Inheritance in C++
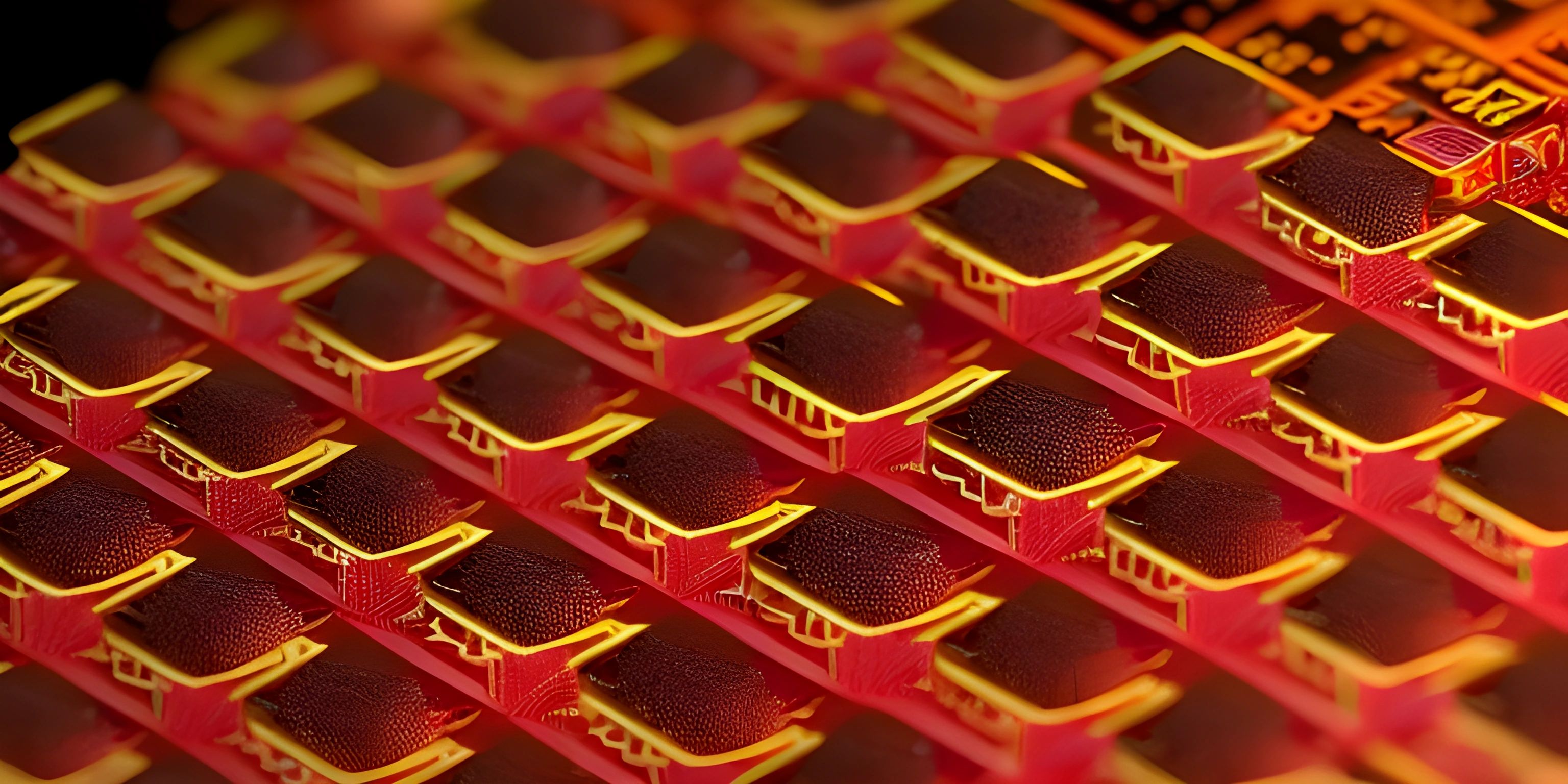
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Inheritance is a key concept in object-oriented programming, and C++ is no exception. It allows you to create new classes that inherit properties and behaviors from existing classes, promoting code reusability and a clean, organized structure. In this article, you'll learn about the basics of inheritance in C++ and how it can be used to create more powerful and efficient programs.
What is Inheritance?
Inheritance is a way to create a new class, called the derived class, by inheriting the properties and behaviors of an existing class, called the base class. This relationship enables the derived class to reuse and extend the functionalities of the base class, creating a hierarchy of classes that share common features.
Syntax
To inherit from a base class in C++, you'll use the colon (:
) followed by an access specifier (public
, protected
, or private
) and the name of the base class. Here's a simple example:
class BaseClass { public: void baseFunction() { // Code for baseFunction } }; class DerivedClass : public BaseClass { public: void derivedFunction() { // Code for derivedFunction } };
In this example, DerivedClass
inherits from BaseClass
. Because the inheritance is specified as public
, all public members of BaseClass
will be accessible in DerivedClass
.
Benefits of Inheritance
- Code Reusability: Inheritance promotes reusing code by allowing derived classes to inherit properties and behaviors from base classes.
- Modularity: It helps in organizing code into a hierarchy of classes, making it easier to maintain and understand.
- Polymorphism: Inheritance enables polymorphism, which allows objects of different classes to be treated as objects of a common base class, increasing flexibility in program design.
Types of Inheritance
C++ supports several types of inheritance, including:
- Single Inheritance: A derived class inherits from a single base class.
- Multilevel Inheritance: A derived class inherits from a base class, which is itself derived from another class, creating a chain of inheritance.
- Multiple Inheritance: A derived class inherits from more than one base class.
- Hierarchical Inheritance: Multiple derived classes inherit from a single base class.
- Hybrid Inheritance: A combination of two or more forms of inheritance.
Access Specifiers and Inheritance
When inheriting from a base class, the access specifier used (public
, protected
, or private
) determines how the base class members are accessible in the derived class:
public
: Base class members keep their original access in the derived class.protected
: Base class public members become protected in the derived class.private
: Base class public and protected members become private in the derived class.
Note that private members of the base class are never directly accessible in the derived class, regardless of the access specifier used.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is inheritance in C++?
Inheritance in C++ is a key concept in object-oriented programming that allows you to create new classes (derived classes) by inheriting properties and behaviors from existing classes (base classes). This promotes code reusability and a clean, organized structure.
What are the benefits of inheritance in C++?
Inheritance in C++ promotes code reusability, modularity, and enables polymorphism. It allows derived classes to inherit properties and behaviors from base classes, organizing code into a hierarchy for easier maintenance and understanding, and supports polymorphism for increased flexibility in program design.
What are the different types of inheritance in C++?
C++ supports several types of inheritance, including single inheritance, multilevel inheritance, multiple inheritance, hierarchical inheritance, and hybrid inheritance.
How do access specifiers affect inheritance in C++?
When inheriting from a base class, the access specifier (public, protected, or private) determines how the base class members are accessible in the derived class. Public members keep their original access, protected members become protected or private, and private members are never directly accessible in the derived class.