Building Web Applications with Rails
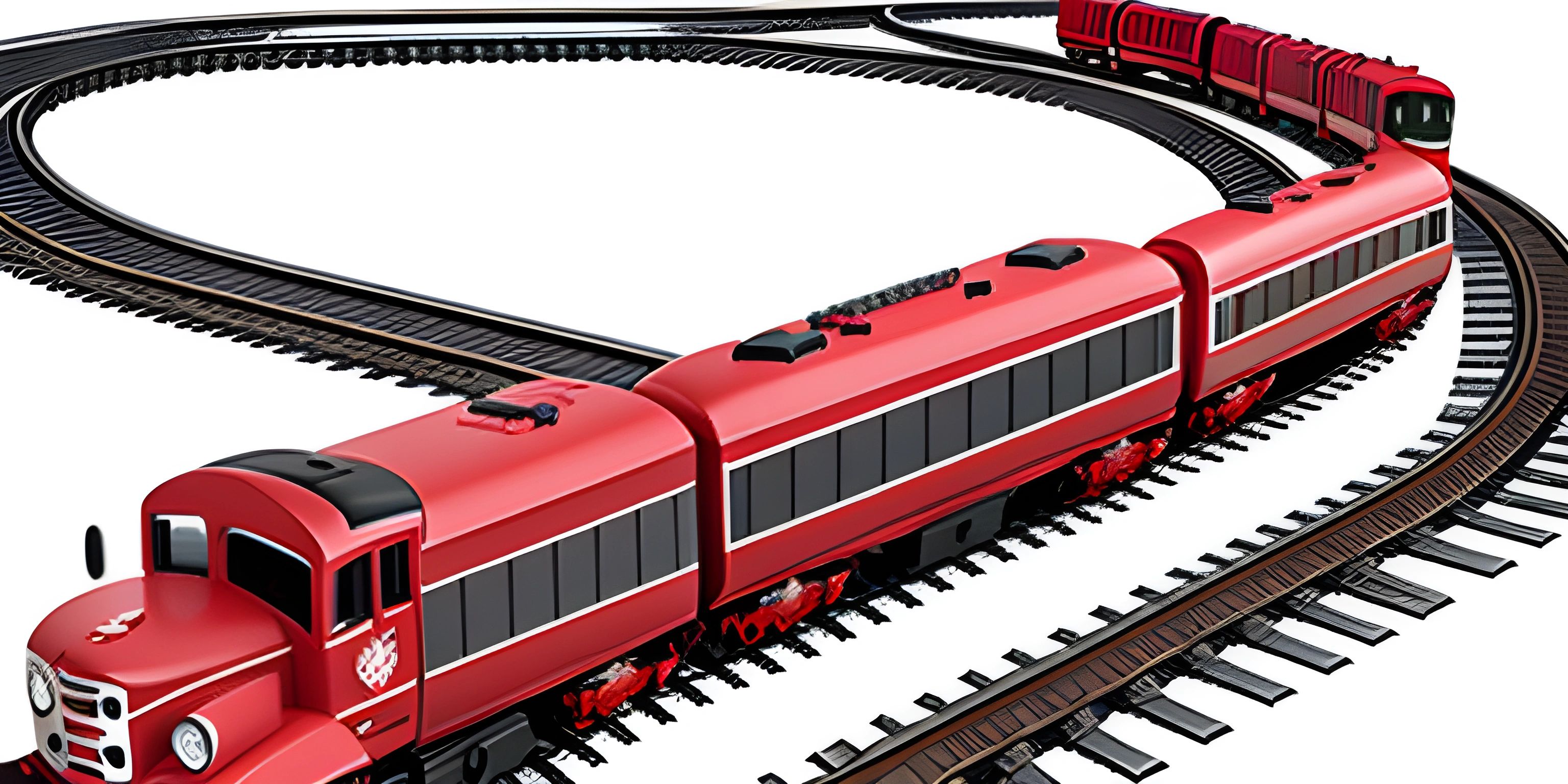
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Web development can be a complex and time-consuming process. Thankfully, there are many frameworks out there that can help make our lives easier. One such framework is Ruby on Rails, often called just "Rails". Rails is a powerful web application framework that provides developers with the tools they need to quickly build and deploy web applications. Without further ado, let's dive into building web applications with Rails!
Installing Ruby on Rails
Before we can start building our Rails application, we need to install the Rails framework. To do that, you first need to have Ruby installed on your system. Rails is a Ruby gem, which means we can easily install it using the following command:
gem install rails
Now that Rails is installed, we can create our first Rails application.
Creating a Rails Application
To create our Rails application, we'll use the rails new
command. In your terminal, navigate to the directory where you want to create your application and run:
rails new my_app
Replace my_app
with the desired name of your application. This will create a new folder with the same name and generate the necessary files and folders for a basic Rails application.
Understanding the Rails File Structure
Let's take a look at the generated files and folders. Some of the most important ones include:
app/
: Contains the main components of your application, such as models, views, and controllers.config/
: Contains configuration files for your application.db/
: Contains your database-related files, such as migrations and your schema.public/
: Contains static files like images, stylesheets, and JavaScript files.Gemfile
: Specifies the gems that your application depends on.
Building a Simple Web Application
Now that we have an understanding of the basic file structure, let's create a simple web application. Rails follows the Model-View-Controller (MVC) architecture, so we'll need to create a model, view, and controller for our application.
Generating a Controller
First, let's generate a controller named Welcome
. In your terminal, run:
rails generate controller Welcome
This will create a new controller file (app/controllers/welcome_controller.rb
) and a new folder for the views (app/views/welcome/
).
Creating a View
Next, let's create a view for the Welcome
controller. Create a new file named index.html.erb
inside the app/views/welcome/
folder. In this file, add the following simple HTML code:
<!DOCTYPE html> <html> <head> <title>Welcome to My App</title> </head> <body> <h1>Hello, Rails!</h1> </body> </html>
Configuring the Route
To display our Welcome
view when a user visits our application, we need to configure a route in config/routes.rb
:
Rails.application.routes.draw do get 'welcome/index' root 'welcome#index' # set the root route to welcome#index end
Running the Rails Server
Now that our simple web application is set up, let's run the Rails server to see it in action. In your terminal, run:
rails server
This will start the Rails server on port 3000 by default. Open your favorite web browser and visit http://localhost:3000 to see your new Rails web application in action!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Ruby on Rails?
Ruby on Rails, often just called "Rails", is a powerful web application framework that allows developers to quickly build and deploy web applications using the Ruby programming language.
How do I install Rails?
To install Rails, first ensure that Ruby is installed on your system. Then, use the following command to install the Rails gem: gem install rails
.
What is the MVC architecture?
The Model-View-Controller (MVC) architecture is a design pattern for organizing code in a way that separates the concerns of data management, user interface, and control flow. Rails follows the MVC architecture.
How do I create a new Rails application?
To create a new Rails application, navigate to the directory where you want to create your application and run the rails new my_app
command, replacing "my_app" with the desired name of your application.
How do I run the Rails server?
To run the Rails server, open your terminal, navigate to your Rails application directory, and run the rails server
command. This will start the Rails server on port 3000 by default.