.
Now, run the following command to apply the changes to your database:
mix ecto.migrate
Updating the Template
Finally, let's customize the template for our new page. Open the file lib/my_app_web/templates/page/index.html.eex
and replace its contents with:
<h1>Hello, Phoenix!</h1> <p>My message: <%= @page.message %></p>
This code displays a heading and the message attribute of our Page
module.
Viewing the Results
Start the Phoenix server again with mix phx.server
, and visit http://localhost:4000
in your browser. You should now see your custom page with the "Hello, Phoenix!" message.
Wrapping Up
You've just built a simple web application using Elixir and the Phoenix Framework! This is just the tip of the iceberg, as Phoenix offers many more features and functionalities to help you build powerful, scalable applications. From here, you can explore the official Phoenix documentation to learn more about what you can do with this fantastic framework. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is the Elixir Phoenix Framework?
The Elixir Phoenix Framework is a highly efficient and productive web framework built on top of the Elixir programming language. It's designed for building high-performance, scalable, and maintainable web applications. Phoenix takes advantage of Elixir's lightweight concurrency and fault-tolerance capabilities, making it ideal for creating modern web applications.
How do I start a new Phoenix web application project?
To start a new Phoenix web application project, follow these steps:
- Install Elixir and the Hex package manager on your system.
- Install the Phoenix archive by running the following command in your terminal:
mix archive.install hex phx_new
- Create a new Phoenix project by running:
mix phx.new project_name
- Change to the project's directory by running
cd project_name
. - Install the project's dependencies by running
mix deps.get
. - Start the Phoenix development server by running
mix phx.server
.
How do I create a new page in a Phoenix web application?
To create a new page in a Phoenix web application, follow these steps:
- Create a new controller module in the
lib/project_name_web/controllers
directory. - Define a new action in the controller that renders the new page.
- Create a new template file in the
lib/project_name_web/templates/controller_name
directory. - Update the
router.ex
file in thelib/project_name_web
directory to define a new route that maps to the newly created action in the controller. - Restart the Phoenix server to see your new page at the specified route.
How do I interact with a database in a Phoenix web application?
In a Phoenix web application, you interact with a database using Ecto, which is a powerful and flexible database wrapper and query generator. To interact with a database, follow these steps:
- Configure your application's database settings in the
config/config.exs
file. - Create a new schema file in the
lib/project_name
directory that defines the structure of the data you want to store in the database. - Create a new migration file in the
priv/repo/migrations
directory that defines the changes to apply to the database structure. - Run the migration using the command
mix ecto.migrate
. - In your controller or context module, use the Ecto functions to query, insert, update, or delete data in the database.
How do I deploy a Phoenix web application to production?
To deploy a Phoenix web application to production, you can use various deployment options like Gigalixir, Heroku, or even deploy it on your own server using tools like Distillery or Docker. In general, the deployment process involves:
- Configuring your application for production, including setting up environment variables, database settings, and any other required configurations.
- Compiling your application for production using
mix release
or a similar tool. - Deploying the compiled application to your chosen platform or server.
- Starting your application and monitoring its performance and logs.