Console Log in JavaScript: Your Debugging Friend
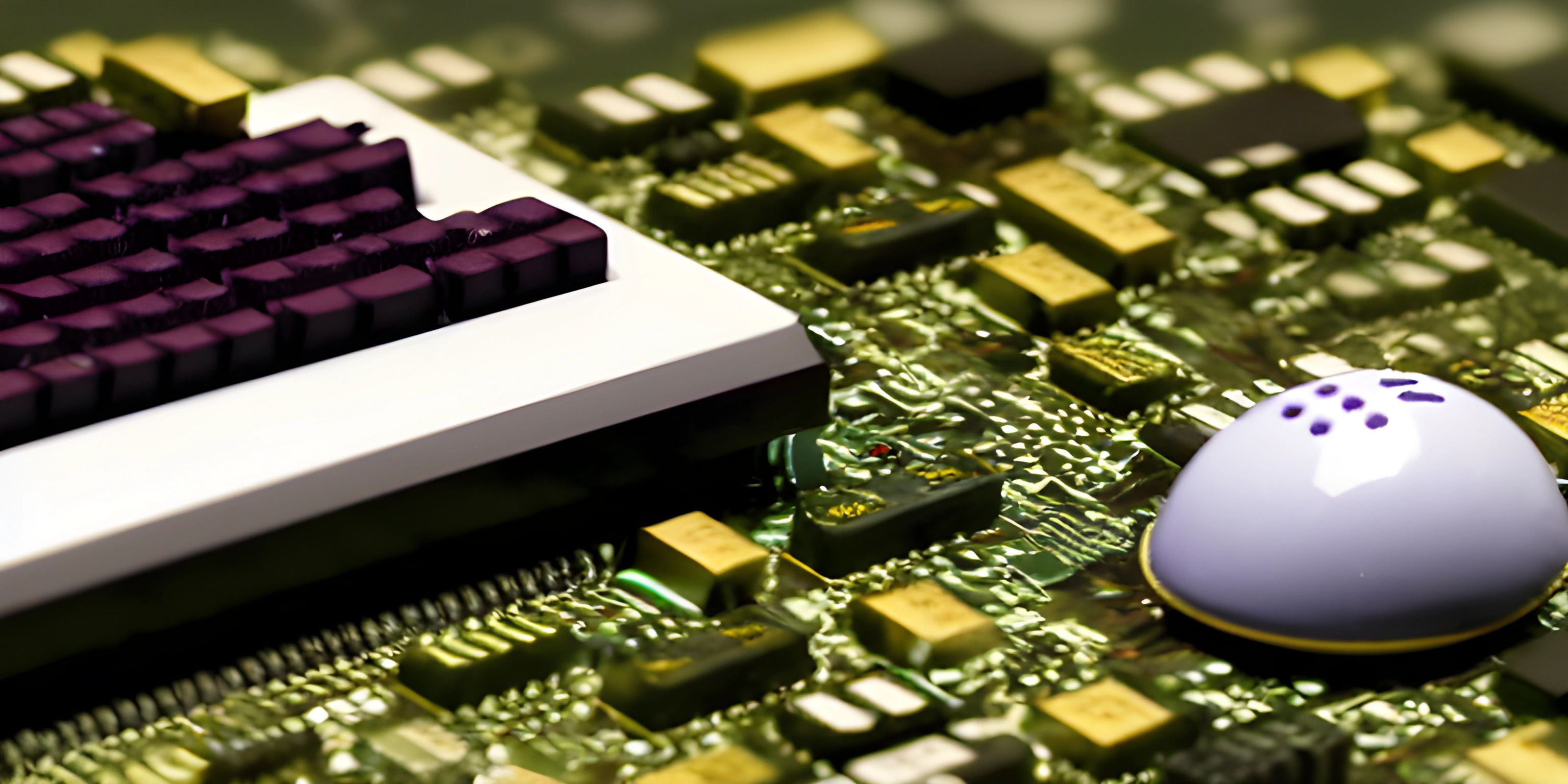
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Console log, the unsung hero of JavaScript, has saved more developer hours than all the energy drinks combined. This diligent function has a simple job but plays a critical role in your coding journey.
Console Log: An Overview
The console.log
function in JavaScript is a debugger's best friend. It allows you to print messages, variables, objects, or any other output to the console. It's like having a secret conversation with your program, where it spills the beans about what it's up to.
It's important to know that the console we're referring to here is not the DOS-like black screen you might be imagining. Instead, it's part of the web browser’s developer tools that you can easily access by right-clicking on a webpage and selecting "Inspect" or "Inspect Element".
How to Use Console Log
The syntax of console.log
is straightforward. Here's a simple usage:
console.log("Hi, I am a message.");
When this line runs, it prints: "Hi, I am a message." to the console. Simple, isn't it? It's like playing Marco Polo with your code. You shout console.log
, and the function echoes back with whatever message you sent.
But wait, there's more! console.log
can also print out variables, arrays, objects, and more. Let's say you have a variable x
that you've set to 10 and you want to make sure it's holding the right value. You can use console.log
like this:
let x = 10; console.log(x);
Your console will merrily print out 10
, confirming that x
is indeed holding the right value.
When to Use Console Log
The console.log
function is most commonly used for debugging purposes. It's like turning on the light in a dark room to find out what's bumping there. It can help you find errors in your code, understand the flow of your program, or check the values of variables at various stages.
Remember, though, that console.log
is not intended to be part of your final, polished code. It's more like scaffolding that helps you during the construction phase but should be removed once the building is complete.
So, next time you're lost in your code, remember that console.log
is there, ready to lend a hand. It might not be flashy, but it's dependable. And in the coding world, that's gold.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Advanced Data Types (psst, it's free!).
FAQ
What is the `console.log` function in JavaScript?
The console.log
function in JavaScript allows you to print messages, variables, objects, or any other output to the console. It is predominantly used for debugging purposes.
How do I use the `console.log` function?
You use it by typing console.log
followed by parentheses containing the message or variable you want to print out. For example, console.log("Hello, World!")
will print "Hello, World!" to the console.
When should I use `console.log`?
You should use console.log
when you're trying to debug your code. It can help you understand the flow of your program, check the values of variables, or spot errors. However, it's not meant to be part of your final code - it's for development use only.