Iterative vs Recursive: A Comparison
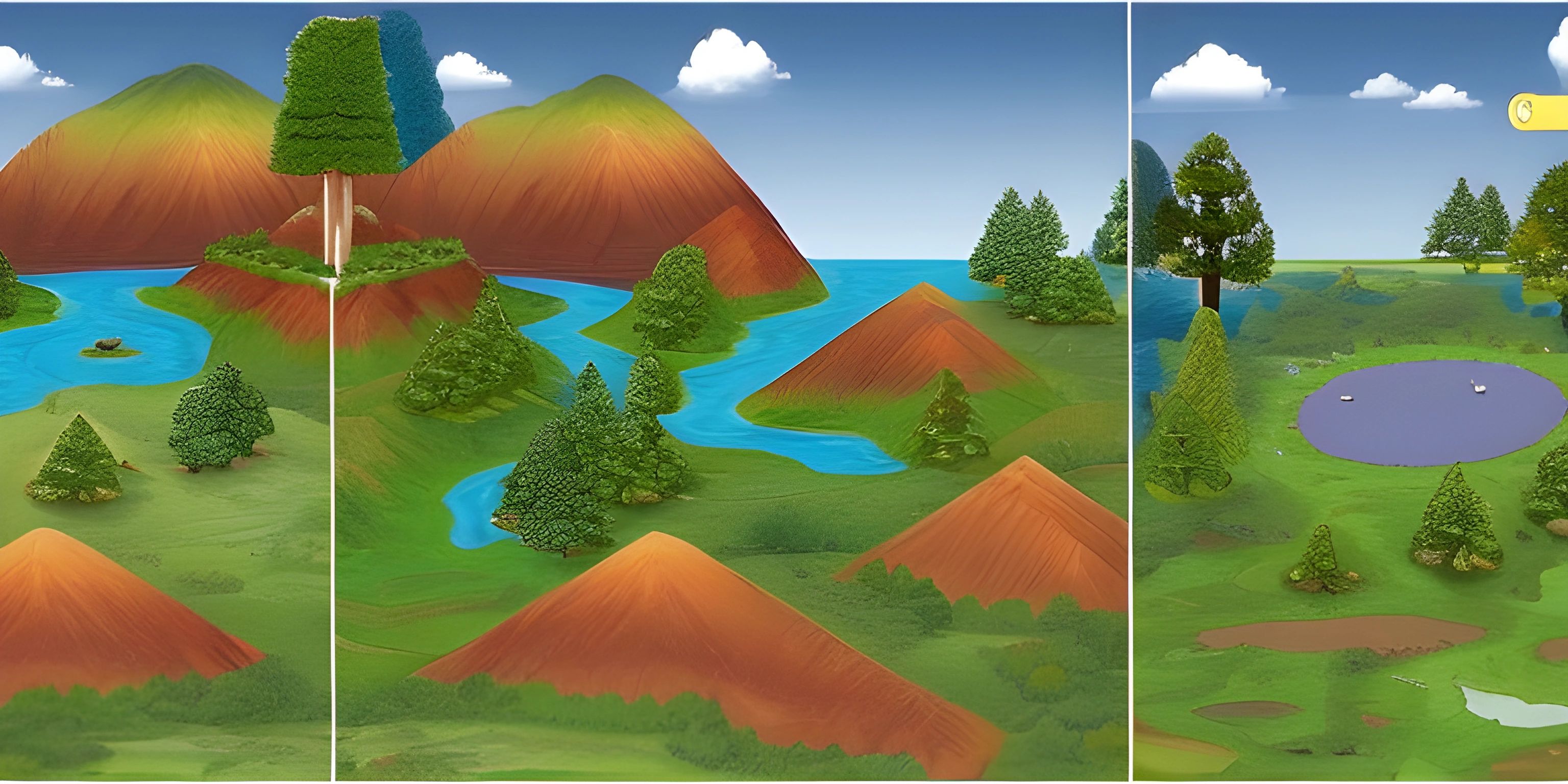
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Diving into the world of programming, we often come across two major techniques - Iterative and Recursive. They are like two sides of the same coin, each with its own merits and demerits. Let's get the ball rolling and dig into the nitty-gritty of these two popular approaches.
Iterative Approach
In an iterative approach, we use loops to solve a problem. It is like being stuck in a never-ending cycle, but with a specific goal in mind. Here's a simple example to illustrate the iterative approach using pseudocode:
for i from 1 to 5 print i
In this example, we're cycling through the range 1 to 5, printing each number on each iteration. It's as straightforward as going through a shopping list.
Recursive Approach
The recursive approach, on the other hand, is a bit like a Russian Doll. A recursive function is a function that calls itself until it reaches a base case. Let's look at the same example as above but solved recursively:
function print_numbers(n) if n > 0 print_numbers(n - 1) print n
If you call print_numbers(5)
, it will print 1 through 5. It may seem magical, but it's just the way recursion works. The function keeps calling itself with a smaller value until it reaches the base case (n > 0).
Comparison
Now that we've got a basic understanding of both iterative and recursive approaches, let's compare them side by side. The iterative solution is straightforward and easy to understand but can get cumbersome when the iteration becomes complex. Recursive solutions, on the other hand, can be elegant and concise but can also be harder to understand, and they can lead to stack overflow errors if the recursion goes too deep.
It's like choosing between a car and a bike. A car can carry a lot of stuff and is comfortable, but it's more expensive and can be difficult to park in crowded places. A bike, on the other hand, is more economical, easier to maneuver but can't carry as much stuff, and you wouldn't want to use it for long-distance travel.
In the end, the choice between iterative and recursive approaches depends on your specific needs and the problem you're trying to solve.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is the iterative approach in programming?
The iterative approach in programming involves using loops to solve a problem. It is like going through a cycle repeatedly until a specific goal is reached. This approach is straightforward and easy to understand, but it can get cumbersome when the iteration becomes complex.
What is the recursive approach in programming?
The recursive approach in programming involves a function calling itself until it reaches a base case. It is like a Russian doll, where one function contains a smaller version of itself. This approach can be elegant and concise but can also be harder to understand, and it can lead to stack overflow errors if the recursion goes too deep.
When should I use iterative or recursive approach?
The choice between iterative and recursive approaches depends on your specific needs and the problem you're trying to solve. If the problem is straightforward and doesn't involve complex iterations, an iterative approach might be more suitable. On the other hand, if the problem involves complex structures like trees or graphs, a recursive approach might be more appropriate. It's like choosing the right tool for the job.