Introduction to TypeScript
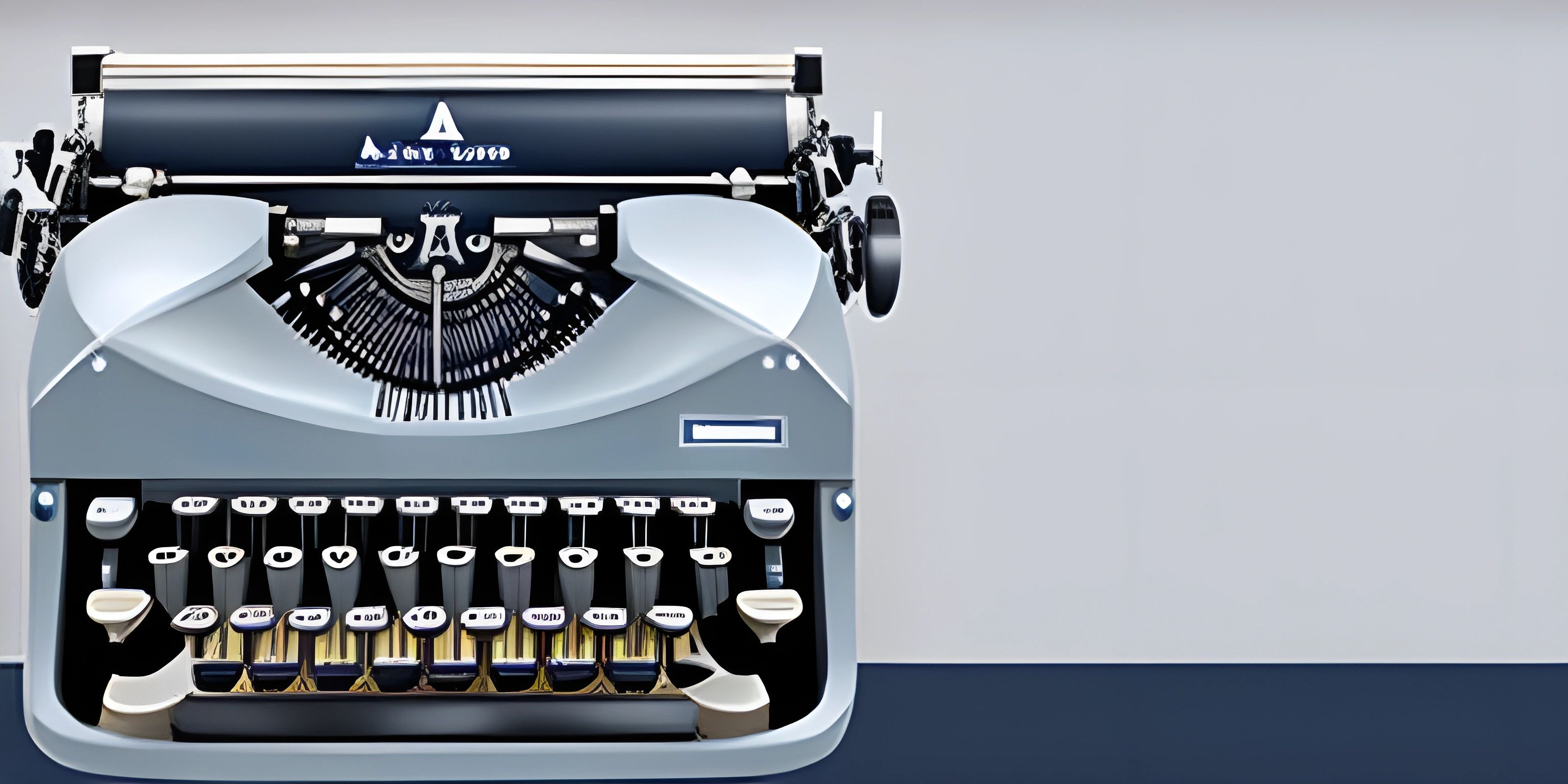
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
TypeScript is a powerful and popular language that has been taking the web development world by storm. This statically-typed superset of JavaScript adds a layer of structure and safety to your code that JavaScript simply can't provide on its own. If you've ever encountered a bug caused by working with the wrong type of value in JavaScript, TypeScript might be the answer to your woes.
What is TypeScript?
TypeScript is an open-source, object-oriented programming language developed and maintained by Microsoft. It was created to address some of the shortcomings of JavaScript, such as the lack of static typing and poor support for large-scale projects. By adding optional static typing and other features, TypeScript allows developers to catch errors early in the development process.
Since TypeScript is a superset of JavaScript, it means that any valid JavaScript code is also valid TypeScript code. This makes it easy to adopt TypeScript in existing projects, as you can gradually introduce TypeScript features into your codebase.
Features of TypeScript
Here are some of the core features that make TypeScript stand out from vanilla JavaScript:
Static Typing
TypeScript introduces static typing, which means that variables, function parameters, and return values can have explicit types. This helps catch errors early, as the TypeScript compiler will alert you if you try to assign a value of the wrong type to a variable or pass an incorrect argument to a function.
For example, if you define a variable as a string
and try to assign a number to it, the compiler will throw an error:
let message: string = "Hello, world!"; message = 42; // Error: Type 'number' is not assignable to type 'string'.
Interfaces
TypeScript allows you to define interfaces, which are a way to describe the shape of an object. This can help ensure that objects have the correct properties and that they conform to a specific structure.
For example, you can define an interface for a Person
object like this:
interface Person { firstName: string; lastName: string; age: number; }
Now you can use this interface to ensure that objects of type Person
have the correct properties:
let person: Person = { firstName: "John", lastName: "Doe", age: 30, };
Classes and Inheritance
TypeScript supports classes and inheritance, similar to other object-oriented programming languages like Java and C#. This enables a more structured approach to organizing your code and encourages reusability.
Here's a simple example of a class in TypeScript:
class Animal { name: string; constructor(name: string) { this.name = name; } makeSound(): void { console.log("The animal makes a sound"); } } class Dog extends Animal { makeSound(): void { console.log("The dog barks"); } } const myDog = new Dog("Buddy"); myDog.makeSound(); // Output: The dog barks
Advanced Types
TypeScript also introduces advanced types, such as union types, intersection types, and mapped types. These types allow you to express more complex relationships between your data structures and can help catch errors that would be hard to find in plain JavaScript.
Conclusion
TypeScript offers a powerful set of features on top of JavaScript, making it a popular choice for many developers. With its static typing, interfaces, classes, and advanced types, TypeScript provides a more robust and structured approach to web development, making it an excellent choice for large-scale projects or those looking for improved type safety.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is TypeScript?
TypeScript is an open-source, object-oriented programming language developed and maintained by Microsoft. It is a superset of JavaScript, meaning that any valid JavaScript code is also valid TypeScript code. TypeScript introduces static typing, interfaces, classes, and advanced types, providing a more robust and structured approach to web development.
What are some features of TypeScript?
Some core features of TypeScript include static typing, interfaces, classes, inheritance, and advanced types, such as union types, intersection types, and mapped types. These features provide a more robust and structured approach to web development, making TypeScript an excellent choice for large-scale projects or those looking for improved type safety.
How does TypeScript relate to JavaScript?
TypeScript is a superset of JavaScript, meaning that any valid JavaScript code is also valid TypeScript code. TypeScript builds on top of JavaScript, adding optional static typing and other features to address some of the shortcomings of JavaScript, such as the lack of static typing and poor support for large-scale projects.