Introduction to Koa.js
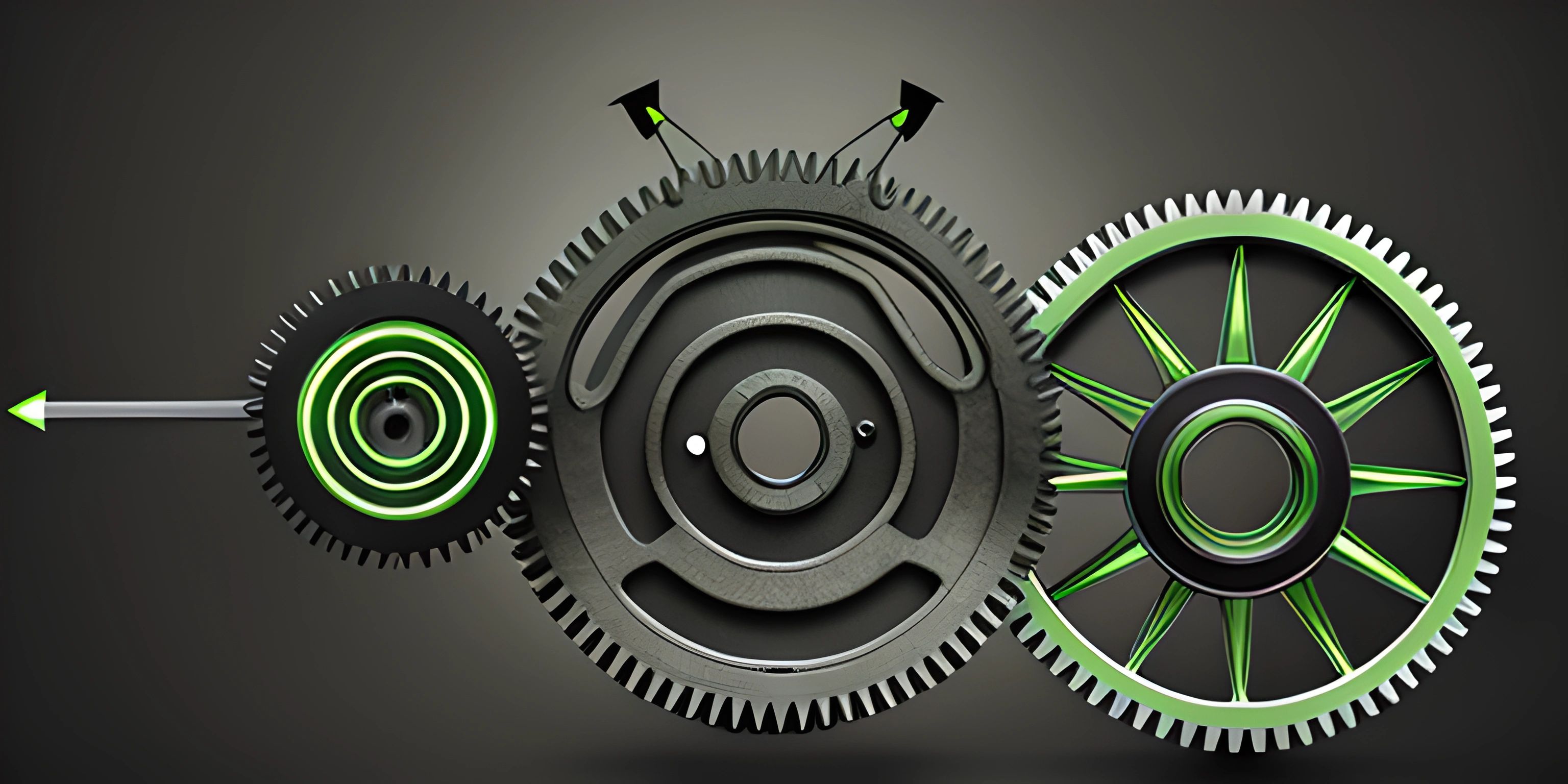
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Stepping into the world of JavaScript web frameworks, you'll quickly notice that Express.js has been reigning supreme. However, the creators of Express.js didn't just sit on their laurels; they went on to create Koa.js, a leaner and meaner framework built to modernize and simplify server-side JavaScript development.
What is Koa.js?
Koa.js, often just called Koa, is a lightweight web framework for Node.js applications. It's designed to be more expressive and robust, making it easier to write clean and maintainable code. Koa.js leverages modern JavaScript features like async/await, resulting in a more enjoyable development experience.
Middleware
At the heart of Koa lies the concept of middleware. Middleware are building blocks that process and modify requests and responses, providing a flexible way to compose your application. Koa's middleware is designed to work as a stack, where each middleware function can decide whether to pass control to the next middleware or directly respond to the request.
Here's a simple example:
const Koa = require("koa"); const app = new Koa(); app.use(async (ctx, next) => { console.log("Middleware 1"); await next(); console.log("Middleware 1 - After next"); }); app.use(async (ctx, next) => { console.log("Middleware 2"); await next(); }); app.listen(3000);
When a request comes in, the console will output:
Middleware 1 Middleware 2 Middleware 1 - After next
Comparison with Express.js
Koa.js, being the spiritual successor of Express.js, shares many similarities with its older sibling. However, there are some key differences that set the two apart:
- Async/await: Koa fully embraces async/await, making your code easier to read and write. This reduces the need for callbacks and helps avoid the dreaded "callback hell."
- Minimalism: Koa is intentionally more minimalistic than Express. This means it has fewer built-in features, allowing you to pick and choose the libraries you want to use, resulting in a leaner and more tailored solution.
- Error handling: Koa has better built-in error handling, which makes it easier to handle and report errors in a consistent manner.
Getting Started with Koa.js
To kickstart your Koa.js journey, you first need to install it via npm:
npm install koa
Next, create an app.js
file and add the following code to set up a basic Koa server:
const Koa = require("koa"); const app = new Koa(); app.use(async (ctx) => { ctx.body = "Hello, Koa!"; }); app.listen(3000, () => { console.log("Server running on http://localhost:3000"); });
Run your server with node app.js
, and visit http://localhost:3000
to see your first Koa.js app in action!
Why Choose Koa.js?
Koa.js is an excellent choice when you're looking for a modern, lightweight, and flexible web framework. Its focus on middleware composition and async/await support enables cleaner and more maintainable code. If you're familiar with Express.js, you'll feel right at home with Koa, while also benefiting from its improvements and modern approach.
Koa.js may not be as feature-rich as Express.js out of the box, but its minimalism allows you to craft a custom solution tailored to your project's needs. So, give Koa.js a try and see if it's the right fit for your next Node.js venture!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).