Exploring MongoDB for Web Development
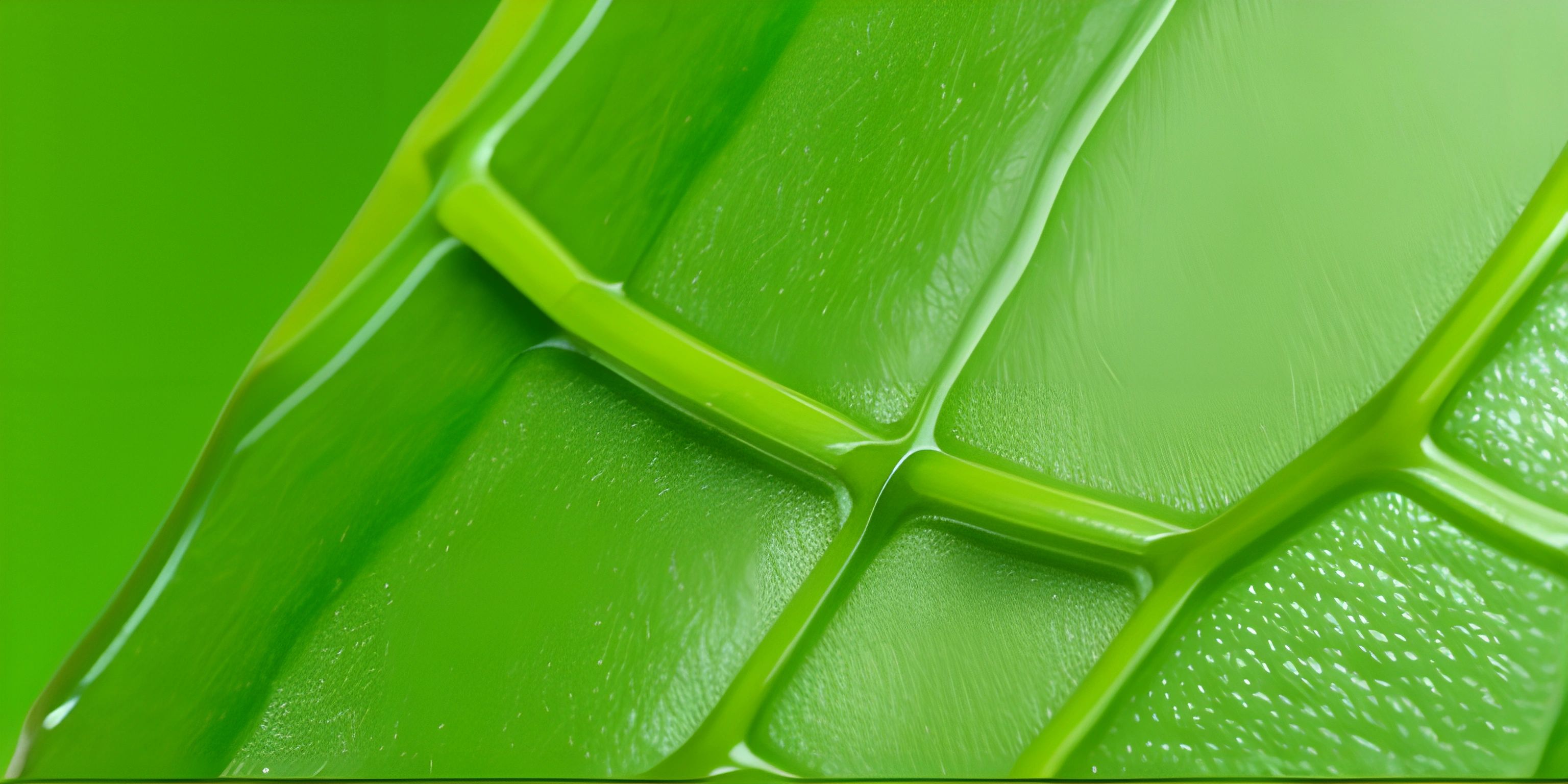
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
MongoDB is a popular NoSQL database that has become a go-to choice for many web developers. It's known for its flexibility, scalability, and performance, making it suitable for projects of all sizes. In this introduction, we'll explore the basics of MongoDB and see how it can be a valuable asset in web development.
What is MongoDB?
MongoDB is a NoSQL database, which means it doesn't follow the traditional relational database model. Instead of using tables, rows, and columns, MongoDB stores data in BSON (Binary JSON) format, which is similar to JSON but supports more data types. BSON documents are stored in collections, which can be thought of like folders for your documents. This flexible structure allows for easy changes in the schema and rapid development.
Features of MongoDB
MongoDB boasts several features that make it attractive for web development:
Schemaless
As mentioned earlier, MongoDB doesn't enforce a strict schema for its collections. This allows developers to modify the data structure as needed without having to worry about changing the database schema.
Scalability
MongoDB can handle a large amount of data and is designed to scale horizontally. This means it can distribute data across multiple servers, making it a great choice for applications that need to handle a lot of concurrent users or large datasets.
Performance
MongoDB is optimized for fast reads and writes, which is essential for responsive web applications. Its indexing capabilities and in-memory storage engine help to achieve high query performance.
Geospatial Support
MongoDB has built-in support for geospatial data, making it ideal for location-based applications.
Using MongoDB in Web Development
To use MongoDB in your web development projects, you'll first need to install MongoDB on your development environment.
Next, choose a programming language and a relevant driver for MongoDB. For example, if you're using Node.js, you'll want the MongoDB Node.js driver.
Once you have the driver installed, you can start interacting with MongoDB in your web application. Here's a simple example using Node.js and the MongoDB driver:
const MongoClient = require("mongodb").MongoClient; // Connection URL const url = "mongodb://localhost:27017"; // Database name const dbName = "mydb"; // Connect to the server MongoClient.connect(url, function (err, client) { if (err) throw err; console.log("Connected successfully to MongoDB"); // Select the database const db = client.db(dbName); // Insert a document const exampleDocument = { name: "John Doe", age: 30, occupation: "Software Developer" }; db.collection("users").insertOne(exampleDocument, function (err, res) { if (err) throw err; console.log("Document inserted"); // Close the connection client.close(); }); });
In this example, we connect to a local MongoDB server, select a database named mydb
, and insert a document into the users
collection.
Conclusion
MongoDB's flexibility, scalability, and performance make it a popular choice for web development projects. Its schemaless design and support for geospatial data further add to its appeal. By learning about MongoDB and incorporating it into your web development toolkit, you'll be well-equipped to create flexible and fast web applications.