Debugging with Node.js Console Log
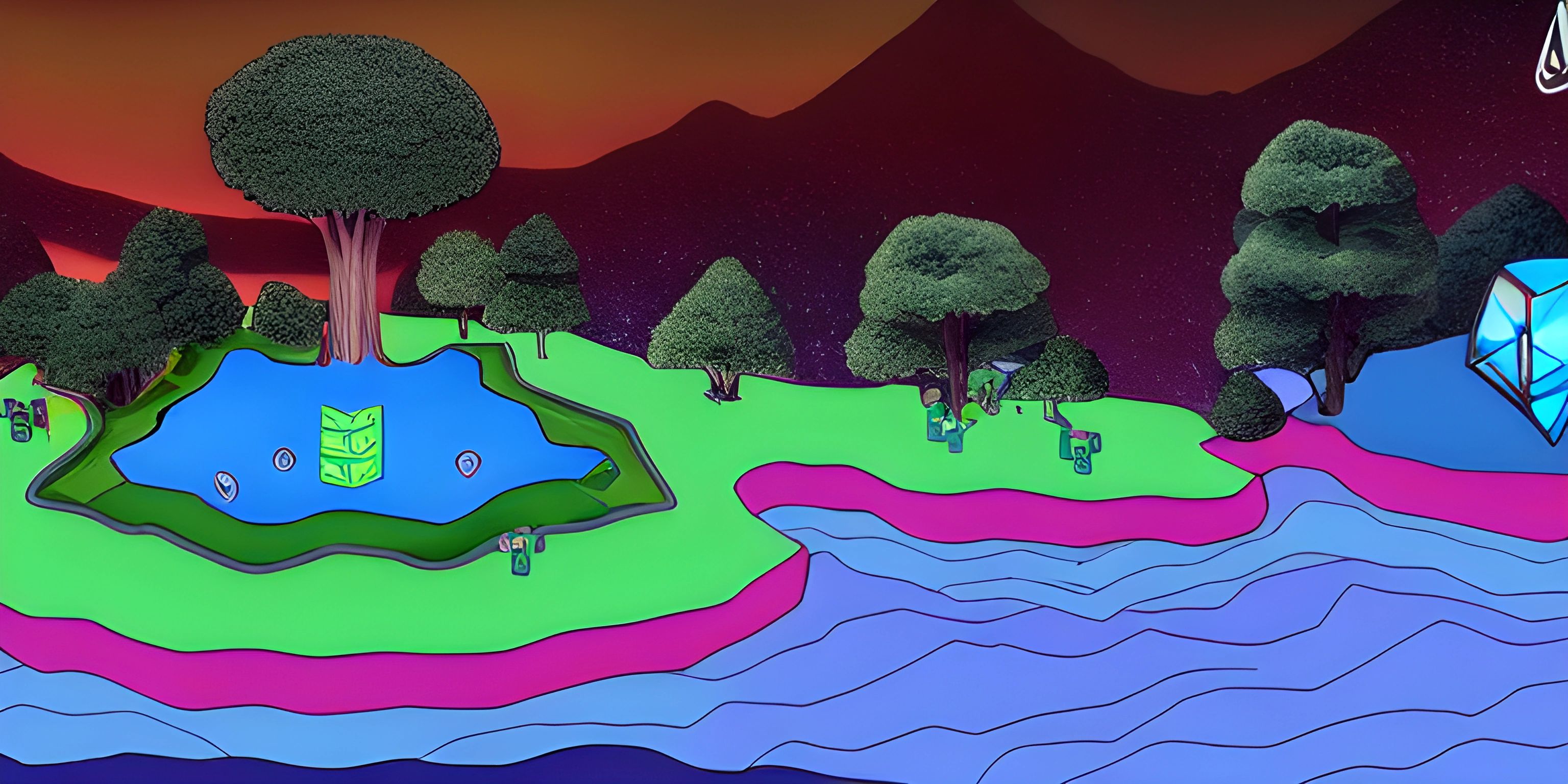
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The journey of a programmer is fraught with peril, and the foes we face are often the bugs within our code. Debugging is the process of finding and resolving these bugs, turning them from monstrous errors into placid lines of code. One of the tools that makes this process a little easier is the humble console.log function used in Node.js.
Console Log - The Debugging Secret Weapon
The console.log function in Node.js is a simple, yet incredibly powerful tool for debugging. It’s like the flashlight in the murky world of coding - it helps you see what’s going on. If you notice that your program isn't behaving as expected, you can use console.log() to print the values of variables, function outputs, or even just a message to help you track the program's flow.
In Node.js, console.log() sends a message to the console, which is typically your terminal or command line. It's like communicating with your future self, leaving a trail of breadcrumbs in the form of console messages.
Let's delve into an example:
var x = 10; console.log("The value of x is: " + x);
In this piece of code, we're first declaring a variable x
and assigning it the value 10. Next, we're using console.log() to print a message to the console. The output of this script would be: The value of x is: 10
.
Debugging with Console Log
Debugging with console.log is as simple as leaving messages for yourself in the code.
Consider this simple function that calculates the area of a rectangle:
function calculateArea(length, width) { return length * width; } console.log(calculateArea(5, 10));
The console will display the output 50
, which is the area of the rectangle with length 5 and width 10. But if you made some changes to the function, and you're not sure why it's not working, you can use console.log inside the function to track down the issue.
For example, if you're getting an unexpected result, you can add a console.log statement to print out the values of length and width:
function calculateArea(length, width) { console.log("Length: " + length + ", Width: " + width); return length * width; } console.log(calculateArea(5, "10"));
This will output:
Length: 5, Width: 10 NaN
This tells us that there's something wrong with our function - it's receiving a string instead of a number for the width, and that's why we're getting NaN
(Not a Number) as a result.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
Why is console.log() useful in debugging?
The console.log() function helps you track the flow of your program and inspect the values of variables and outputs of functions at various points in time. It can be particularly helpful in identifying where and why your program is behaving unexpectedly.
How can I use console.log() in my Node.js application?
To use console.log(), simply call the function with the message or variable you want to print as an argument, like so: console.log("This is a console message"). The message will be printed to the console when the line of code is executed.
What does the output NaN mean?
NaN stands for 'Not a Number'. It's a special value that indicates a missing or undefined numerical result. For example, if you try to perform a mathematical operation on a string, the result will be NaN.