Exploring Next.js and Its Features
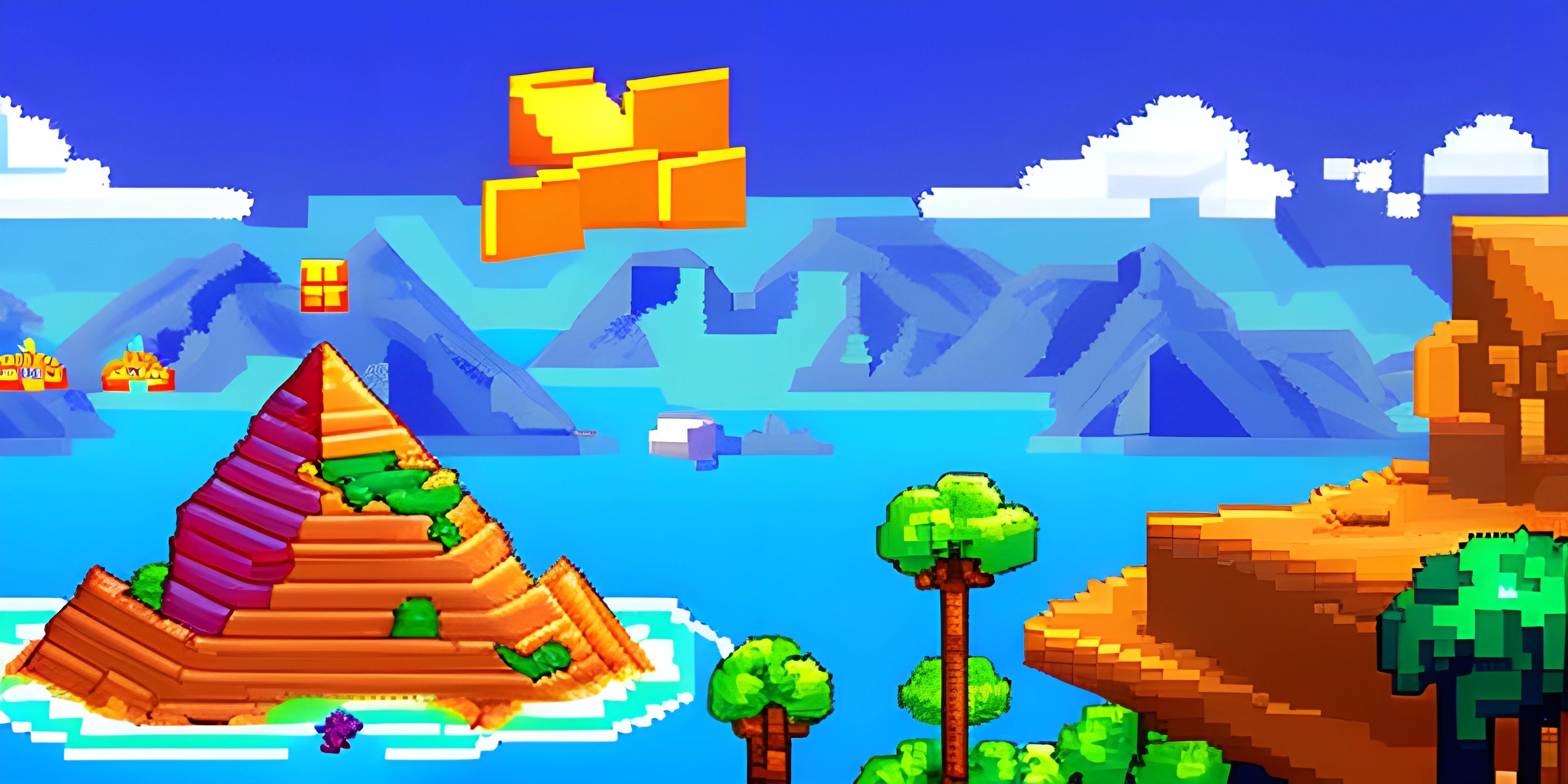
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever feel like you're at a buffet and can't decide between the juicy steak and the tantalizing lobster? Well, welcome to the world of JavaScript frameworks! From Angular to Vue, there's a smorgasbord of options to choose from. But today, we're putting the spotlight on a particularly delectable dish: Next.js.
What's Next.js?
Next.js is a React-based framework that makes it easy to build server-side rendered and statically generated websites. It's kind of like taking the power of React and supercharging it with some extra features that make you go "wow, I can do that?!"
Server-side Rendering
Server-side rendering (SSR) is a technique where the server generates the HTML for a page on each request. This is the opposite of client-side rendering where all the magic happens in the user's browser. Next.js makes SSR as easy as pie, even easier than baking one if you're kitchen-challenged like me.
Here's a basic example:
import { getServerSideProps } from "next"; export default function Page({ data }) { return <div>{data}</div>; } export async function getServerSideProps() { const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data } }; }
In this snippet, getServerSideProps
is a special function that Next.js calls before rendering the Page component on the server. This function fetches some data from an API and passes it as a prop to the Page component.
Static Site Generation
Next.js also makes it a breeze to generate static sites. This is great for sites where the content doesn't change often. It's like preparing a bunch of sandwiches in advance for a picnic, instead of making them on the spot.
Here's how you can do it:
import { getStaticProps } from "next"; export default function Page({ data }) { return <div>{data}</div>; } export async function getStaticProps() { const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data } }; }
Similar to getServerSideProps
, getStaticProps
is a special function that Next.js calls when generating the static HTML for the Page component. This function fetches some data from an API and passes it as a prop to the Page component.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Next.js?
Next.js is a popular JavaScript framework that simplifies server-side rendering and static site generation. It's based on React, and offers a powerful set of features to build modern, performance-optimized web apps.
What is server-side rendering?
Server-side rendering (SSR) is a technique where the server generates the HTML for a page on each request, unlike client-side rendering where the browser generates the HTML. This can improve the performance of your website, especially for users with slow internet connections.
What is static site generation?
Static site generation is a method where the HTML pages of a website are generated at build time, instead of on each request. This makes the website very fast to load, as the server just has to serve pre-generated HTML files.
How does Next.js simplify server-side rendering and static site generation?
Next.js provides special functions like getServerSideProps
for server-side rendering and getStaticProps
for static site generation. These functions can fetch data and pass it as props to your components, making it easy to generate dynamic HTML on the server or at build time.
Can I use Next.js for client-side rendered apps?
Yes, Next.js supports client-side rendering as well. You can even mix server-side rendering, static site generation, and client-side rendering in the same Next.js app, choosing the best approach for each page.