Introduction to Kotlin
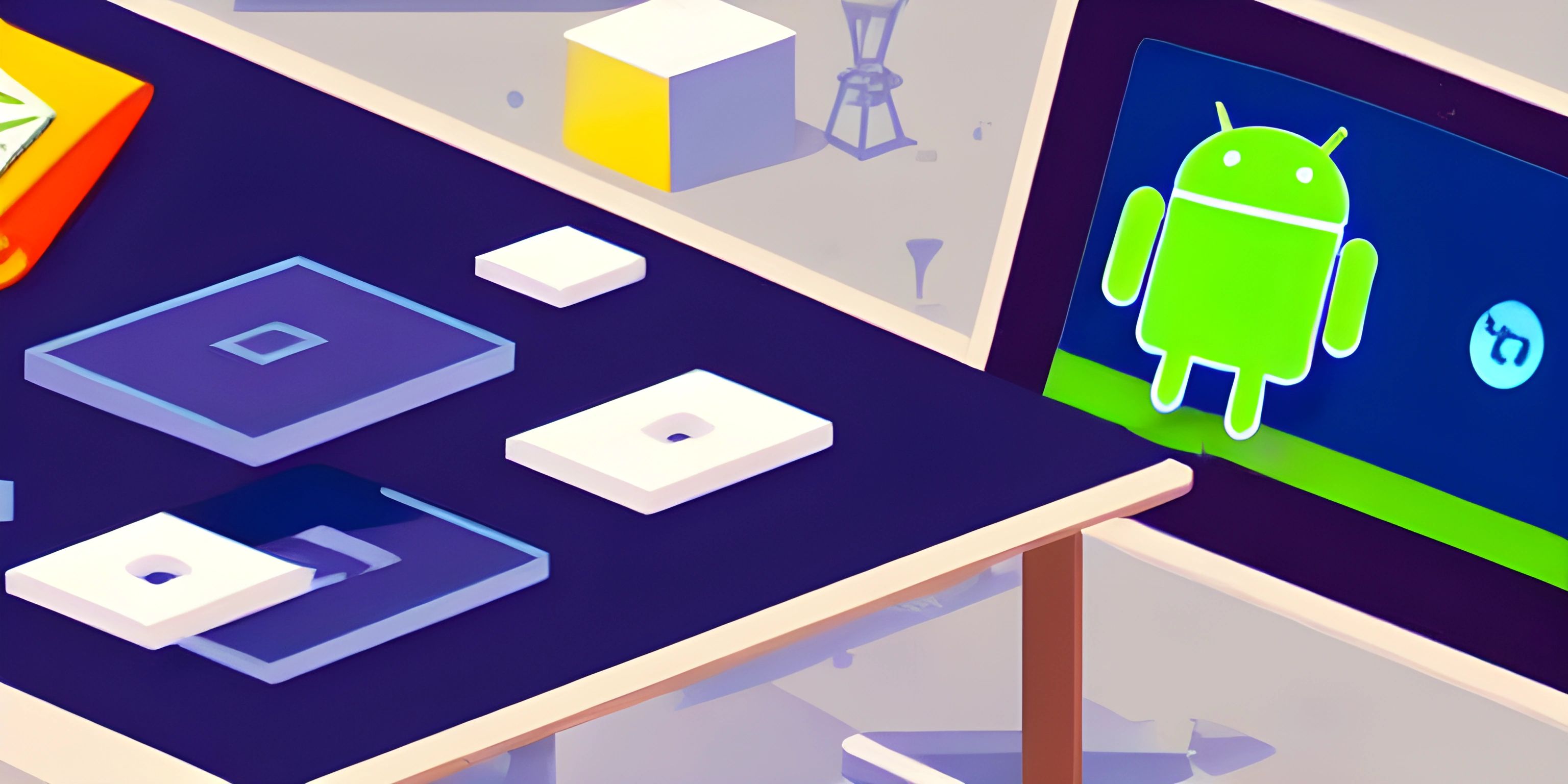
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you're an Android app developer or looking to become one, you've probably heard of Kotlin. It's a relatively new kid on the block, but it's one that's quickly making a name for itself. Kotlin is a modern, statically typed programming language that is fully interoperable with Java.
Why Kotlin?
Android developers have long been using Java to create apps. So why the sudden shift towards Kotlin? Well, it's not that sudden. Kotlin was designed to address some of the issues that developers face with Java, such as null pointer exceptions, boilerplate code, and more. It's no wonder that Google officially announced Kotlin as a preferred language for Android app development at Google I/O 2019.
Now let's dive into some fundamental Kotlin concepts.
Hello Kotlin
The first step in learning a new language is typically creating a "Hello, World!" program. In Kotlin, it's as straightforward as this:
fun main() { println("Hello, World!") }
This code declares a function named main
that takes no arguments and returns no value. The function prints out the string "Hello, World!" when executed.
Variables
In Kotlin, you can create variables using the var
(mutable) and val
(immutable) keywords. Here's how you can declare variables in Kotlin:
var mutable: String = "I can be changed" val immutable: String = "I cannot be changed"
For more details on variables in Kotlin, you can visit our comprehensive guide.
Functions
In Kotlin, functions are declared with the fun
keyword. Here is an example:
fun greet(name: String): String { return "Hello, $name" }
This function takes a string as input and returns a string. The function greeting is explained in more detail in our functions guide.
Null Safety
One of Kotlin's standout features is its built-in null safety. If you've programmed in Java, you're likely all too familiar with the dreaded NullPointerException. In Kotlin, you have to be explicit about whether a variable can hold a null value.
var canBeNull: String? = "I can be null" var cannotBeNull: String = "I cannot be null"
For a deep dive into null safety in Kotlin, check out our complete guide.
Kotlin for Android
Kotlin's modern language features, seamless interoperability with Java, and Google's strong backing make it a solid choice for Android app development. Understanding Kotlin fundamentals is a stepping stone in your journey to becoming an Android app developer.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Kotlin?
Kotlin is a statically typed programming language that is fully interoperable with Java. It's modern, concise, and safe, and it has been officially announced as a preferred language for Android app development by Google.
Why should I learn Kotlin for Android app development?
Kotlin addresses many issues that developers face with Java, such as null pointer exceptions and boilerplate code. Furthermore, it's officially supported by Google, which means you have access to a wealth of resources and a strong community.
What are some of the fundamental concepts in Kotlin?
Some of the fundamental concepts in Kotlin are functions, variables, and null safety. Functions are declared with the fun
keyword, variables are declared with var
(mutable) and val
(immutable), and null safety is built into the language to prevent NullPointerExceptions.
How is null safety handled in Kotlin?
In Kotlin, you have to be explicit about whether a variable can hold a null value. If a variable can hold a null value, it must be declared with a question mark after the type, like this: var canBeNull: String?
. If it cannot hold a null value, it is declared normally. This helps prevent null pointer exceptions.
Can I use Java and Kotlin together in my Android app?
Yes, Kotlin is fully interoperable with Java. This means you can use both Kotlin and Java in your Android app, and they will work seamlessly together. This is one of the reasons why Kotlin has been adopted so quickly by the Android community.