Python Turtle Basics
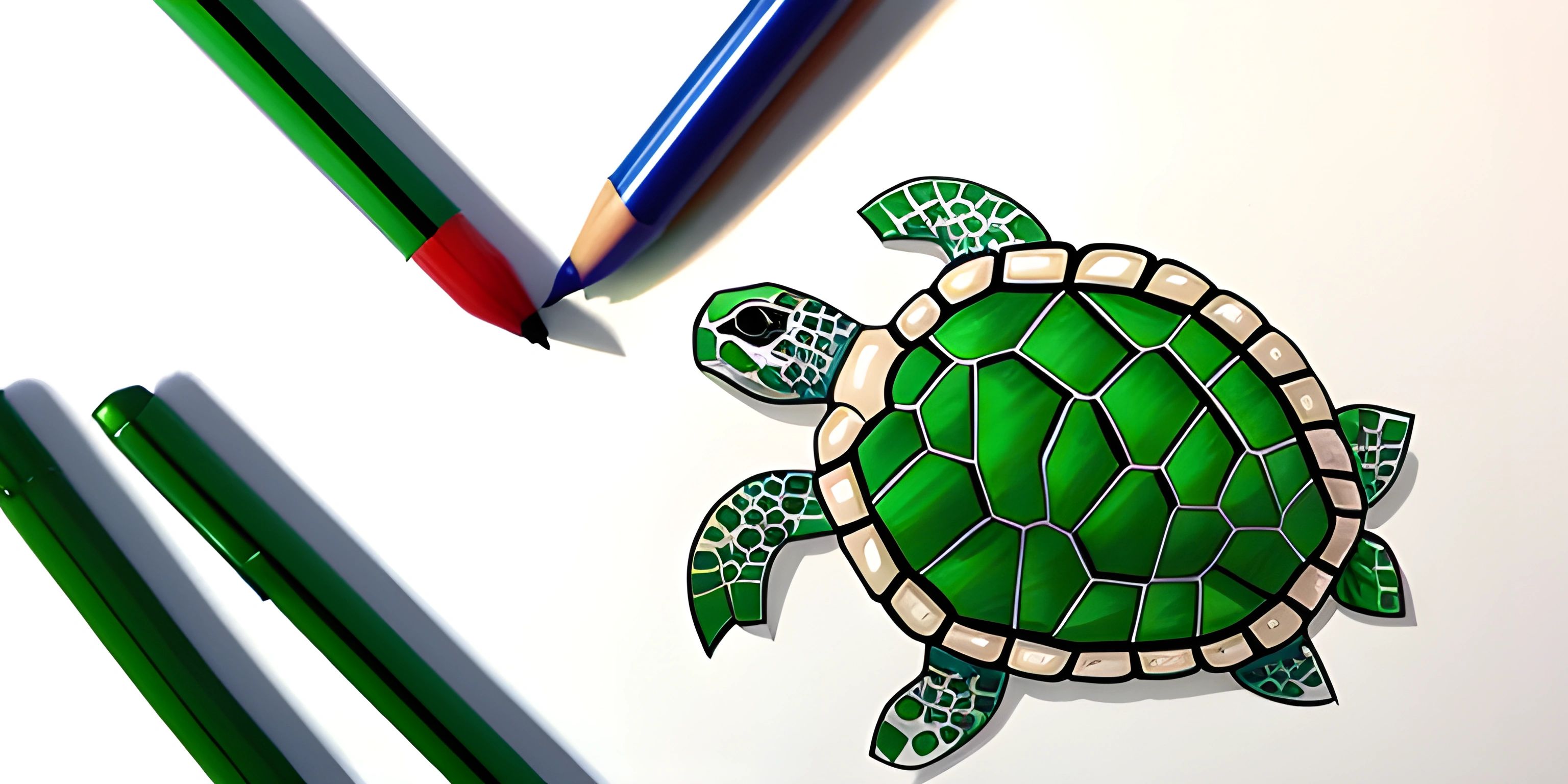
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming meets art in the world of Python Turtle, a library that lets you create captivating visuals by controlling a tiny turtle that crawls across a digital canvas. But don't worry, no actual turtles were harmed in the making of your masterpieces! The turtle is simply a metaphorical way to understand how the library works.
Setup
To get started, you'll need to install the Turtle library, which comes pre-installed with Python. Simply import it using the following command:
import turtle
With that out of the way, let's meet your new digital friend and learn how to make them draw some shapes.
Creating a Turtle
To create a turtle, you'll need to instantiate a new Turtle
object. This will also open a window with a canvas where your turtle will roam.
my_turtle = turtle.Turtle()
Now that you have a turtle, you can instruct it to perform various tasks, like moving forward or turning.
Basic Drawing Commands
Here are some simple commands to get your turtle moving and drawing:
Move Forward
To move your turtle forward by a specified distance, use the forward()
method:
my_turtle.forward(100) # Moves the turtle forward by 100 units
Turn Left or Right
You can change your turtle's direction by using the left()
and right()
methods:
my_turtle.left(90) # Turns the turtle 90 degrees to the left my_turtle.right(45) # Turns the turtle 45 degrees to the right
Pen Control
Your turtle draws by dragging a pen across the canvas. You can control the pen with these commands:
my_turtle.penup() # Lifts the pen up, so the turtle won't draw while moving my_turtle.pendown() # Puts the pen down, allowing the turtle to draw again
Changing Colors
Add some flair to your artwork by changing the pen color with the pencolor()
method:
my_turtle.pencolor("red") # Changes the pen color to red
Drawing a Square
Now that you're familiar with some basic commands, let's create a simple program using Python Turtle to draw a square:
import turtle my_turtle = turtle.Turtle() # Draw a square for _ in range(4): my_turtle.forward(100) my_turtle.right(90) # Close the window when clicked turtle.done()
This program moves the turtle forward by 100 units, then turns right by 90 degrees, repeating this process four times to complete the square.
Wrapping Up
Python Turtle is a fun and engaging way to learn programming concepts while creating delightful visuals. You've taken your first steps by learning the basics of using the Turtle library. As you progress, you'll discover more advanced techniques, such as loops, functions, and conditional statements, to create even more intricate designs.
So, grab your digital turtle and start painting the canvas of infinite possibilities!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).