JavaScript String Comparison
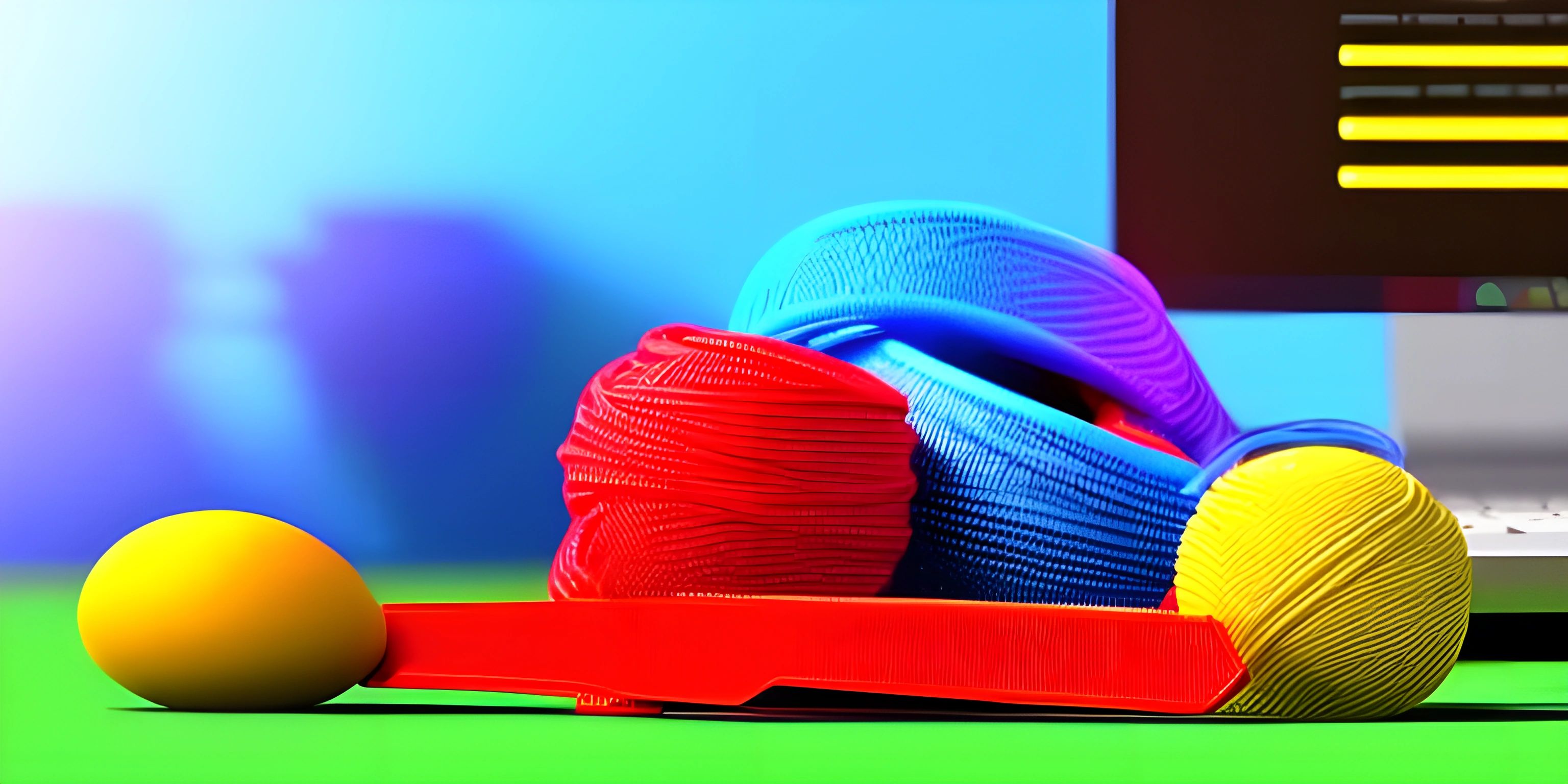
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When working with JavaScript, there's a good chance you'll need to compare two strings at some point. Whether you're sorting names, checking passwords, or making sure two URLs match, string comparison is a common task in programming.
String Equality
JavaScript provides several ways to check if two strings are equal. The simplest way is by using the equality operator (==
). This operator checks if the two strings have the same characters in the same order, regardless of whether they're stored in different variables or if they're hardcoded strings.
var string1 = "Hello, World!"; var string2 = "Hello, World!"; console.log(string1 == string2); // prints 'true'
In the above code, string1
and string2
hold the same characters, so the equality operator returns true
.
However, JavaScript also provides the identity operator (===
). This operator not only checks if the strings are identical, but also if they're of the same type. Since JavaScript is a dynamically-typed language, meaning variables can hold values of any type, this distinction can be important.
var string1 = "100"; var string2 = 100; console.log(string1 === string2); // prints 'false' console.log(string1 == string2); // prints 'true'
Even though the variables string1
and string2
both hold values that "look like" numbers, the identity operator checks their types and returns false
because string1
is a string and string2
is a number.
Lexicographic Comparison
When it comes to comparing strings lexicographically (i.e., alphabetically), things get a bit more complex. JavaScript uses the <
, >
, <=
, and >=
operators to compare strings based on their Unicode values.
var string1 = "apple"; var string2 = "banana"; console.log(string1 < string2); // prints 'true'
In this case, "apple" comes before "banana" in the dictionary, so string1 < string2
returns true
.
However, these operators don't always behave as you might expect because they're comparing Unicode values rather than English dictionary order. For example, capital letters have lower Unicode values than lowercase letters, so "Apple" will come before "banana" lexicographically.
var string1 = "Apple"; var string2 = "banana"; console.log(string1 < string2); // prints 'true'
In conclusion, JavaScript provides several ways to compare strings, but it's important to understand how these comparison operators work to avoid unexpected behavior. Always consider the type of comparison you need to make and choose the appropriate operator for your use case.
FAQ
How can I check if two strings are equal in JavaScript?
You can check if two strings are equal using the equality operator (==
), which checks if the two strings have the same characters in the same order. JavaScript also provides the identity operator (===
), which checks if the strings are identical and of the same type.
How does JavaScript compare strings lexicographically?
JavaScript uses the <
, >
, <=
, and >=
operators to compare strings based on their Unicode values. This means that "apple" comes before "banana", but it also means that "Apple" comes before "banana" because capital letters have lower Unicode values than lowercase letters.
What's the difference between the equality operator and the identity operator?
The equality operator (==
) checks if the two strings have the same characters in the same order, while the identity operator (===
) also checks if they're of the same type. This distinction can be important in JavaScript, which is a dynamically-typed language.
Do the lexicographic comparison operators in JavaScript work like they do in the dictionary?
Not always. JavaScript's lexicographic comparison operators compare strings based on their Unicode values, not English dictionary order. This can lead to unexpected results, like "Apple" coming before "banana".