Implementing Dynamic Routing in Next.js Apps
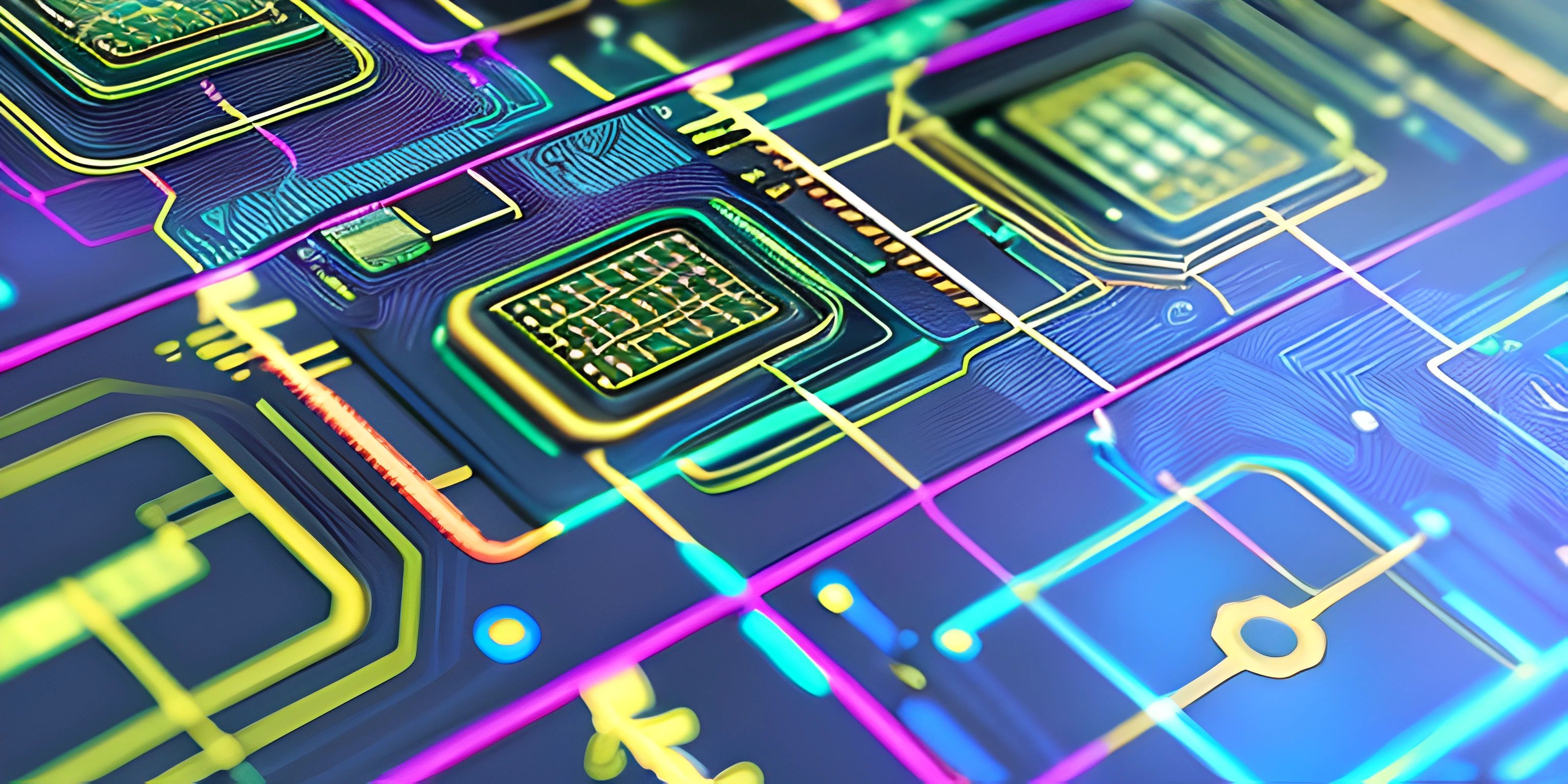
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Next.js is a powerful framework for building React applications that offers a ton of features out of the box. One such feature is dynamic routing, which allows you to create more flexible and scalable navigation systems in your app. Let's dive into how dynamic routing works in Next.js and how to implement it in your projects.
What is Dynamic Routing?
Dynamic routing is a technique that allows a single page to handle multiple different URLs by using placeholders within the route itself. This makes it easy to create pages that can handle a wide range of data without having to create separate pages for each possible case.
In Next.js, dynamic routes are created using the [parameter]
syntax in file or folder names. For example, the file pages/posts/[id].js
would be a dynamic route that could handle URLs like /posts/1
, /posts/42
, and so on.
Setting Up Dynamic Routes in Next.js
To get started with dynamic routing in your Next.js app, follow these steps:
1. Create a Dynamic Route File
First, create a new file in the pages
folder of your Next.js app with the [parameter]
syntax. Let's say we want to create a dynamic route for blog posts, where each post has a unique ID. We would create a file named pages/posts/[id].js
.
2. Access the Route Parameter
In your newly created dynamic route file, you can access the parameter from the URL using the useRouter
hook from next/router
. Here's an example of how to do this:
import { useRouter } from "next/router"; function Post() { const router = useRouter(); const { id } = router.query; return <div>Post ID: {id}</div>; } export default Post;
This code will display the post ID from the URL on the page. The useRouter
hook provides the query
object, which contains the route parameters.
3. Fetch Data Based on the Route Parameter
Once you have access to the route parameter, you can use it to fetch data for your page. Let's update our Post
component to fetch the post data using the getStaticProps
function:
export async function getStaticProps({ params }) { const post = await getPostData(params.id); return { props: { post } }; }
In this example, the getPostData
function would be a custom function that fetches the post data based on the provided ID. The result is then passed as a prop to the Post
component.
4. Specify the Dynamic Route Paths
Next.js needs to know which paths to generate for your dynamic routes. You can do this using the getStaticPaths
function. This function should return an array of possible route parameters for your dynamic route. Here's an example:
export async function getStaticPaths() { const paths = getAllPostIds(); return { paths, fallback: false }; }
In this example, the getAllPostIds
function would return an array of post IDs. The fallback
property determines what happens if a requested path isn't found in the paths
array. If fallback
is false
, Next.js will return a 404 page for non-existent paths.
Wrapping Up
Dynamic routing in Next.js is a powerful feature that can help you build more scalable and flexible navigation systems for your React applications. By leveraging the [parameter]
syntax, the useRouter
hook, and the getStaticProps
and getStaticPaths
functions, you can easily create dynamic pages that handle a wide range of data without creating separate pages for each case. Now you're ready to implement dynamic routing in your own Next.js projects!
FAQ
What is dynamic routing in Next.js?
Dynamic routing in Next.js is a feature that allows you to create pages with flexible, dynamic paths that adapt to various navigation scenarios. It makes it easy to build complex navigation structures without having to hard-code each individual route. By using a special file naming syntax in the pages
directory, Next.js can automatically generate routes based on the file and directory structure.
How do I create a dynamic route in Next.js?
To create a dynamic route in Next.js, you need to use a special file naming syntax in your pages
directory. Use square brackets [
and ]
to enclose the dynamic part of the route. For example, if you want to create a dynamic route for user profiles, you can create a file named profile/[username].js
, and Next.js will automatically generate routes like /profile/johndoe
or /profile/janedoe
. Inside the [username].js
file, you can access the dynamic username
parameter using the router
object from the next/router
module.
How can I access the dynamic parameter value in my Next.js component?
To access the value of the dynamic parameter in your Next.js component, you'll need to use the useRouter
hook from the next/router
module. Here's an example of how to do this:
import { useRouter } from 'next/router'; function UserProfile() { const router = useRouter(); const { username } = router.query; return ( <div> <h1>User Profile: {username}</h1> </div> ); } export default UserProfile;
Can I create nested dynamic routes in Next.js?
Yes, you can create nested dynamic routes in Next.js. To do this, create a directory structure that reflects the desired nested routing structure, and use the special file naming syntax for dynamic parts. For example, to create nested dynamic routes for categories and products, you can create a directory named category
and inside it, create a file named [category]/[product].js
. This will generate routes like /category/electronics/product123
.
How do I generate static pages for dynamic routes using Next.js' static site generation feature?
With Next.js, you can generate static pages for dynamic routes using the getStaticPaths
and getStaticProps
functions. In your dynamic route file, export the getStaticPaths
function to return an array of possible parameter values, and export the getStaticProps
function to fetch the data for each parameter value. Here's an example:
export async function getStaticPaths() { const paths = [ { params: { username: 'johndoe' } }, { params: { username: 'janedoe' } }, ]; return { paths, fallback: false }; } export async function getStaticProps({ params }) { const { username } = params; const userData = await fetchUserData(username); return { props: { userData } }; }