Introduction to Kotlin
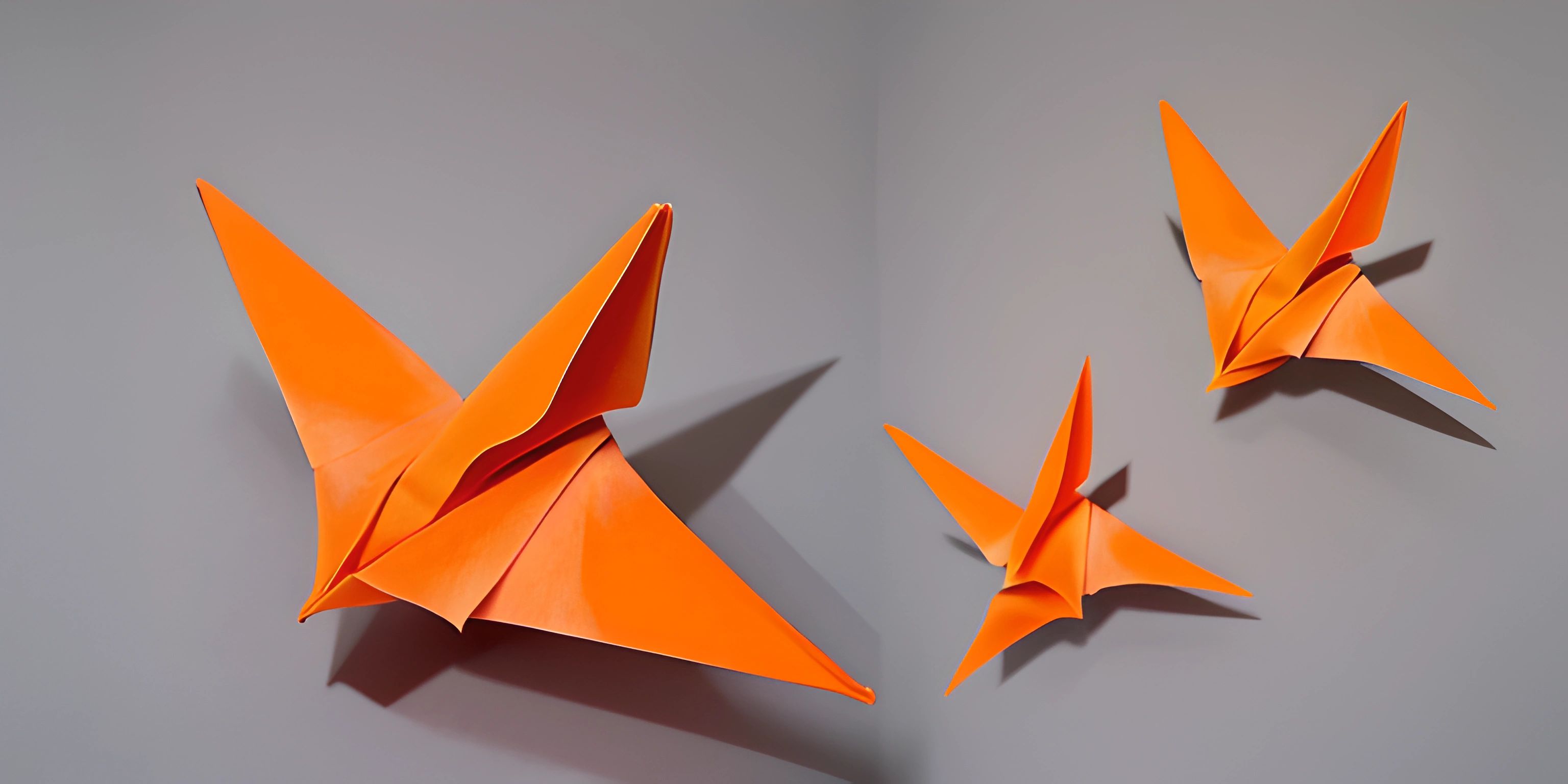
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Kotlin is a modern programming language that runs on the Java Virtual Machine (JVM) and is designed for simplicity and readability. Developed by JetBrains, Kotlin is gaining popularity for its concise syntax, interoperability with Java, and its use in Android app development.
Basic Syntax
Kotlin's syntax is easy on the eyes and shares similarities with other programming languages like Java, C#, and Swift. Let's start with the classic "Hello, World!" example:
fun main() { println("Hello, World!") }
Here, fun main()
is the entry point of our program, and println()
is a built-in function that prints the given text to the console.
Variables and Data Types
Kotlin has two types of variables: val
for immutable (read-only) and var
for mutable (changeable) variables. The language also has built-in data types like String
, Int
, Double
, and Boolean
. Here's an example:
val name: String = "CrateCoder" var age: Int = 25
In this example, name
is an immutable variable of type String
, while age
is a mutable variable of type Int
.
Control Structures
Kotlin offers a variety of control structures, such as if
, when
, for
, and while
. Here's an example of a simple if-else
statement:
if (age >= 18) { println("You are an adult.") } else { println("You are a minor.") }
This code checks if age
is greater than or equal to 18 and prints the appropriate message.
Functions
Functions in Kotlin are declared using the fun
keyword. You can define a function that takes parameters and returns a value like this:
fun greet(name: String): String { return "Hello, $name!" } val greeting = greet("CrateCoder") println(greeting)
In this example, the greet
function takes a String
parameter and returns a greeting message.
Kotlin and Java Interoperability
One of Kotlin's core strengths is its seamless interoperability with Java. You can call Java code from Kotlin and vice versa, making it easier to gradually migrate existing Java projects to Kotlin or use Kotlin libraries in Java projects.
Android Development
Kotlin has been officially supported by Google for Android app development since 2017. Its expressive syntax, null safety features, and Java compatibility make it a favorite among Android developers.
Conclusion
Kotlin is a powerful, expressive, and modern programming language that offers simplicity, readability, and excellent Java interoperability. Its growing popularity in Android development and other platforms makes it a valuable addition to any programmer's toolkit.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Kotlin and what makes it unique?
Kotlin is a modern, statically typed programming language that runs on the Java Virtual Machine (JVM). It was developed by JetBrains and is designed for simplicity, readability, and interoperability with Java. Kotlin's syntax is concise, expressive, and easy to learn, making it a popular choice for developers. It's also officially supported by Android, which makes it a great choice for building Android apps.
How does Kotlin's interoperability with Java work?
Kotlin was designed with Java interoperability in mind, which means you can use Java libraries and frameworks seamlessly alongside Kotlin code. Additionally, you can call Kotlin code from Java and vice versa, allowing you to gradually migrate to Kotlin or integrate Kotlin into an existing Java project. Kotlin's standard library also provides useful extensions to Java's standard classes, making it even more convenient to work with.
What are some key features of Kotlin?
Some notable features of Kotlin include:
- Null safety: Kotlin helps prevent NullPointerExceptions by distinguishing between nullable and non-nullable types at compile time.
- Extension functions: You can add new functionality to existing classes without modifying their source code.
- Smart casts: Kotlin automatically casts types when it's safe to do so, reducing the need for explicit casting.
- Data classes: Easily create classes that are intended to hold data, with automatically generated
equals()
,hashCode()
, andtoString()
methods. - Lambda expressions and higher-order functions: Kotlin supports functional programming constructs, making it easier to write concise, expressive code.
How do I get started with Kotlin development?
To start developing with Kotlin, you'll need to install the Kotlin compiler or an IDE that supports Kotlin, such as IntelliJ IDEA. You can also use the online Kotlin Playground for quick experimentation. Once your development environment is set up, you can start learning the language basics and syntax, explore Kotlin's standard library, and practice by building small projects. There are many resources available, including the official Kotlin documentation, online tutorials, and courses, to help you learn and master Kotlin.
Can I use Kotlin for web development?
Yes, Kotlin can be used for web development! In addition to running on the JVM, Kotlin can also be compiled to JavaScript, which means you can use it for both frontend and backend development. Kotlin/JS is a Kotlin-to-JavaScript transpiler that enables you to write web applications in Kotlin and leverage popular JavaScript libraries and frameworks. For backend development, you can use Kotlin with popular JVM-based web frameworks like Spring, Vert.x, or Ktor.