Perlin Noise Basics
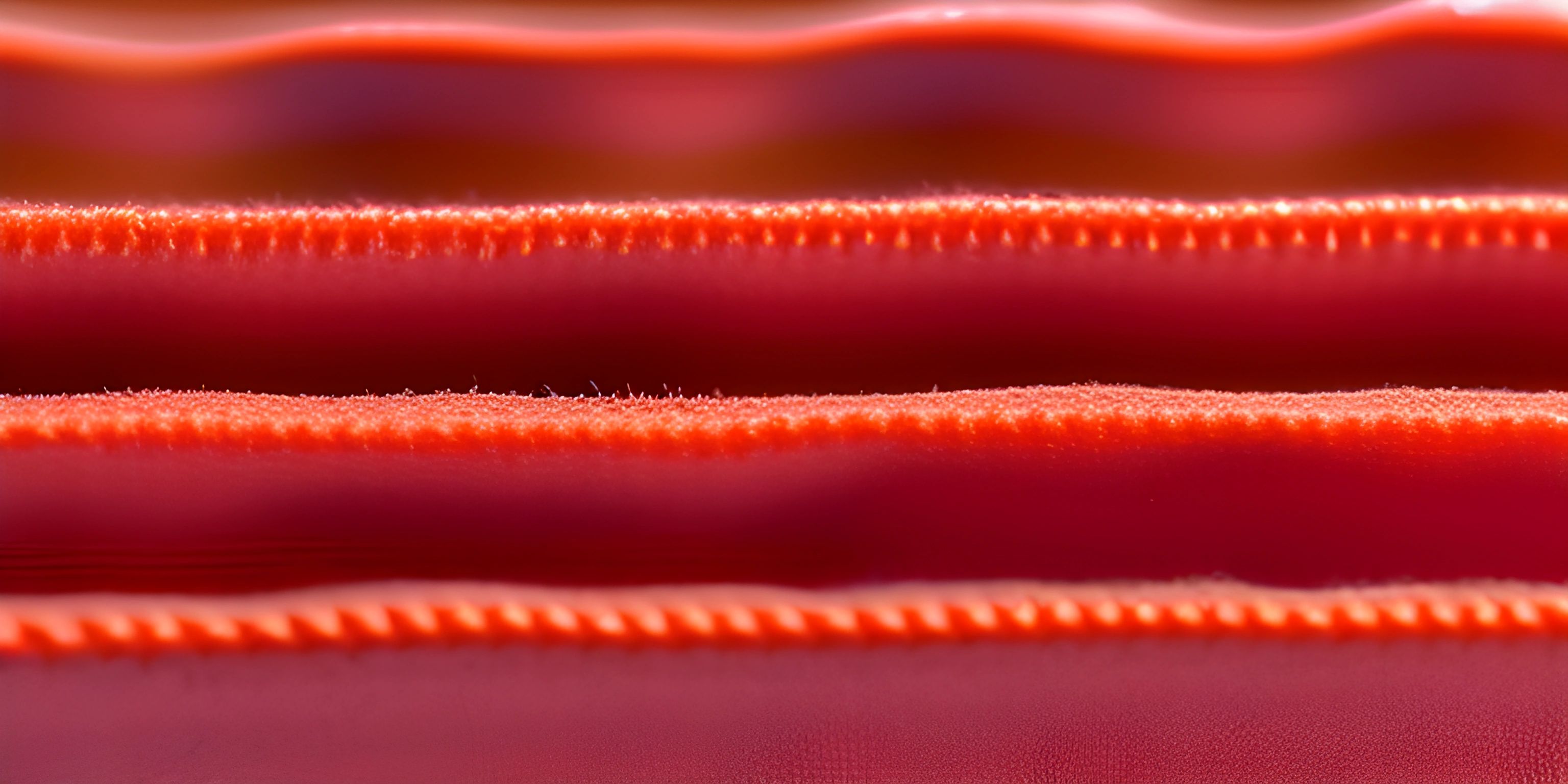
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine a world where landscapes flow seamlessly, clouds drift lazily across the sky, and textures blend together harmoniously. This isn't the whimsical daydream of an over-caffeinated artist; it's the magic of Perlin noise! Named after Ken Perlin, who invented it in 1983, Perlin noise is a type of gradient noise often used for procedural generation, particularly in the fields of computer graphics and game development.
What is Perlin Noise?
At its core, Perlin noise is a gradient noise function that generates smooth, continuous random values. Unlike simple random noise, which can be jarring and abrupt, Perlin noise produces a more natural, organic appearance. It's like the difference between the harsh buzz of static on a TV and the gentle rustle of leaves in the wind.
How Perlin Noise Works
Perlin noise creates a grid of gradient vectors. Each point on the grid is assigned a random gradient vector, which influences the noise values around it. The final noise value for any point in space is determined by interpolating between these gradients.
Here's an analogy: imagine you're standing on a grassy hill. Each blade of grass represents a gradient vector. When you walk across the hill, the height of the grass changes smoothly, without any sudden jumps or gaps. This smooth transition is the essence of Perlin noise.
Generating Perlin Noise
Let's dive into an example of generating Perlin noise in Python. We'll use the noise
library, which provides a simple interface for creating Perlin noise.
First, install the noise
library if you haven't already:
pip install noise
Now, let's generate a simple 2D Perlin noise map:
import numpy as np import matplotlib.pyplot as plt from noise import pnoise2 # Define the dimensions of the noise map width, height = 100, 100 # Create an empty array to store the noise values noise_map = np.zeros((width, height)) # Generate the noise values for i in range(width): for j in range(height): noise_map[i][j] = pnoise2(i / 10, j / 10, octaves=6, persistence=0.5, lacunarity=2.0, repeatx=width, repeaty=height, base=42) # Plot the noise map plt.imshow(noise_map, cmap='gray') plt.colorbar() plt.show()
In this example, we use the pnoise2
function to generate 2D Perlin noise. The parameters adjust the characteristics of the noise:
octaves
: The number of levels of detail.persistence
: Controls the amplitude of each octave.lacunarity
: Controls the frequency of each octave.repeatx
andrepeaty
: Ensure the noise wraps around, making it tileable.base
: The seed for the random number generator.
Applications of Perlin Noise
Perlin noise is a versatile tool with many applications, particularly in the realms of computer graphics and procedural generation.
Terrain Generation
One of the most common uses of Perlin noise is generating realistic terrain in video games and simulations. By combining multiple layers of Perlin noise, developers can create mountains, valleys, and other natural features that blend smoothly together.
import numpy as np import matplotlib.pyplot as plt from noise import pnoise2 def generate_terrain(width, height, scale=100.0): terrain = np.zeros((width, height)) for i in range(width): for j in range(height): terrain[i][j] = pnoise2(i / scale, j / scale, octaves=6, persistence=0.5, lacunarity=2.0, repeatx=width, repeaty=height, base=42) return terrain terrain = generate_terrain(200, 200, scale=50.0) plt.imshow(terrain, cmap='terrain') plt.colorbar() plt.show()
Texture Generation
Perlin noise is also used to create natural-looking textures for materials like wood, marble, and clouds. By manipulating the noise parameters and combining different noise layers, artists can achieve a wide range of visual effects.
import numpy as np import matplotlib.pyplot as plt from noise import pnoise2 def generate_texture(width, height, scale=100.0): texture = np.zeros((width, height)) for i in range(width): for j in range(height): texture[i][j] = pnoise2(i / scale, j / scale, octaves=4, persistence=0.6, lacunarity=2.0, repeatx=width, repeaty=height, base=24) return texture texture = generate_texture(200, 200, scale=25.0) plt.imshow(texture, cmap='plasma') plt.colorbar() plt.show()
Animation and Special Effects
In addition to static images, Perlin noise can be used to create dynamic effects like flowing water, billowing smoke, and flickering flames. By animating the noise over time, you can achieve realistic, fluid movement.
import numpy as np import matplotlib.pyplot as plt from noise import pnoise3 import matplotlib.animation as animation # Define the dimensions of the noise map width, height = 100, 100 frames = 60 # Create an empty array to store the noise values noise_map = np.zeros((width, height, frames)) # Generate the noise values for i in range(width): for j in range(height): for k in range(frames): noise_map[i][j][k] = pnoise3(i / 20, j / 20, k / 10, octaves=4, persistence=0.5, lacunarity=2.0, repeatx=width, repeaty=height, repeatz=frames, base=42) # Create an animation fig = plt.figure() im = plt.imshow(noise_map[:, :, 0], cmap='inferno', animated=True) def updatefig(frame): im.set_array(noise_map[:, :, frame]) return [im] ani = animation.FuncAnimation(fig, updatefig, frames=frames, interval=50, blit=True) plt.show()
Conclusion
Perlin noise is an essential tool for anyone interested in procedural generation and computer graphics. Its ability to create smooth, natural-looking randomness makes it ideal for terrain, textures, and animations. Whether you're designing a video game world or creating stunning visual effects, understanding the basics of Perlin noise will open up a world of creative possibilities.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is the difference between Perlin noise and simple random noise?
Perlin noise generates smooth, continuous random values, while simple random noise can be abrupt and jarring. Perlin noise produces a more natural and organic appearance, making it ideal for procedural generation and computer graphics.
What are some common applications of Perlin noise?
Perlin noise is commonly used in terrain generation, texture creation, and animation. It helps create realistic landscapes, natural-looking textures, and dynamic special effects.
How do you generate Perlin noise in Python?
You can use the noise
library in Python to generate Perlin noise. Functions like pnoise2
and pnoise3
can create 2D and 3D Perlin noise, respectively, with various parameters to control the characteristics of the noise.
Can Perlin noise be used for real-time applications?
Yes, Perlin noise can be used for real-time applications such as game development and animations. By adjusting the parameters and optimizing the noise generation process, you can achieve real-time performance.
What are the key parameters for generating Perlin noise?
The key parameters for generating Perlin noise include octaves
, persistence
, lacunarity
, and base
. Octaves
control the levels of detail, persistence
affects the amplitude, lacunarity
influences the frequency, and base
sets the seed for the random number generator.