TypeScript Language Basics
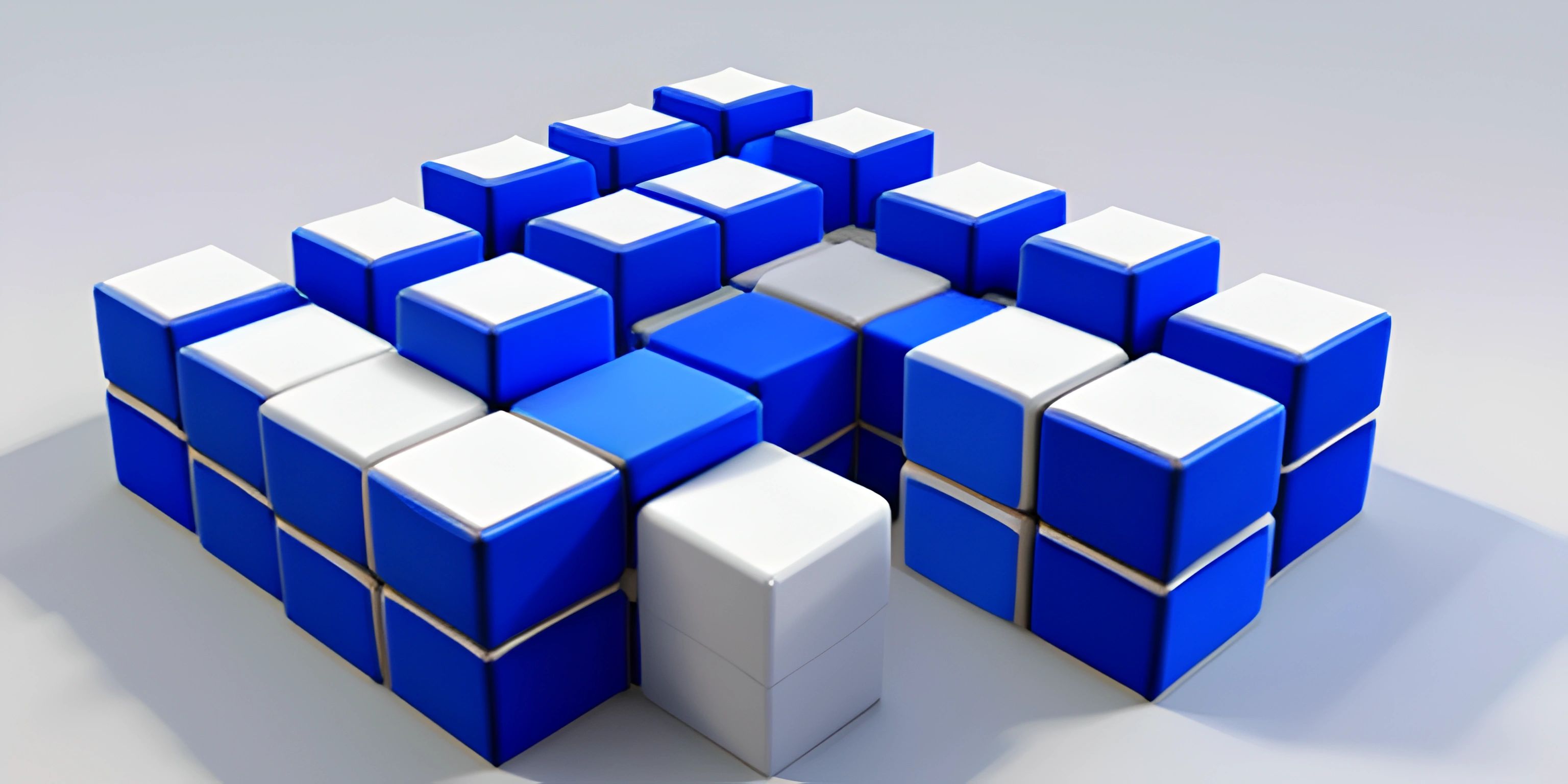
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the world of TypeScript! TypeScript is a powerful, statically-typed superset of JavaScript that allows developers to build robust and maintainable applications. This introduction to TypeScript will cover the basics of its syntax, types, and other language features that make it a popular choice for developers.
TypeScript vs JavaScript
Before we dive into TypeScript, let's quickly understand how it relates to JavaScript. TypeScript is a superset of JavaScript, meaning that all valid JavaScript code is also valid TypeScript code. However, TypeScript adds additional features and type information that help catch potential issues in your code before it runs. This makes your code safer and more predictable.
TypeScript code is compiled into JavaScript, which is then executed by the browser or server-side runtime like Node.js. This means that TypeScript is not directly executed but instead acts as a layer of safety and structure on top of JavaScript.
Basic Syntax
TypeScript syntax builds on the familiar syntax of JavaScript. Here's a simple example of TypeScript code:
function greet(name: string): void { console.log(`Hello, ${name}!`); } greet("TypeScript");
In this example, we have a greet
function that takes a single parameter name
of type string
and returns nothing (void
). The syntax for specifying the type of a variable is done by adding a colon and the type right after the variable name, like name: string
.
Basic Types
TypeScript comes with a range of built-in types that allow you to be specific about the data your functions and variables work with. Some of the most common types are:
string
: Represents a sequence of characters, like"hello world"
.number
: Represents a numeric value, like42
or3.14
.boolean
: Represents a true or false value.
Here's an example of using these basic types in TypeScript:
let firstName: string = "John"; let age: number = 30; let isStudent: boolean = false; console.log(`${firstName} is ${age} years old and is a student: ${isStudent}`);
Advanced Types
TypeScript provides more advanced types and type manipulation features, like:
- Arrays: Using the
[]
syntax, you can create an array of a specific type, likenumber[]
orstring[]
.
let favoriteNumbers: number[] = [3, 7, 42];
- Tuples: A tuple is a fixed-length array with specific types for each element.
let personInfo: [string, number] = ["Alice", 25];
- Enums: Enumerations allow you to define a set of named constants.
enum Color { Red, Green, Blue, } let favoriteColor: Color = Color.Blue;
- Any: The
any
type is used when you don't know the type of a variable or when you want to opt out of type checking.
let mysteryValue: any = "this could be anything";
- Unknown: Similar to
any
, theunknown
type is used for values you don't know the types of, but with stricter type checking.
let unknownValue: unknown = "this could also be anything";
These are just a few examples of the many types and features TypeScript offers. As you dive deeper into TypeScript, you'll discover more advanced types like interface
, type alias
, and utility types that help you model your application in a safe and flexible way.
Conclusion
This brief introduction to TypeScript has covered the basic syntax, types, and some more advanced features. TypeScript is a powerful language that builds on JavaScript's foundation, making it a popular choice for developers who want to write safer and more robust code. As you continue exploring TypeScript, you'll find more advanced features and patterns to help you write clean, maintainable applications. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Advanced Data Types (psst, it's free!).