Working with MongoDB Driver for Node.js
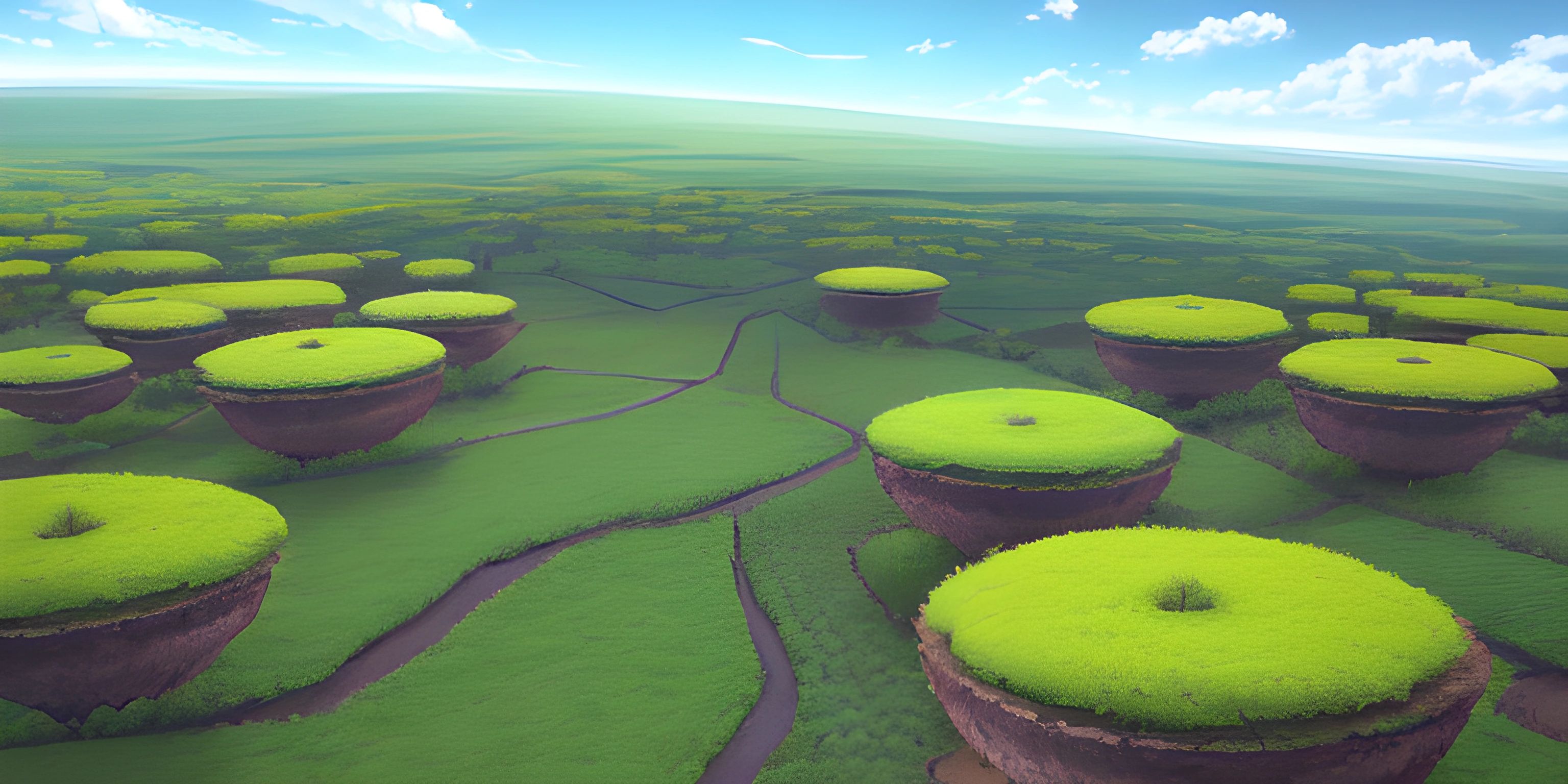
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
MongoDB is a popular NoSQL database, perfect for developers who need flexibility and scalability. And when you pair it with Node.js, you get a powerful combo that can tackle any challenge. But to make these two play nice together, we need a translator, a middleman. Enter the MongoDB driver for Node.js.
MongoDB and Node.js: A Quick Intro
MongoDB is a document-oriented database that stores data in a flexible, JSON-like format. It's excellent for dealing with large amounts of data and can handle a variety of data types.
On the other hand, Node.js is a JavaScript runtime that allows you to build scalable network applications. It's loved for its fast performance and being JavaScript-based, making it a go-to for many web developers.
The MongoDB Driver
The MongoDB driver for Node.js is essentially the bridge that allows Node.js applications to communicate with MongoDB. It acts as an interpreter, allowing your JavaScript code to communicate with MongoDB in a language it understands.
Here's a simple example that shows how to connect to a MongoDB database using the Node.js driver:
const MongoClient = require("mongodb").MongoClient; const uri = "mongodb+srv://<username>:<password>@cluster0.mongodb.net/test?retryWrites=true&w=majority"; const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true }); client.connect(err => { const collection = client.db("test").collection("devices"); // perform actions on the collection object client.close(); });
This script imports the MongoDB client, then connects to a MongoDB database. It then opens a collection (like a table in a relational database), and finally closes the connection.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is the MongoDB driver for Node.js?
The MongoDB driver for Node.js is a tool that allows Node.js applications to interact with MongoDB databases. It acts as an interpreter between the JavaScript code in your Node.js application and the MongoDB database, converting commands and data back and forth between formats that each can understand.
How do I connect to a MongoDB database using the Node.js driver?
To connect to a MongoDB database, you first need to import the MongoDB client using require("mongodb").MongoClient
. Then, you create a new MongoClient object, passing in your MongoDB URI and an options object. Finally, you use the .connect()
method on the MongoClient object to connect to the database.
Why would I use MongoDB with Node.js?
MongoDB and Node.js are a common pairing in the world of web development. They both work well with JavaScript, making the combination a natural fit for many web developers. Moreover, MongoDB's flexible and scalable nature makes it a great choice for many types of applications, and Node.js's ability to handle many requests simultaneously makes it a good fit for web services and applications.