Exploring Conditional Statements, Loops, and Functions
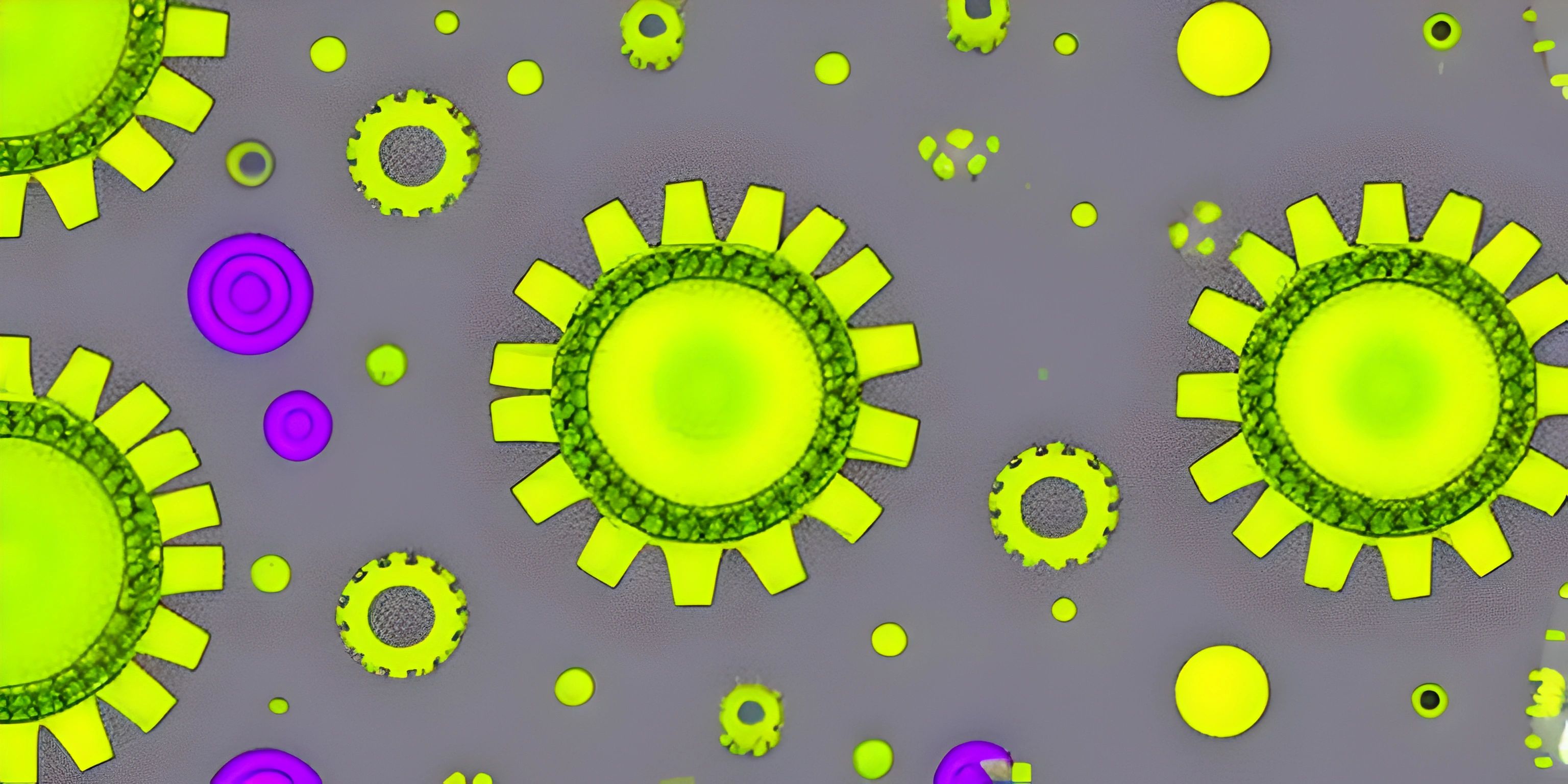
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In programming, logic and algorithms are the foundation of any code. They control the flow of a program and determine how it processes data. In this article, we will explore conditional statements, loops, and functions, which are essential concepts in programming logic and algorithms.
Conditional Statements
Conditional statements are used to make decisions in code based on whether a specific condition is true or false. The most common conditional statements are if
, else
, and else if
. Here's a basic example in JavaScript:
let age = 18; if (age >= 18) { console.log("You are an adult."); } else { console.log("You are a minor."); }
In this example, the if
statement checks if the age
variable is greater than or equal to 18. If the condition is true, it will print "You are an adult." If the condition is false, the else
statement will execute, and it will print "You are a minor."
Loops
Loops are used to repeat a specific block of code until a certain condition is met. The most common types of loops are for
, while
, and do-while
. Here's an example of a for
loop in JavaScript:
for (let i = 0; i < 5; i++) { console.log("Iteration", i); }
In this example, the for
loop initializes a variable i
with a value of 0. It then checks if i
is less than 5. If the condition is true, it will execute the block of code inside the loop and increment i
by 1. This process will repeat until the condition is false (i.e., i
is no longer less than 5).
Functions
Functions are reusable blocks of code that perform a specific task. They can be defined with parameters and can return a value. Functions help to break down complex tasks into smaller, more manageable pieces of code. Here's an example of a function in JavaScript:
function sum(a, b) { return a + b; } let result = sum(3, 4); console.log(result); // Output: 7
In this example, we define a function called sum
that takes two parameters, a
and b
. The function returns the sum of the two parameters. We then call the function with the values 3 and 4 and store the result in the variable result
.
Understanding conditional statements, loops, and functions is crucial for writing efficient and effective code. Mastering these concepts will help you tackle more complex programming challenges and develop a strong foundation in programming logic and algorithms.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What are the main concepts covered in this article about programming logic and algorithms?
This article covers the fundamentals of programming logic and algorithms, with a focus on conditional statements, loops, and functions. These concepts are essential for understanding how to control the flow of a program and perform various tasks efficiently.
What are conditional statements and why are they important in programming?
Conditional statements are used to make decisions in a program based on whether a certain condition is met. They allow the program to execute different blocks of code depending on the outcome of a test expression. This feature is crucial in programming as it enables the creation of more complex and dynamic applications that can adapt to different situations.
How do loops help in making repetitive tasks easier in programming?
Loops are used to repeatedly execute a block of code as long as a specified condition is true. They simplify repetitive tasks by reducing the amount of code required and making the program more efficient. Loops are especially useful when working with data structures, such as arrays or lists, where you might need to perform the same operation on multiple elements.
Can you provide a brief overview of functions and their role in programming logic?
Functions are reusable blocks of code that can be called with specific inputs (arguments) and return a value after performing a specific task. Functions promote modularity and reusability in programming, making the code more organized and easier to maintain. They also help to break down complex problems into smaller, manageable pieces, allowing programmers to focus on individual aspects of the problem.
What are some common programming languages that utilize conditional statements, loops, and functions?
Most programming languages, including popular ones like Python, JavaScript, Java, C++, and Ruby, support the use of conditional statements, loops, and functions. These concepts are fundamental to programming and form the basis for creating efficient and versatile programs across various languages and platforms.