React Introduction
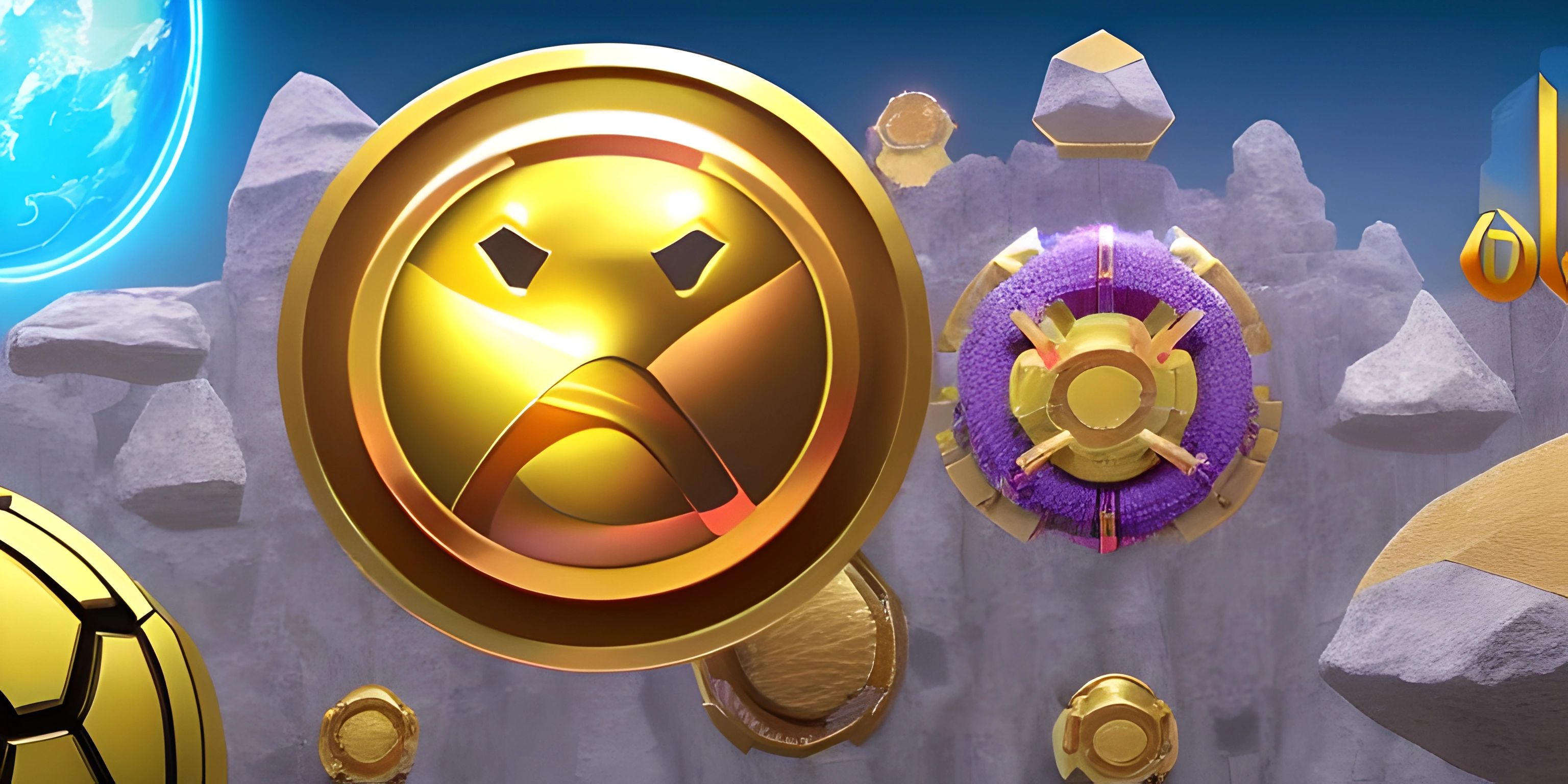
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
React, a popular JavaScript library, has taken the world of web development by storm. Created by Facebook, React is designed to simplify the process of building user interfaces, allowing developers to focus on what matters most: creating a seamless and enjoyable experience for users.
What is React?
React is a library for building user interfaces (UIs) through the use of reusable components. These components are the building blocks of a React application, encapsulating both the visual elements and the underlying logic.
In a traditional web development approach, UI elements are often tightly coupled with the JavaScript code that manipulates them. This can make it difficult to manage, maintain, and scale applications as they grow. React addresses this problem by promoting a more modular and maintainable structure.
How Does React Work?
React works by utilizing a virtual representation of the DOM, known as the Virtual DOM. The Virtual DOM is an in-memory representation of the actual web page, allowing React to quickly update the UI without touching the real DOM.
Whenever there's a change in the application's state, React updates the Virtual DOM, calculates the difference between the new and old Virtual DOM (called "diffing"), and then efficiently updates the real DOM with only the necessary changes. This process, known as reconciliation, allows React to deliver optimal performance.
Components: The Building Blocks of a React App
React components are essentially JavaScript functions or classes that return a piece of JSX (JavaScript XML), which is a syntax extension that allows you to write HTML-like code within your JavaScript code. Components can be composed of other components, promoting reusability and maintainability.
There are two main types of components in React: class components and functional components. Class components are built using ES6 classes and provide access to more advanced features like state and lifecycle methods, while functional components are simpler and typically used for presentational purposes.
Here's an example of a simple functional component:
import React from "react"; function Greeting() { return ( <div> <h1>Welcome to React!</h1> <p>Let's build some amazing interfaces.</p> </div> ); } export default Greeting;
State and Props
React components have two primary ways of managing data: state and props. State represents the internal data of a component, while props are external data passed down from a parent component.
State can be managed within class components using this.state
and this.setState()
, and within functional components using the useState
hook.
Props, on the other hand, are immutable and are passed down from parent to child components as arguments. This promotes a unidirectional data flow, making it easier to reason about the application's data flow and state management.
Final Thoughts
React has revolutionized the way we build web interfaces by providing a modular, maintainable, and performance-driven structure. With its focus on components, state management, and an efficient reconciliation process, React empowers developers to create powerful and scalable applications.
Now that you're familiar with the basics, it's time to explore more advanced topics like React hooks, React lifecycle methods, and Redux to enhance your React development skills.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).