Introduction to Stacks
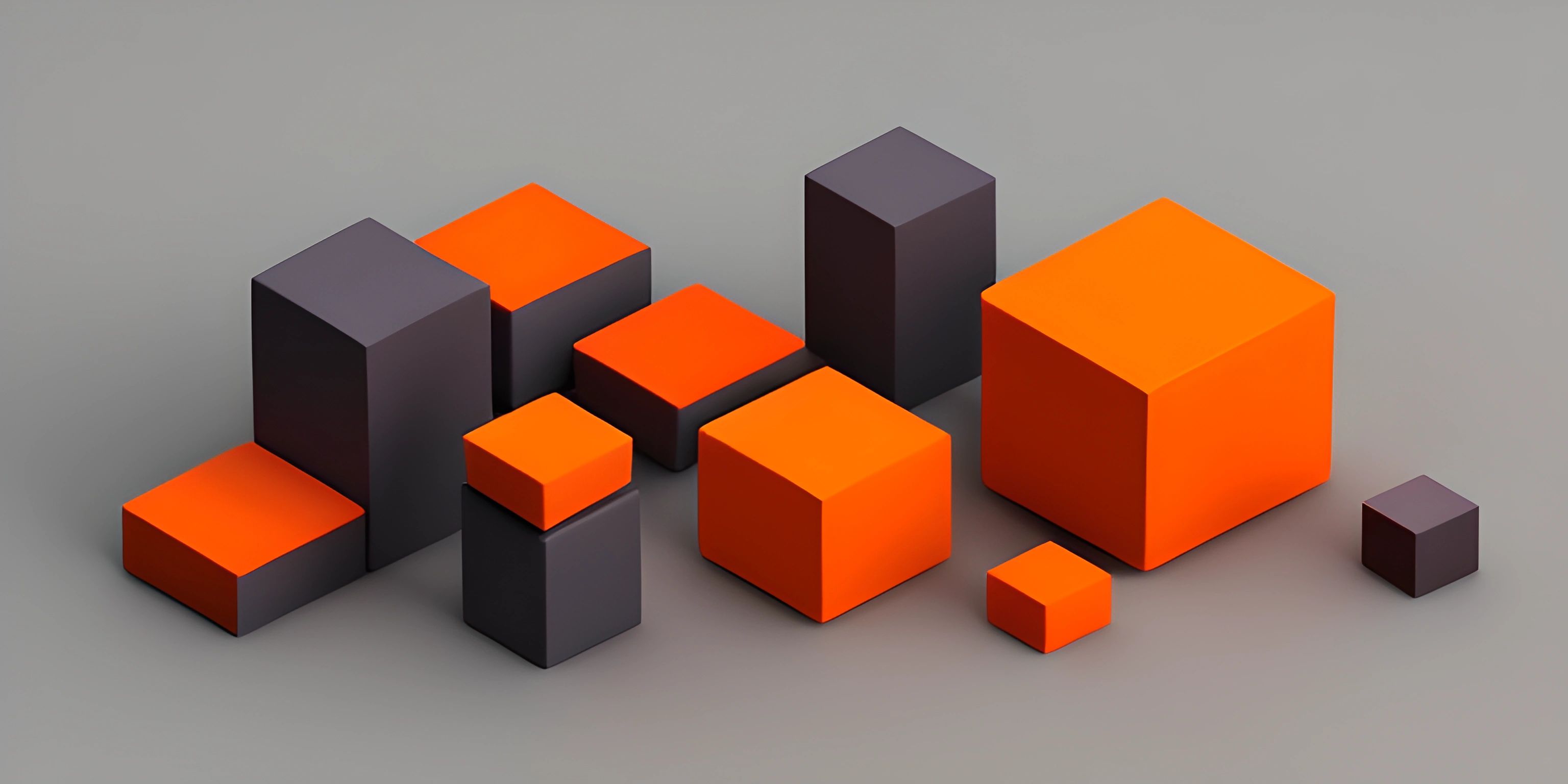
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
You might have heard people say, "Life is like a stack of pancakes." Well, that's not what we are going to talk about here, but it's a fun way to introduce you to stacks, a fundamental data structure in programming.
What is a Stack?
A stack is a data structure that follows the LIFO (Last In, First Out) principle. This means that the last element added to the stack is the first one to be removed. Think of it as a pile of pancakes: you can't eat the one at the bottom without first removing the ones on top!
In programming, stacks can be used to store and organize data in a way that's easy to access and manage.
Basic Operations
There are two primary operations you can perform on a stack:
- Push: This operation adds an element to the top of the stack.
- Pop: This operation removes and returns the top element from the stack.
Additionally, you can also peek to view the top element without removing it.
Here's an example in Python:
stack = [] # Push elements onto the stack stack.append("pancake1") stack.append("pancake2") stack.append("pancake3") # Pop the top element off the stack top_pancake = stack.pop() print(top_pancake) # Output: pancake3 # Peek at the new top element new_top_pancake = stack[-1] print(new_top_pancake) # Output: pancake2
Use Cases
Stacks are incredibly versatile and can be used in many programming scenarios. Some common use cases include:
- Undo/Redo functionality: By keeping track of actions in a stack, you can easily implement undo and redo operations in applications such as text editors or image editors.
- Balancing symbols: Stacks can be used to validate the balance of symbols in a given string, such as checking for matching parentheses or brackets in code.
- Function call management: Programming languages like C++ and Java utilize stacks to manage function calls, helping keep track of return addresses and local variables.
There are many more scenarios where stacks prove to be the perfect solution. As you dive deeper into the world of programming, you'll undoubtedly encounter stacks and appreciate their elegant simplicity. Now go forth and stack those pancakes, er, I mean, data elements!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Data Types (psst, it's free!).
FAQ
What is a stack in programming?
A stack is a fundamental data structure in programming that follows the Last-In-First-Out (LIFO) principle. It means that the most recently added element in the stack is the first one to be removed. Stacks are used in various scenarios, such as managing function calls, reversing strings, or evaluating expressions.
How do I perform basic operations on a stack?
Basic operations on a stack include push, pop, and peek. Here's a brief explanation of each operation:
- Push: Adds an element to the top of the stack.
- Pop: Removes the topmost element from the stack.
- Peek: Returns the topmost element without removing it from the stack.
Can you provide an example of using a stack in code?
Sure! Here's a simple example using Python:
class Stack: def __init__(self): self.items = [] def push(self, item): self.items.append(item) def pop(self): if not self.is_empty(): return self.items.pop() def peek(self): if not self.is_empty(): return self.items[-1] def is_empty(self): return len(self.items) == 0 # Initialize a stack object my_stack = Stack() # Push elements onto the stack my_stack.push(1) my_stack.push(2) my_stack.push(3) # Pop the top element from the stack print(my_stack.pop()) # Output: 3 # Peek at the top element of the stack print(my_stack.peek()) # Output: 2
What are some common use cases for stacks in programming?
Stacks are often used in a variety of programming scenarios, such as:
- Managing function calls and their execution order.
- Reversing strings or sequences.
- Parsing and evaluating expressions, such as arithmetic or logical expressions.
- Implementing undo/redo functionality in applications.
What are the main differences between stacks and queues?
The primary difference between stacks and queues lies in their element removal order:
- Stacks follow the Last-In-First-Out (LIFO) principle, meaning the most recently added element is removed first.
- Queues follow the First-In-First-Out (FIFO) principle, meaning the oldest element in the queue is removed first.