Getting Started with C++ Programming
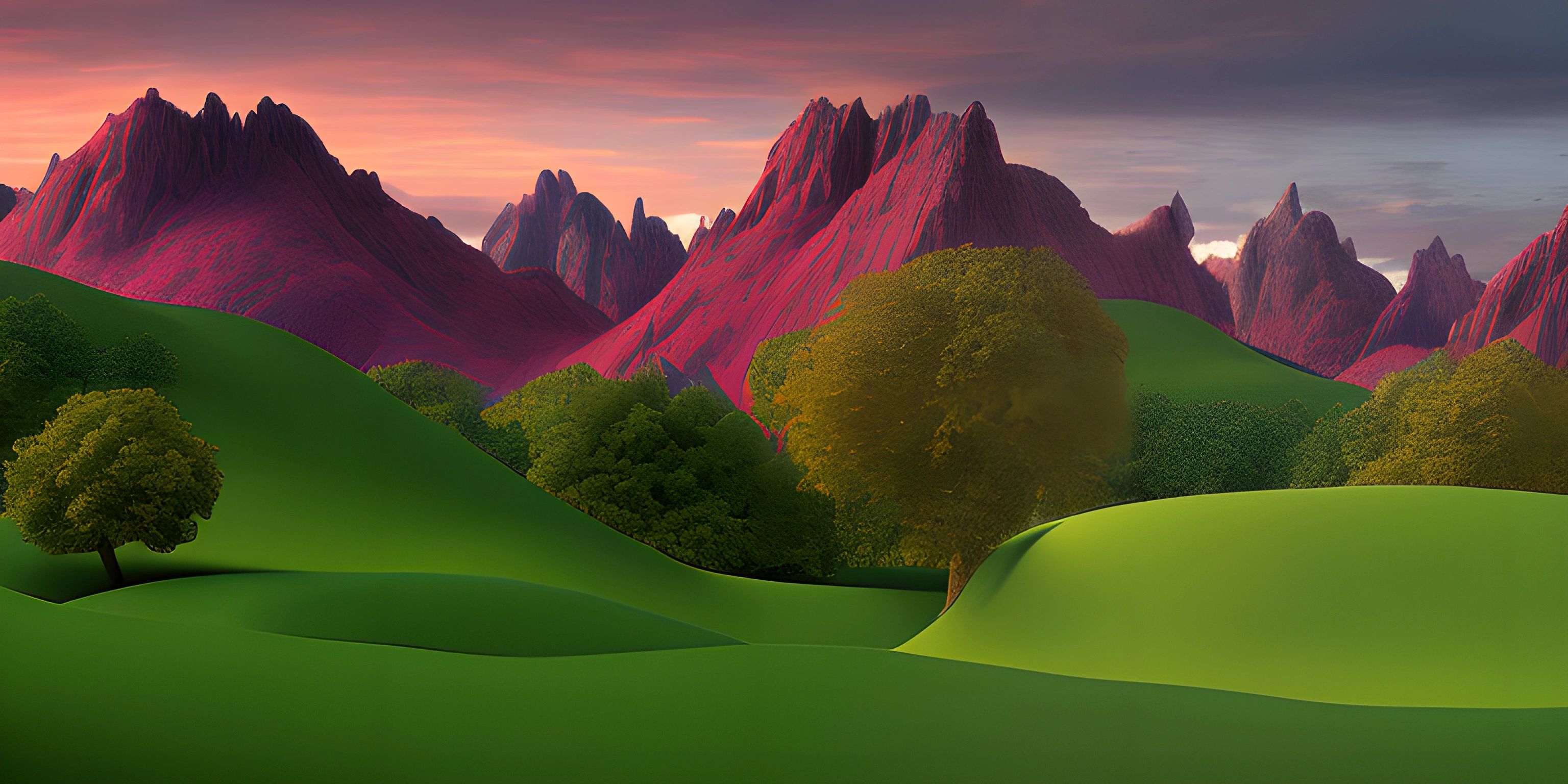
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
C++ is a powerful, versatile, and widely-used programming language that has been around since the 1980s. With applications ranging from systems programming to game development, it's no surprise that C++ has stood the test of time. Let's dive into the basics and get you started with C++ programming!
Setting Up Your Environment
To begin, you'll need a compiler and an Integrated Development Environment (IDE) to write, compile, and run your C++ code. Some popular choices include Visual Studio, Code::Blocks, and CLion. Pick an IDE, install it, and set up a new project to start writing C++ code.
Hello, World! in C++
Let's begin with the classic "Hello, World!" program. Here's what it looks like in C++:
#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
This simple program demonstrates some core C++ concepts, such as #include
for importing libraries, the main
function, and the std::cout
object for printing to the console.
Variables and Types
C++ is a statically-typed language, which means that you must declare the type of a variable before using it. The most commonly used types include int
for integers, float
for floating-point numbers, double
for double-precision floating-point numbers, and char
for characters.
Here's an example of declaring and initializing variables in C++:
int age = 25; float height = 1.75f; double pi = 3.141592653589793; char initial = 'A';
Functions
Functions are reusable blocks of code that perform a specific task. A function declaration includes a return type, a function name, and an optional list of parameters. The main
function is the entry point of any C++ program, and its return type is int
.
Here's an example of a simple function that adds two integers:
int add(int a, int b) { return a + b; } int main() { int result = add(5, 10); std::cout << "The sum is: " << result << std::endl; return 0; }
Control Structures
Control structures are used to direct the flow of execution in a program. The most common control structures are if
, for
, and while
.
Here's an example using if
, for
, and while
in C++:
int main() { int age = 18; if (age >= 18) { std::cout << "You are an adult" << std::endl; } else { std::cout << "You are not an adult" << std::endl; } for (int i = 0; i < 5; ++i) { std::cout << "Loop iteration: " << i << std::endl; } int count = 0; while (count < 5) { std::cout << "Count: " << count << std::endl; count++; } return 0; }
These are just the basics of C++ programming. As you progress, you'll learn about more advanced topics like pointers, classes, and templates.
FAQ
What is C++ used for?
C++ is a versatile programming language used in various domains, such as systems programming, game development, embedded systems, and high-performance computing.
What are some popular C++ IDEs?
Some popular C++ Integrated Development Environments (IDEs) are Visual Studio, Code::Blocks, and CLion.
How do I declare and initialize a variable in C++?
To declare and initialize a variable in C++, specify its type, followed by its name and an assignment operator with its initial value. For example: int age = 25;
.
What are the basic control structures in C++?
The most common control structures in C++ are if
, for
, and while
. These are used to manage the flow of execution in a program based on conditions and loops.