MATLAB Operators
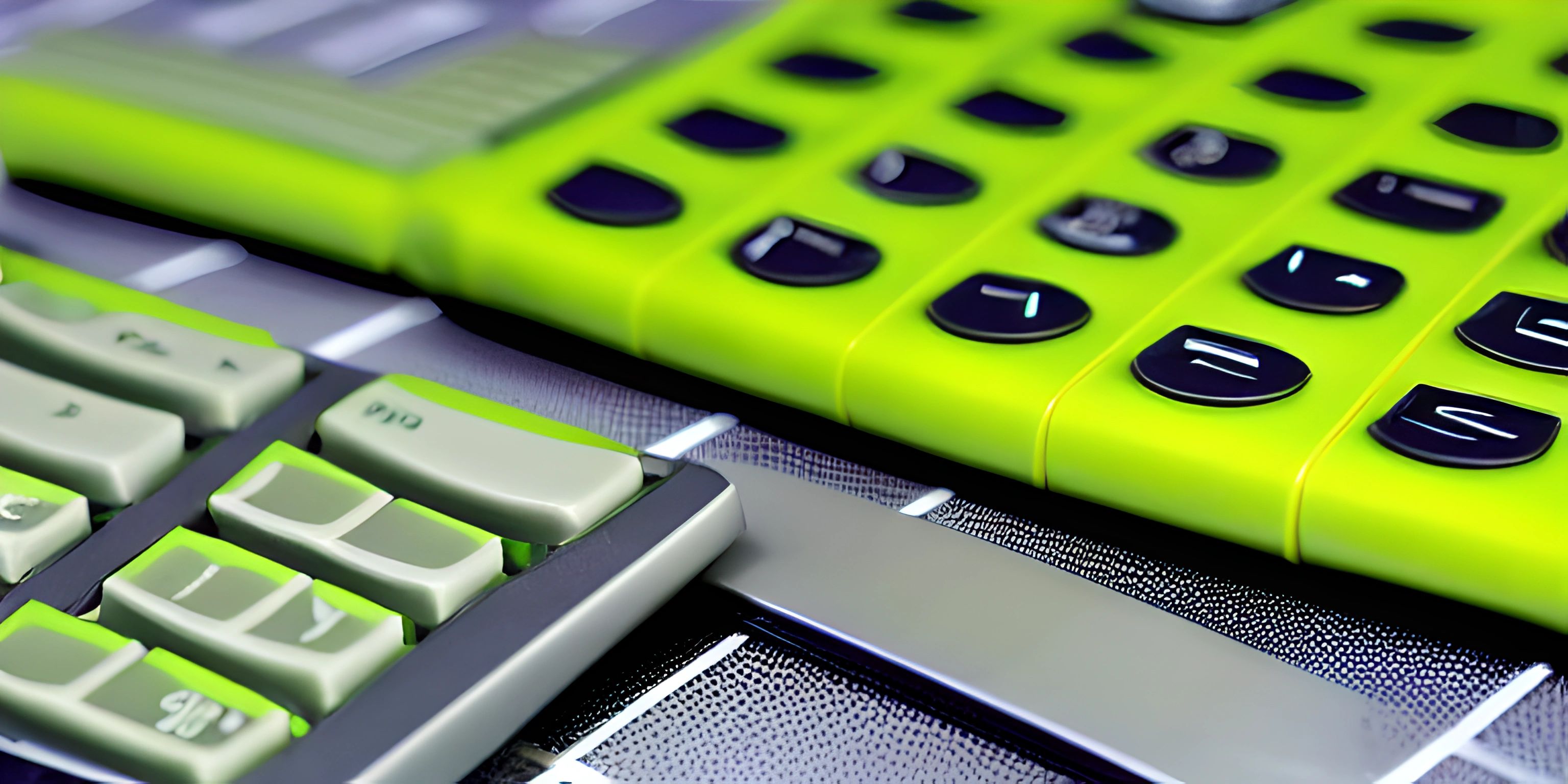
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Operators in MATLAB are used to perform various operations on variables, constants, and expressions. They can be categorized into arithmetic, relational, logical, and bitwise operators. Let's dive into each category and learn how to use them in MATLAB.
Arithmetic Operators
Arithmetic operators are used to perform mathematical operations. Here's a list of common arithmetic operators in MATLAB:
+
Addition-
Subtraction*
Multiplication/
Division^
Exponentiationmod
Modulus\
Left Division
Here's how to use some of these operators in MATLAB:
a = 5 + 3; % Addition b = 5 - 3; % Subtraction c = 5 * 3; % Multiplication d = 5 / 3; % Division e = 5 ^ 3; % Exponentiation f = mod(5, 3); % Modulus g = 5 \ 3; % Left Division
Relational Operators
Relational operators are used to compare values and return a logical value (true
or false
). Here's a list of common relational operators in MATLAB:
==
Equal to~=
Not equal to>
Greater than<
Less than>=
Greater than or equal to<=
Less than or equal to
Here's how to use some of these operators in MATLAB:
a = 5 == 3; % Returns false b = 5 ~= 3; % Returns true c = 5 > 3; % Returns true d = 5 < 3; % Returns false e = 5 >= 3; % Returns true f = 5 <= 3; % Returns false
Logical Operators
Logical operators are used to perform logical operations on boolean values (true
or false
). Here's a list of common logical operators in MATLAB:
&&
Logical AND||
Logical OR~
Logical NOT
Here's how to use some of these operators in MATLAB:
a = true && false; % Returns false b = true || false; % Returns true c = ~true; % Returns false
Bitwise Operators
Bitwise operators are used to perform operations on integers at the bit level. Here's a list of common bitwise operators in MATLAB:
&
Bitwise AND|
Bitwise OR~
Bitwise NOT^
Bitwise XORbitshift
Bitwise Shift
Here's how to use some of these operators in MATLAB:
a = 5 & 3; % Returns 1 (bitwise AND) b = 5 | 3; % Returns 7 (bitwise OR) c = ~5; % Returns -6 (bitwise NOT) d = 5 ^ 3; % Returns 6 (bitwise XOR) e = bitshift(5, 2); % Returns 20 (bitwise shift left)
Now you have an understanding of different types of operators in MATLAB and how to use them. Make sure to practice, and you'll be a MATLAB operator ninja in no time!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Supporting All Operations (psst, it's free!).
FAQ
What are the four categories of operators in MATLAB?
The four categories of operators in MATLAB are arithmetic, relational, logical, and bitwise operators.
How do you perform addition and subtraction in MATLAB?
In MATLAB, you can perform addition and subtraction using the +
and -
operators, respectively. For example, a = 5 + 3
for addition, and b = 5 - 3
for subtraction.
What is the purpose of relational operators in MATLAB?
Relational operators in MATLAB are used to compare values and return a logical value (true
or false
) based on the comparison result.
How can I perform bitwise XOR operation in MATLAB?
To perform the bitwise XOR operation in MATLAB, use the ^
operator. For example, d = 5 ^ 3
will perform bitwise XOR on the numbers 5 and 3.