Android App Development with Java
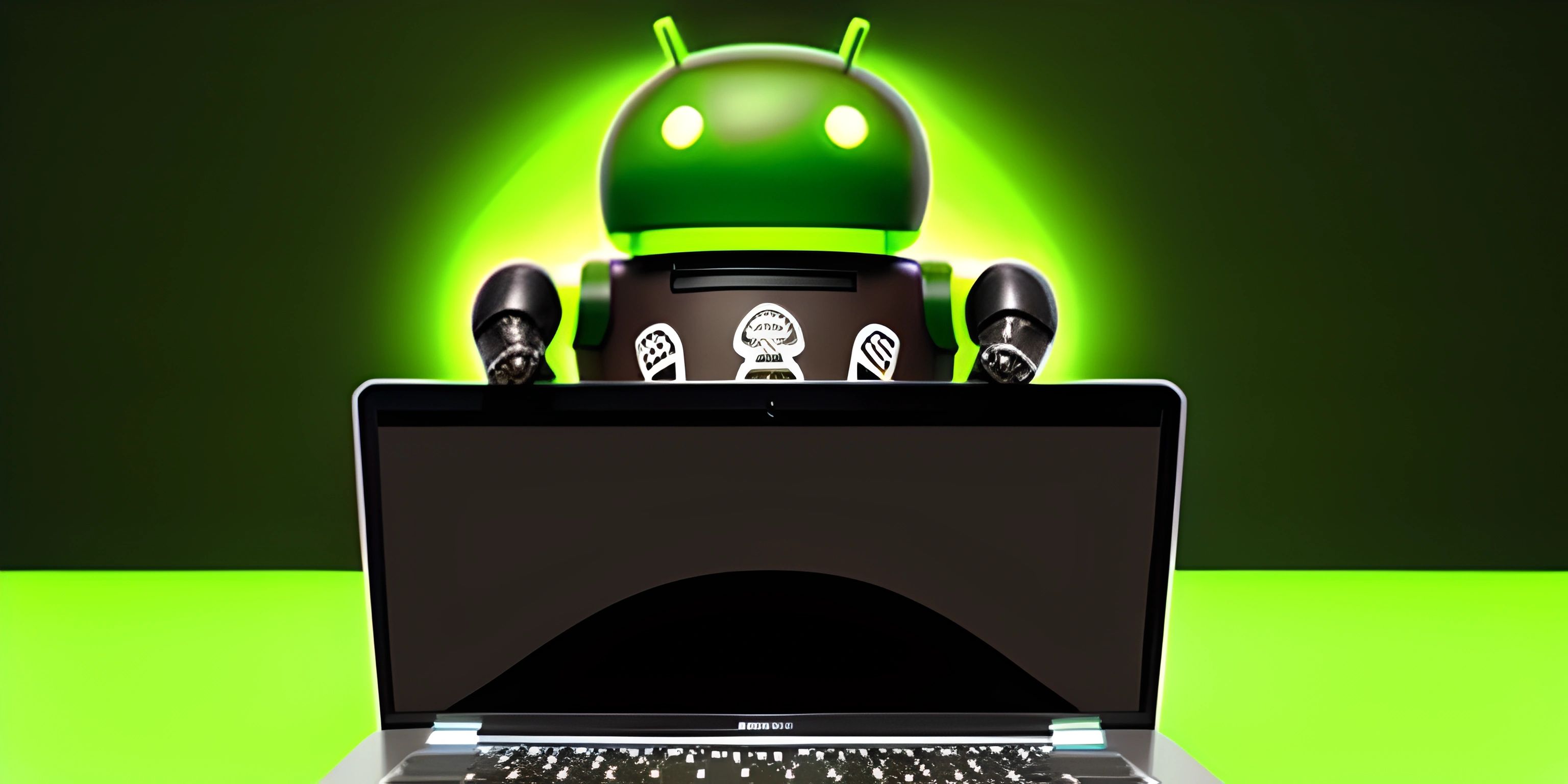
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Mobile devices are everywhere, and Android is one of the most popular operating systems in the world. With millions of users, the demand for well-designed, functional apps is high. To tap into this market, we're going to explore developing Android apps using Java and Android Studio.
Setting Up Your Environment
Before you can start building your Android app, you need to set up your development environment. You'll need to install Java Development Kit (JDK) and Android Studio, the official Android development environment, on your computer.
Once you have the JDK and Android Studio installed, you're ready to create your first Android project.
Creating a New Android Project
Open Android Studio and select "Create New Project". You'll be guided through a series of steps to configure your app:
- Choose a project template: Select the "Empty Activity" template for a basic project.
- Configure your project: Enter a name, package name, save location, language (Java), and minimum API level. The minimum API level determines the oldest Android version your app will support.
After you have configured your project, Android Studio will generate the necessary files and folders.
Understanding the Project Structure
Your new Android project has a specific structure, with several files and folders that serve different purposes. Let's explore some key components:
app/src/main/java/
: This folder contains the Java source files for your app, including theMainActivity.java
file, where you'll write most of your code.app/src/main/res/layout/
: This folder contains the XML files that define your app's user interface. Theactivity_main.xml
file corresponds to the main screen of your app.app/src/main/res/values/
: This folder holds XML files for organizing resources, such as strings, colors, and dimensions.
Building a Basic App
Now that you understand the project structure, let's create a simple app with a button and a text view. When the user taps the button, the text view will display a message.
- Open the
activity_main.xml
file and modify the layout by adding a button and a text view:
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tap me!" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="" app:layout_constraintBottom_toTopOf="@+id/button" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent"/> </androidx.constraintlayout.widget.ConstraintLayout>
- Next, open the
MainActivity.java
file and modify the code to handle button clicks:
package com.example.myapp; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Button button = findViewById(R.id.button); final TextView textView = findViewById(R.id.textView); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { textView.setText("Hello, Android!"); } }); } }
- Finally, click the "Run" button in Android Studio to test your app on an emulator or an actual Android device.
Congrats! You've just built a basic Android app using Java and Android Studio.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
How do I set up my environment for Android app development with Java?
First, you need to install the Java Development Kit (JDK) on your computer. Then, download and install Android Studio, the official Android development environment.
What is the minimum API level in an Android project?
The minimum API level determines the oldest version of Android that your app will support. When creating a new project in Android Studio, you'll have to choose a minimum API level based on your target audience and device compatibility.
What are the key components of an Android project structure?
The key components include the app/src/main/java/
folder for Java source files, the app/src/main/res/layout/
folder for XML layout files, and the app/src/main/res/values/
folder for organizing resources like strings, colors, and dimensions.
How do I create a basic Android app with a button and a text view?
First, modify the activity_main.xml
file to add a button and a text view to the layout. Next, update the MainActivity.java
file to handle button clicks and change the text view's content. Finally, run your app on an emulator or a real device to test it.