NestJS Introduction
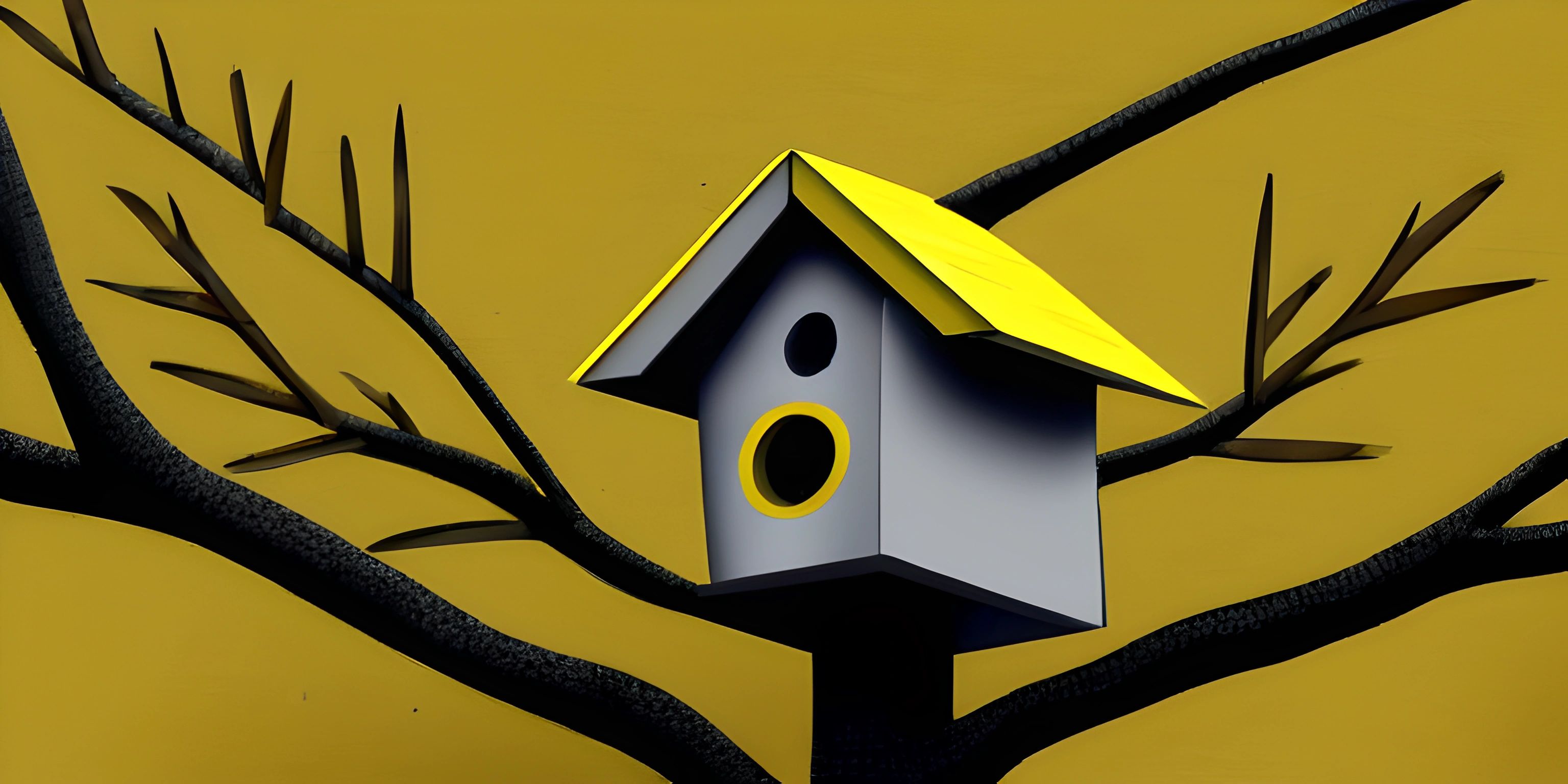
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
NestJS is a powerful back-end framework for building efficient, scalable, and maintainable Node.js applications. It's built on top of TypeScript, which provides a strong foundation for developing modern, statically-typed applications. One of the key features of NestJS is its highly modular architecture that encourages developers to follow best practices and create well-organized, reusable components.
Why NestJS?
NestJS is designed to help developers create highly testable, scalable, and loosely-coupled applications. It combines the best aspects of object-oriented programming, functional programming, and reactive programming, while leveraging popular libraries and tools like Express and RxJS. In other words, NestJS is a one-stop-shop for developing modern, versatile, and maintainable applications.
NestJS Architecture
The architecture of NestJS is highly inspired by Angular, which makes it easy for developers with Angular experience to pick it up quickly. The main building blocks of a NestJS application are modules, controllers, and providers.
Modules
A module in NestJS is a class decorated with @Module()
. It serves as a container for organizing related components, such as controllers and providers, in a coherent way. Modules help to keep the application structure clean and maintainable.
import { Module } from "@nestjs/common"; import { ExampleController } from "./example.controller"; import { ExampleService } from "./example.service"; @Module({ controllers: [ExampleController], providers: [ExampleService], }) export class ExampleModule {}
Controllers
A controller in NestJS is responsible for handling incoming HTTP requests and returning a response. Controllers are classes decorated with @Controller()
, and they define the routes and request handlers for the application.
import { Controller, Get } from "@nestjs/common"; @Controller("example") export class ExampleController { @Get() getExample(): string { return "Hello from the ExampleController!"; } }
Providers
Providers in NestJS are classes, values, or factories that can be injected into other components, such as controllers or other providers. They are used to encapsulate and share functionality across the application. Providers are decorated with @Injectable()
when they are classes.
import { Injectable } from "@nestjs/common"; @Injectable() export class ExampleService { getMessage(): string { return "Hello from the ExampleService!"; } }
Getting Started with NestJS
To start building an application with NestJS, you'll need to have Node.js and TypeScript installed on your machine. You can then use the NestJS CLI to generate a new project:
npm i -g @nestjs/cli nest new my-nestjs-project
This will create a new NestJS project with a basic structure, including a root module, a sample controller, and a sample provider. From there, you can begin to explore the many powerful features and libraries that NestJS has to offer.
As you can see, NestJS provides a robust and scalable foundation for building modern, maintainable Node.js applications. Its modular architecture, inspired by Angular, ensures that your code stays clean and well-organized. So, put on your NestJS hat and start building the next big thing!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Cratecode Playground (psst, it's free!).
FAQ
What is NestJS?
NestJS is a progressive Node.js framework for building efficient, reliable, and scalable server-side applications. It uses modern JavaScript, is built with TypeScript, and combines elements of OOP (Object Oriented Programming), FP (Functional Programming), and FRP (Functional Reactive Programming). Nest provides an out-of-the-box application architecture that allows developers to create highly testable, scalable, and easily maintainable applications.
How does NestJS architecture work?
NestJS architecture is based on a modular structure, which organizes the application into separate modules. Each module is responsible for a specific part of the application's functionality. The main building blocks of NestJS architecture are:
- Modules: They are used to group related components, providers, and other modules.
- Controllers: They are responsible for handling incoming HTTP requests and returning responses to the client.
- Providers: They are services, repositories, or factories that can be injected into other components, making the application more modular and efficient.
- Middleware: They are functions that can execute before the request reaches the controller, typically used for tasks like logging or authentication.
- Pipes, Guards, and Interceptors: These are additional components that can be used to manipulate the request/response cycle and enforce specific conditions.
How do I get started with NestJS?
To get started with NestJS, you need to have Node.js (version 10 or higher) and npm installed on your system. Then, you can install the Nest CLI globally by running the following command:
npm i -g @nestjs/cli
Once the CLI is installed, you can create a new NestJS project using the command:
nest new project-name
This will create a new directory named project-name
with a basic NestJS application. Change to the project directory and run the application using the following commands:
cd project-name npm run start
Now you're ready to explore and build your NestJS application!
Can I use NestJS with other databases?
Yes, NestJS is highly extensible and can be easily integrated with various databases, including both SQL and NoSQL databases. Some popular choices include PostgreSQL, MySQL, MongoDB, and Redis. NestJS provides a dedicated package called @nestjs/typeorm
for working with TypeORM, which is a popular Object Relational Mapper (ORM) supporting various databases. Additionally, you can use other ORMs or database drivers according to your needs.
What are some best practices for working with NestJS?
Some best practices for working with NestJS include:
- Use the modular structure provided by NestJS, keeping related components and providers within their respective modules.
- Follow the Single Responsibility Principle, ensuring each component or provider only has one responsibility.
- Use Dependency Injection (DI) to manage dependencies between components and providers.
- Write tests for your application using the built-in testing utilities provided by NestJS.
- Follow the official NestJS documentation and community guidelines to keep up-to-date with the latest best practices and recommendations.