Understanding Java Syntax
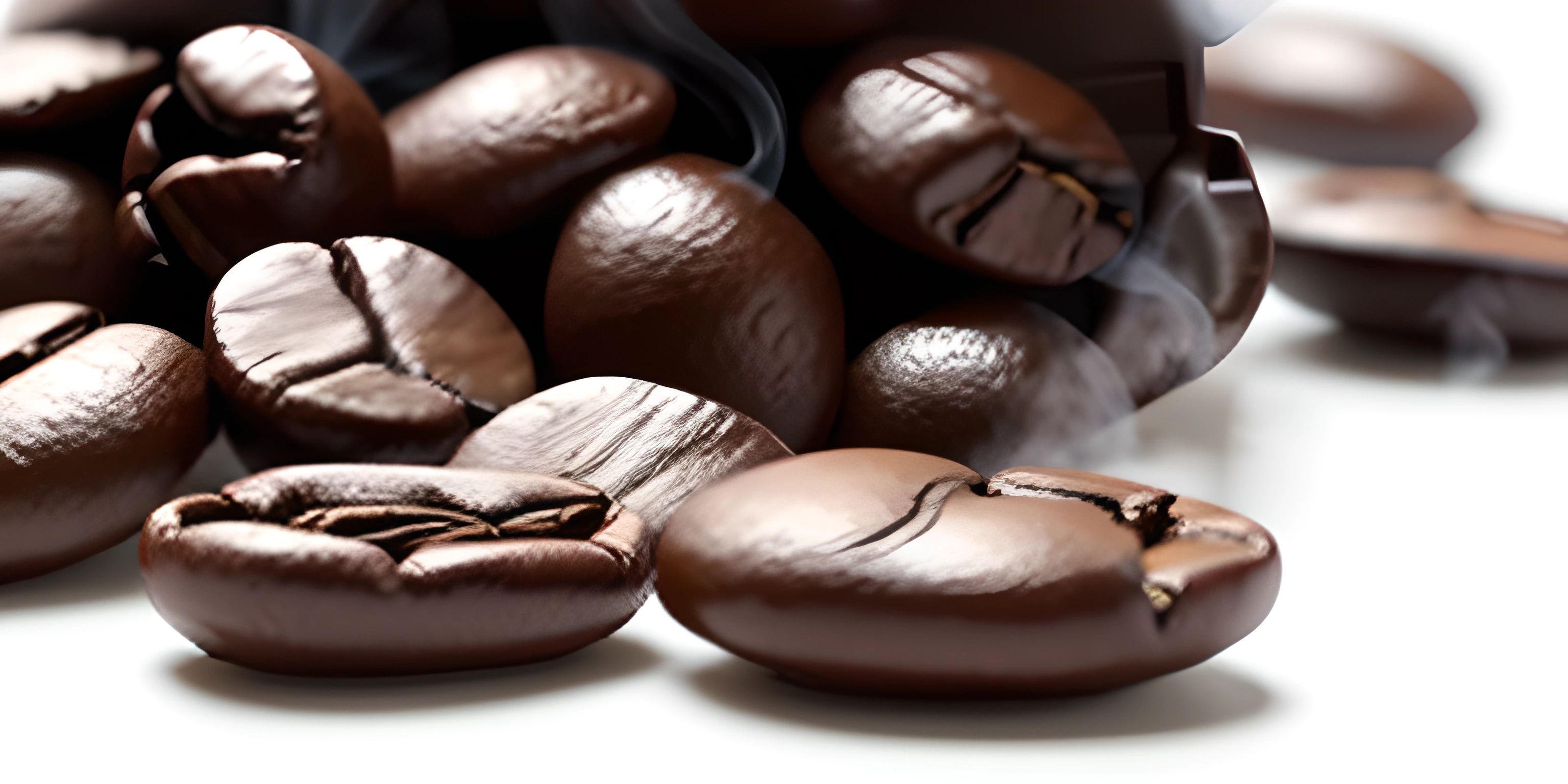
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Java is a widely-used programming language known for its versatility, security, and simplicity. To write effective Java code, it's essential to understand the basic syntax and structure that underpin the language. So let's dive in!
Java Structure
A Java program is composed of classes, methods, and statements. The basic structure of a Java program is as follows:
-
Class: A class is a blueprint for creating objects. It defines the properties and methods that the objects can have. Each Java file should have at least one class.
-
Method: A method is a group of statements that perform a specific task. Methods are defined within a class. The most important method in a Java program is the
main
method, which is the entry point of the program. -
Statements: Statements are instructions that perform a specific action, such as declaring variables or calling methods. They are written within methods.
Here's a simple example of a Java program:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Let's break it down:
-
public class HelloWorld
: This line declares a public class namedHelloWorld
. The class name should match the file name (HelloWorld.java). -
public static void main(String[] args)
: This line defines themain
method, which is the entry point of the program. When the program runs, themain
method is executed first. -
System.out.println("Hello, World!");
: This statement prints "Hello, World!" to the console. It is contained within themain
method.
Java Syntax
Now that you're familiar with the basic structure of a Java program, let's discuss some important syntax rules:
-
Case Sensitivity: Java is case-sensitive, meaning that
hello
andHello
are treated as different identifiers. -
Semicolons: Every statement in Java must end with a semicolon (
;
). Forgetting a semicolon can lead to compilation errors. -
Curly Braces: Curly braces (
{}
) are used to define the scope of classes, methods, and other blocks of code. Make sure to properly match opening and closing braces. -
Comments: Java supports single-line comments (using
//
) and multi-line comments (using/* */
). Comments are ignored by the compiler and serve as documentation for your code. -
Naming Conventions: Java follows specific naming conventions for classes, methods, and variables:
- Classes: Use PascalCase (e.g.,
MyClass
) - Methods: Use camelCase (e.g.,
myMethod()
) - Variables: Use camelCase (e.g.,
myVariable
)
- Classes: Use PascalCase (e.g.,
-
Access Modifiers: Java uses access modifiers to control the visibility of classes, methods, and variables. Common access modifiers include
public
,private
, andprotected
. -
Data Types: Java has several primitive data types (e.g.,
int
,double
,char
) and reference data types (e.g.,String
,Object
). -
Operators: Java supports various operators for performing arithmetic, comparison, and logical operations.
With these syntax rules in mind, you're ready to start writing Java code! Remember, practice makes perfect. The more you code, the more familiar you'll become with Java's syntax and structure. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What is the basic structure of a Java program?
The basic structure of a Java program consists of the following components:
- Class declaration: Every Java program begins with a class declaration. A class is a blueprint for creating objects in Java. The class name should be written in PascalCase (e.g.,
MyClassName
).
public class MyClassName { // Your code goes here }
- Main method: The main method is the entry point of your Java program. It is where the Java Virtual Machine (JVM) starts executing your code. It must be written inside the class declaration.
public static void main(String[] args) { // Your code goes here }
- Statements: Inside the main method, you'll write statements to perform various tasks, such as declaring variables, calling methods, and performing calculations.
How do I declare variables and assign values in Java?
In Java, you declare variables by specifying the data type followed by the variable name. You can also assign a value to the variable using the assignment operator =
. For example, to declare an integer variable and assign a value, you would write:
int myNumber = 42;
Remember that each statement in Java must end with a semicolon (;
).
What are the rules for naming variables and methods in Java?
In Java, variable and method names must follow these rules:
- They can contain letters (a-z, A-Z), digits (0-9), underscores (_), and dollar signs ($).
- They cannot start with a digit.
- They are case-sensitive.
- They cannot be Java reserved words (e.g.,
int
,class
,if
, etc.). When naming variables, it's best to use camelCase (e.g.,myVariableName
). Method names should also follow camelCase and be descriptive of their purpose (e.g.,calculateSum
).
Can you give a brief overview of Java data types?
Java has two categories of data types: primitive data types and reference data types.
- Primitive data types: These are the basic data types in Java, such as
int
,float
,double
,char
,boolean
,byte
,short
, andlong
. They directly store values in memory. - Reference data types: These are non-primitive data types, such as classes, arrays, and interfaces. They store references to memory locations where the actual data is stored.
For example, when declaring a variable of type
String
(a reference data type), you would write:
String myString = "Hello, world!";
How do I write comments in Java?
In Java, you can write comments in two ways:
- Single-line comments: Use
//
to write a single-line comment. Anything after//
on the same line will be ignored by the compiler.
// This is a single-line comment
- Multi-line comments: Use
/*
and*/
to write multi-line comments. Everything between these symbols will be ignored by the compiler.
/* This is a multi-line comment */