Java Methods for Beginners
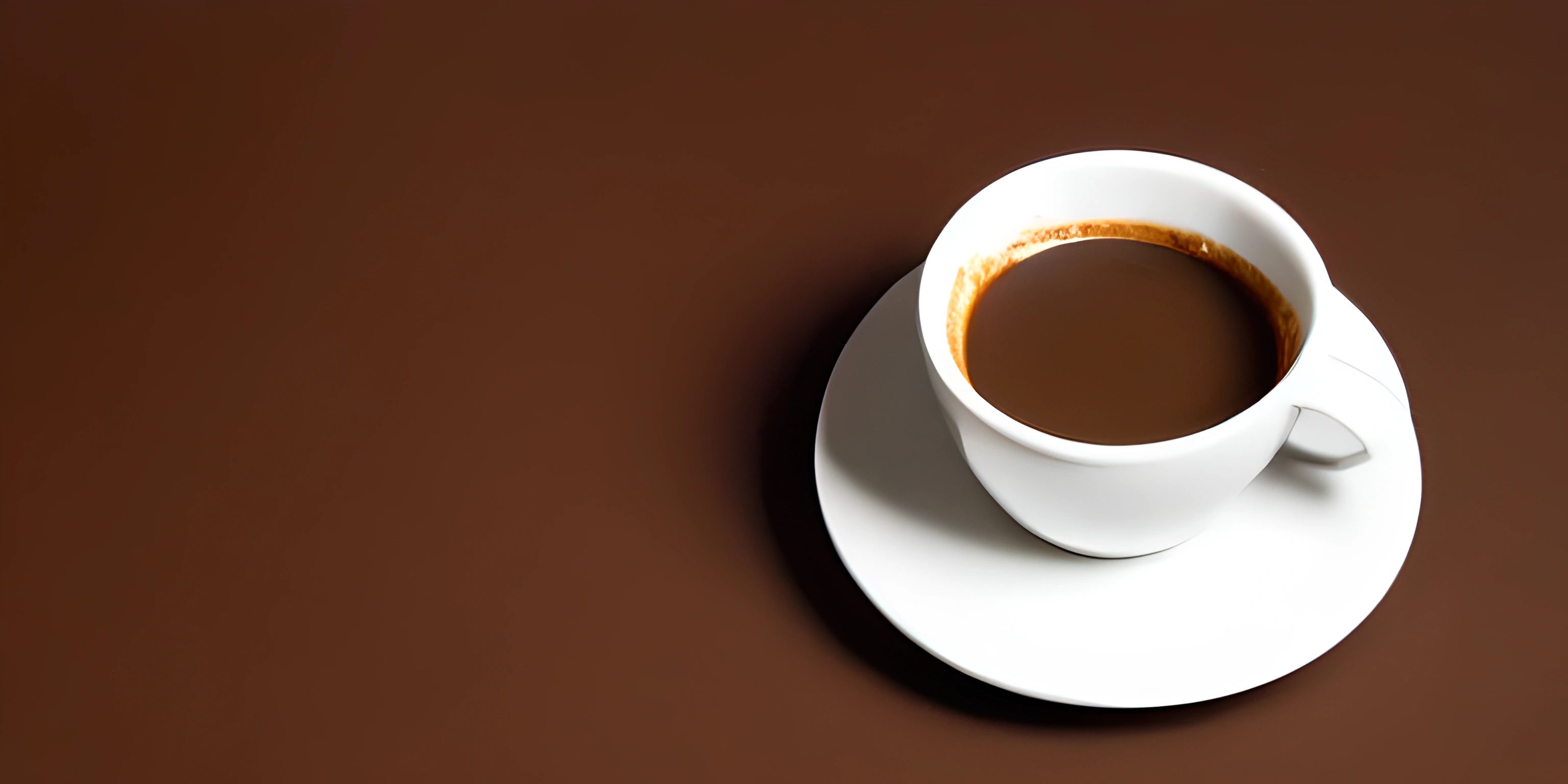
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Starting your journey with Java programming? You'll quickly come across a concept called methods. They are essential building blocks of Java programs, allowing you to organize and reuse code. In this beginner-friendly guide, we'll explore Java methods, how to create and use them, and why they're beneficial.
What are Methods?
Methods, also called functions in some programming languages, are groups of code statements designed to perform a specific task. Methods help you break down a complex program into smaller, manageable pieces. They improve code readability, reduce redundancy, and make your program easier to maintain.
Think of methods like recipes. Each recipe consists of a series of steps that lead to a delicious outcome. In the same way, methods contain code statements that work together to achieve a particular result.
Declaring a Java Method
In Java, you declare a method within a class. The basic syntax for declaring a method is:
accessModifier returnType methodName(parameters) { // method body }
- accessModifier: Determines the visibility of the method (e.g.,
public
,private
, orprotected
). - returnType: Indicates the data type of the value the method returns (e.g.,
int
,String
, etc.). If the method doesn't return a value, use the keywordvoid
. - methodName: The name you give to the method. It should be descriptive and follow the camelCase naming convention.
- parameters: A list of input values the method takes, enclosed in parentheses. Parameters allow you to pass data to the method when you call it.
Here's an example of a simple Java method:
public void greet() { System.out.println("Hello, world!"); }
This method, named greet
, prints "Hello, world!" to the console. It doesn't take any parameters and doesn't return a value.
Calling a Java Method
To use a method, you need to call or invoke it. To call a method, write its name followed by parentheses ()
and a semicolon ;
. If the method takes parameters, include the arguments within the parentheses.
Let's call the greet
method from the previous example:
public static void main(String[] args) { // Create an instance of the class containing the method MyClass obj = new MyClass(); // Call the greet method obj.greet(); }
When you run this code, the output will be:
Hello, world!
Working with Parameters and Return Values
Methods can take input values (parameters) and return a value. Let's create a method that adds two integers and returns the result:
public int add(int a, int b) { // Add the two numbers and store the result int sum = a + b; // Return the result return sum; }
Now, let's call the add
method and print the result:
public static void main(String[] args) { MyClass obj = new MyClass(); // Call the add method with two integers as arguments int result = obj.add(3, 5); // Print the result System.out.println("The sum is: " + result); }
The output will be:
The sum is: 8
Conclusion
Java methods are crucial for organizing and reusing code. They help you break down complex tasks into smaller, more manageable pieces. With this beginner's guide, you now have a solid understanding of how to create and use methods in Java. Keep practicing, and soon you'll be writing efficient, reusable code like a pro!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is a Java method and why is it important for beginners to learn about them?
A Java method is a block of code that performs a specific task, and can be called by other parts of the program. Learning about methods is crucial for Java beginners because they help organize and structure the code, making it more readable and reusable. Understanding methods allows you to write efficient and modular code, which is essential in real-world applications.
How do I create a Java method?
To create a Java method, you need to define it within a class. The syntax for creating a method is as follows:
modifier returnType methodName(parameters) { // method body }
The modifier determines the access level of the method, returnType specifies the type of value the method returns, methodName is the name you give the method, and parameters are the input values the method accepts. For example, here's a simple method that adds two integers:
public int addNumbers(int a, int b) { int sum = a + b; return sum; }
How do I call a Java method in my program?
To call a Java method, you need to use the method name followed by parentheses, with any required arguments inside. If the method is defined within the same class, you can call it directly. If the method is in a different class, you need to create an instance of the class first (unless the method is static). Here's an example of calling the addNumbers
method from a main
method in the same class:
public static void main(String[] args) { // Create an instance of the class MyClass myInstance = new MyClass(); // Call the addNumbers method int result = myInstance.addNumbers(3, 4); System.out.println("The sum is: " + result); }
Can Java methods return different data types?
Yes, Java methods can return different data types, including primitive types (like int
, double
, char
, etc.), reference types (like objects and arrays), or even no value at all (using the void
keyword). When defining a method, you must specify the return type, and the method must return a value of that type.
What is method overloading in Java, and how can I use it?
Method overloading in Java allows you to define multiple methods with the same name but different parameters. This can make your code more flexible and readable. To use method overloading, simply create multiple methods with the same name but different parameter lists:
public int multiply(int a, int b) { return a * b; } public double multiply(double a, double b) { return a * b; }
In this example, there are two multiply
methods with different parameter types (int and double). When calling the method, Java will automatically select the appropriate version based on the arguments provided.