Perlin Noise Explained
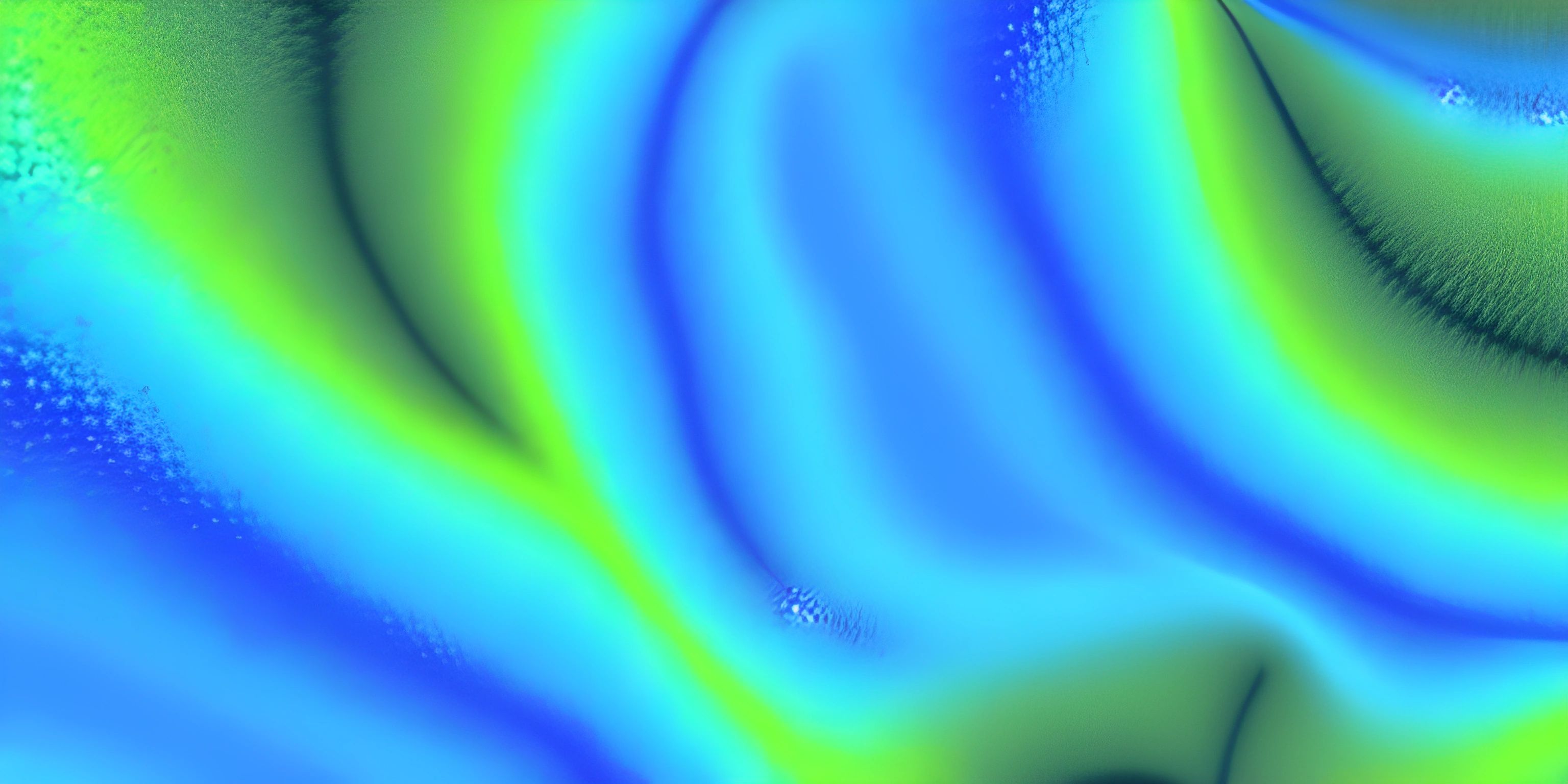
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Perlin Noise! It sounds like the title of a sci-fi novel, but it’s actually a mathematical concept that breathes life into computer graphics and generative art. If you’ve ever admired the beauty of procedurally generated landscapes in video games or the mesmerizing patterns in digital art, chances are you’ve encountered Perlin Noise. Created by Ken Perlin in 1983, this algorithm has become an essential tool in the toolbox of artists and developers alike.
What is Perlin Noise?
Imagine you’re an artist, but instead of a brush, you have an infinite canvas and a mathematical formula. Perlin Noise is that formula. It’s a type of gradient noise that produces a more natural, coherent structure compared to simple random noise. Think of it as the difference between the randomness of TV static and the flowing, organic patterns of rolling hills.
The Mathematics Behind It
Perlin Noise can be a bit tricky to wrap your head around at first, but let’s break it down step by step. The core idea is to create smooth transitions between random values. This is achieved through a combination of interpolation and gradient vectors.
- Grid and Gradients: Imagine a grid overlaying your canvas. At each grid intersection (or lattice point), assign a random gradient vector. This vector will influence the noise value at nearby points.
- Dot Product: For each point on the canvas, compute the dot product between the gradient vectors and the distance vector from the point to the lattice points.
- Interpolation: Interpolate the results to get the final noise value. This smooth blending is what gives Perlin Noise its characteristic look.
Here’s a simplified example in Python to help visualize the process:
import numpy as np def fade(t): # Fade function as defined by Ken Perlin. This eases coordinate values # so that they will "ease" towards integral values. return t * t * t * (t * (t * 6 - 15) + 10) def lerp(a, b, x): # Linear interpolation return a + x * (b - a) def grad(hash, x, y): # Convert lower 4 bits of hash into 12 gradient directions h = hash & 15 u = x if h < 8 else y v = y if h < 4 else x if h in (12, 14) else 0 return (u if h & 1 == 0 else -u) + (v if h & 2 == 0 else -v) def perlin(x, y): # Determine grid cell coordinates X = int(x) & 255 Y = int(y) & 255 # Relative x and y coordinates within grid cell x = x - int(x) y = y - int(y) # Compute fade curves for x and y u = fade(x) v = fade(y) # Hash coordinates of the 4 square corners n00 = grad(permutation[X + permutation[Y]], x, y) n01 = grad(permutation[X + permutation[Y + 1]], x, y - 1) n10 = grad(permutation[X + 1 + permutation[Y]], x - 1, y) n11 = grad(permutation[X + 1 + permutation[Y + 1]], x - 1, y - 1) # Add blended results from 4 corners of square return lerp(lerp(n00, n10, u), lerp(n01, n11, u), v) permutation = np.arange(256) np.random.shuffle(permutation) permutation = np.stack([permutation, permutation]).flatten() # Generate a 2D grid of Perlin noise values width, height = 5, 5 noise_grid = np.zeros((width, height)) for i in range(width): for j in range(height): noise_grid[i, j] = perlin(i / width, j / height) print(noise_grid)
Applications in Generative Art
Perlin Noise isn’t just a mathematical curiosity; it’s a cornerstone of generative art. Its smooth, natural transitions make it perfect for creating organic patterns and textures.
Landscapes and Terrain Generation
One of the most common uses of Perlin Noise is in generating realistic landscapes. By applying Perlin Noise at different scales and combining the results (a process known as fractal noise), you can create detailed and varied terrains.
import matplotlib.pyplot as plt def generate_terrain(width, height, scale): terrain = np.zeros((width, height)) for i in range(width): for j in range(height): terrain[i, j] = perlin(i / scale, j / scale) return terrain terrain = generate_terrain(100, 100, 10) plt.imshow(terrain, cmap='terrain') plt.colorbar() plt.show()
Texture Generation
Perlin Noise can also be used to generate textures for materials like wood, marble, and clouds. By tweaking the parameters and combining multiple layers of noise, you can simulate a wide range of natural textures.
def generate_wood_texture(width, height, scale): texture = np.zeros((width, height)) for i in range(width): for j in range(height): x = i / width * scale y = j / height * scale texture[i, j] = np.sin(x + perlin(x, y) * scale) return texture wood_texture = generate_wood_texture(100, 100, 5) plt.imshow(wood_texture, cmap='gray') plt.show()
Beyond Art: Procedural Generation
Perlin Noise isn’t just for artists; it’s also a powerful tool in the world of procedural generation. From the dungeons of “Minecraft” to the star systems of “No Man’s Sky,” Perlin Noise helps create immersive, endless worlds.
Game Development
In game development, Perlin Noise is used to create terrains, weather patterns, and even sound effects. Its ability to generate coherent, natural-looking data makes it invaluable for creating immersive environments.
Animation and Visual Effects
Perlin Noise is also a staple in animation and visual effects. It can be used to create realistic smoke, fire, and other dynamic effects. By animating the input values over time, you can create smooth, flowing motion.
Conclusion
Perlin Noise is a versatile and powerful tool that has found applications in a wide range of fields, from generative art to game development. Its ability to create natural, organic patterns makes it a favorite among artists and developers alike. Whether you're creating a digital masterpiece or a sprawling virtual world, understanding Perlin Noise opens up a world of possibilities.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Art with Code (psst, it's free!).
FAQ
What is Perlin Noise used for?
Perlin Noise is commonly used in generative art, procedural generation, game development, and animation. It helps create natural-looking patterns and textures, as well as realistic terrains and dynamic visual effects.
How does Perlin Noise differ from simple random noise?
Perlin Noise produces smooth, coherent patterns, whereas simple random noise (like TV static) is completely unstructured. This makes Perlin Noise ideal for simulating natural phenomena.
Can Perlin Noise be used in 3D applications?
Yes, Perlin Noise can be extended to three dimensions (and beyond) to create 3D textures and terrains. This is particularly useful in game development and 3D modeling.
What is fractal noise?
Fractal noise is created by combining multiple layers (or octaves) of Perlin Noise at different scales. This technique produces more complex and detailed patterns, often used in landscape generation.
Are there alternatives to Perlin Noise?
Yes, there are other noise algorithms like Simplex Noise and Worley Noise. Each has its own strengths and weaknesses, and the choice depends on the specific requirements of the project.