Node.js Readline Basics
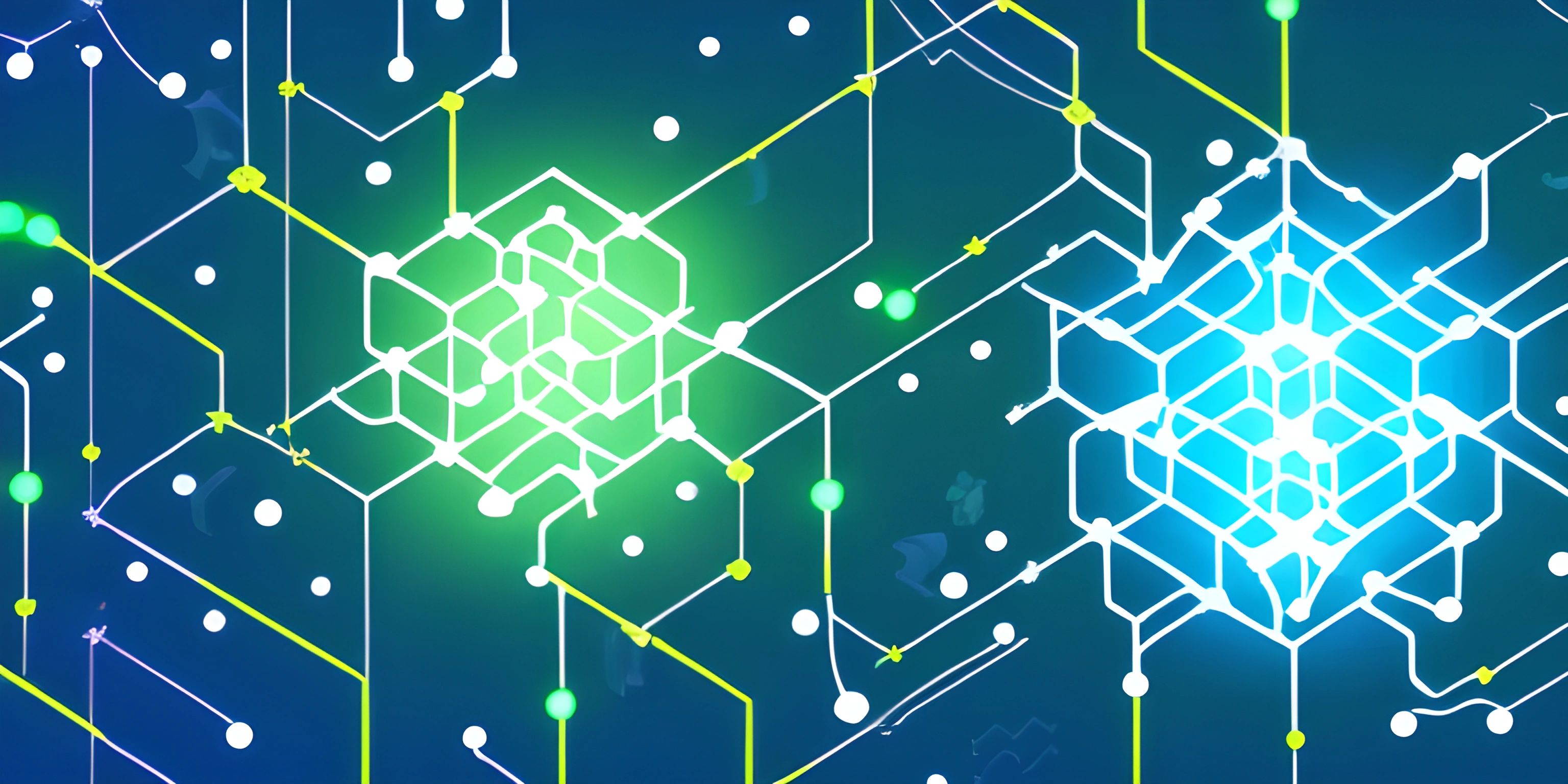
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The readline
module in Node.js provides an easy way to interact with the command line and get user input. It can be used to read data from a readable stream (like process.stdin
) one line at a time. In this article, we will cover the basics of using the readline
module to get user input in a Node.js application.
Importing the Readline Module
First, you need to import the readline
module. You can do this using the require
function:
const readline = require("readline");
Creating a Readline Interface
Next, you need to create an instance of the readline.Interface
class. This class represents a readline interface and provides methods for reading and writing data to a readable and writable stream. You can create an instance of this class using the readline.createInterface()
method:
const rl = readline.createInterface({ input: process.stdin, output: process.stdout });
Here, we pass an object with two properties: input
and output
. The input
property should be a readable stream, and the output
property should be a writable stream. In this case, we use process.stdin
and process.stdout
, which represent the standard input and output streams, respectively.
Reading User Input
To read a line of user input, you can use the rl.question()
method. This method takes two arguments: a prompt to display to the user and a callback function to execute when the user enters their input. The callback function will receive the user input as its first argument:
rl.question("What is your name? ", (name) => { console.log(`Hello, ${name}!`); rl.close(); });
Here, we display the prompt "What is your name?" and wait for the user to enter their input. When they do, we print a greeting with their name and close the readline interface using the rl.close()
method.
Closing the Readline Interface
It's important to close the readline interface when you're done using it. This releases the resources associated with the interface and allows the Node.js process to exit. You can close the interface using the rl.close()
method, as shown in the previous example.
Full Example
Here's a complete example that demonstrates how to use the readline module to get user input in Node.js:
const readline = require("readline"); const rl = readline.createInterface({ input: process.stdin, output: process.stdout }); rl.question("What is your name? ", (name) => { console.log(`Hello, ${name}!`); rl.close(); });
Now you have a basic understanding of how to use the readline
module in Node.js to get user input. You can use this knowledge to create more interactive command-line applications and gather information from users as needed.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: File I/O (Reading) (psst, it's free!).
FAQ
What is the readline module in Node.js?
The readline module in Node.js is a built-in module that provides an interface for reading data from a readable stream (like process.stdin
) one line at a time. It's commonly used to get user input in command-line applications.
How do I use the readline module in my Node.js project?
To use the readline module in your Node.js project, you need to first require it. Here's an example of how to create a basic readline interface:
const readline = require("readline"); const rl = readline.createInterface({ input: process.stdin, output: process.stdout });
Now you can use rl
to read lines of input from the user, and write output to the console.
How do I ask the user for input using the readline module?
To ask the user for input using the readline module, you can use the question
method on the readline interface (e.g. rl.question
). Here's an example:
rl.question("What's your name? ", (answer) => { console.log(`Hello, ${answer}!`); rl.close(); });
This code asks the user for their name and then greets them with their entered name.
How do I handle errors and events in the readline module?
The readline interface is an EventEmitter
, which means you can handle events like 'line' and 'close' using the on
method. Here's an example that demonstrates handling events:
rl.on("line", (input) => { console.log(`Received input: ${input}`); }); rl.on("close", () => { console.log("Readline interface closed."); });
This code listens for when a user enters a line of input and when the readline interface is closed.
Can I customize the readline interface prompts and appearance?
Yes, you can customize the readline interface prompts and appearance by modifying the options passed to readline.createInterface()
. For example, you can change the prompt to display a custom string like this:
const rl = readline.createInterface({ input: process.stdin, output: process.stdout, prompt: "My Custom Prompt > " }); rl.prompt();
Now, the prompt will display "My Custom Prompt > " instead of the default "> ".