MATLAB Data Types
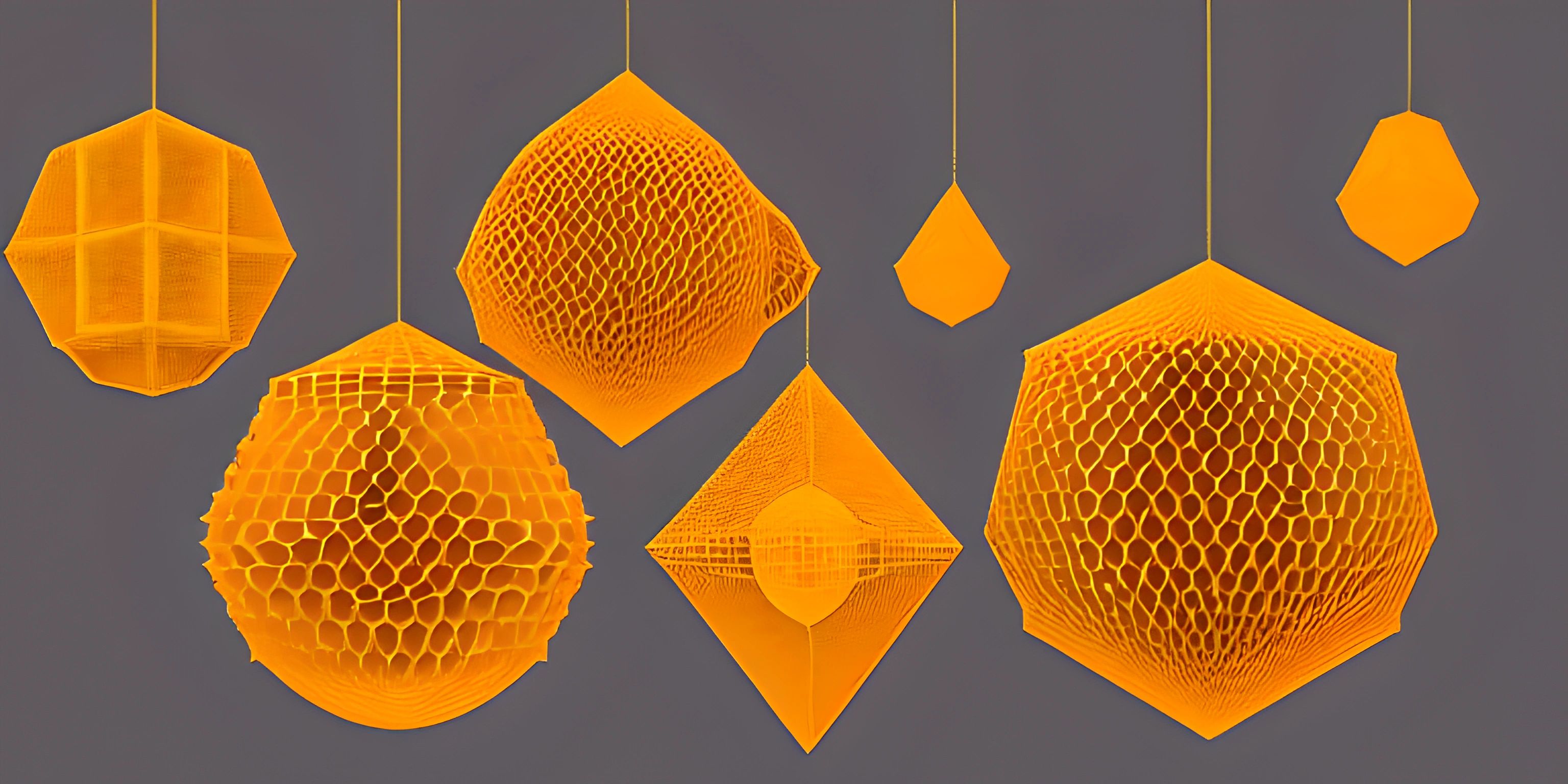
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
MATLAB is a programming language widely used in engineering, mathematics, and scientific research. One key aspect of any programming language is the ability to store and manipulate various data types. In this article, we'll look at different data types that MATLAB offers and how to work with them effectively.
Numeric Data Types
MATLAB is widely known for its capabilities in numerical computing, so it's no surprise that it has a variety of numeric data types. There are two main categories of numeric data types in MATLAB: floating-point and integer.
Floating-point
Floating-point numbers are used to represent real numbers, and MATLAB supports single-precision (single
) and double-precision (double
) floating-point numbers. By default, MATLAB uses double-precision numbers. Here's an example:
a = 3.14159265359; b = single(a);
In this example, a
is a double-precision floating-point number, while b
is a single-precision version of a
. Converting to single-precision can save memory, but at the cost of reduced precision.
Integer
MATLAB supports several integer data types, including int8
, int16
, int32
, and int64
. Additionally, there are the unsigned counterparts: uint8
, uint16
, uint32
, and uint64
. Here's an example of working with integers:
c = int32(42); d = uint8(255);
In this example, c
is a 32-bit signed integer, while d
is an 8-bit unsigned integer.
Logical Data Type
The logical data type in MATLAB represents boolean values, i.e., true
and false
. These are used in conditional statements and logical operations. Here's an example:
e = true; f = (3 > 2); % f will be true since 3 is greater than 2
Character and String Data Types
MATLAB offers two ways to represent text: characters and strings. Characters are single Unicode characters, while strings are sequences of characters. You can create a character array using single quotes ('
) and a string using double quotes ("
).
g = 'Hello world!'; h = "Hello world!";
In this example, g
is a character array, while h
is a string. Strings are generally recommended over character arrays for modern MATLAB code, as they offer more natural and consistent behavior.
Cell Arrays and Structures
MATLAB provides two data types for storing heterogeneous data: cell arrays and structures.
Cell Arrays
A cell array is an array where each element can contain data of any type. You can create a cell array using the cell
function or by using curly braces ({}
). Here's an example:
i = {'apple', 42, true};
Here, i
is a cell array containing a string, an integer, and a logical value.
Structures
Structures in MATLAB are similar to cell arrays, but they offer named fields, making the data easier to understand and organize. You can create structures using the struct
function or by using the dot (.
) syntax. Here's an example:
j.name = 'John Doe'; j.age = 30; j.isStudent = false;
Here, j
is a structure with fields name
, age
, and isStudent
.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Data Types (psst, it's free!).
FAQ
What are the main data types in MATLAB?
The main data types in MATLAB include numeric (floating-point and integer), logical, character, string, cell array, and structure.
What is the difference between a character array and a string in MATLAB?
A character array in MATLAB is a sequence of characters created using single quotes, while a string is a sequence of characters created using double quotes. Strings offer more natural and consistent behavior and are recommended for modern MATLAB code.
How are cell arrays and structures used in MATLAB?
Cell arrays and structures in MATLAB are used to store heterogeneous data, i.e., data of different types. Cell arrays store data in a simple array format, while structures store data with named fields, making it easier to organize and understand.
What is the difference between a single-precision and double-precision floating-point number in MATLAB?
In MATLAB, a single-precision floating-point number (single
) has less memory usage and less precision compared to a double-precision floating-point number (double
). By default, MATLAB uses double-precision numbers.