PHP Intro for Web Development
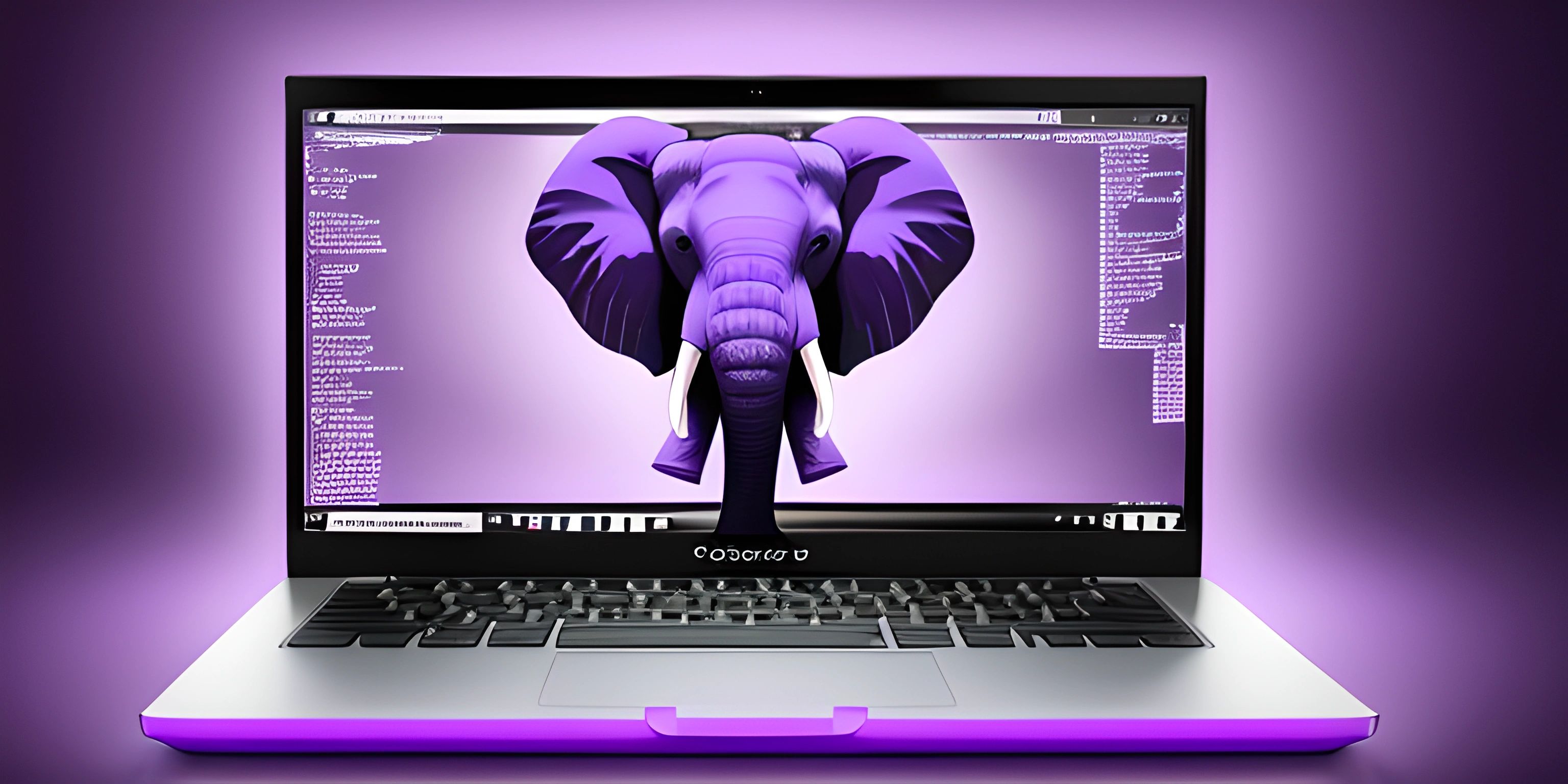
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
PHP, which once stood for "Personal Home Page" but now means "PHP: Hypertext Preprocessor," is a widely-used, general-purpose scripting language perfect for web development. With its roots dating back to 1994 when Rasmus Lerdorf first created it, PHP has grown into a powerful server-side language that powers many of the websites we know and love today.
Server-side Scripting with PHP
PHP is a server-side scripting language, which means it runs on the server before being delivered to the client's browser. This is in contrast to client-side scripting languages such as JavaScript, which run in the browser itself.
The magic of PHP lies in its ability to generate dynamic content. With PHP, you can create web pages that change based on user input, time of day, or any other factors you can imagine. This allows for a more interactive and personalized experience for users visiting your website.
Here's an example of how PHP can be used to display a simple "Hello, World!" message on your web page:
<!DOCTYPE html> <html> <head> <title>PHP Intro</title> </head> <body> <?php echo "Hello, World!"; ?> </body> </html>
In this example, we have embedded PHP code right inside our HTML using the <?php
and ?>
tags. The echo
statement is used to output the "Hello, World!" message to the browser.
Working with Variables and Functions
PHP offers a wealth of variables and functions to help you create dynamic web applications. Variables in PHP are denoted by a dollar sign ($
) followed by the variable name.
Here's an example of using variables to greet a user with a personalized message:
<?php $name = "John Doe"; echo "Hello, " . $name . "!"; ?>
In this example, we've created a variable called $name
, assigned it the value "John Doe," and then used it in our echo
statement. The period (.
) is used to concatenate (join) strings in PHP.
PHP also offers a vast array of built-in functions for various tasks, such as string manipulation, math operations, and working with dates and times. You can even create your own custom functions to help organize your code and make it more reusable. Here's an example of creating a function to greet users:
<?php function greetUser($name) { return "Hello, " . $name . "!"; } $username = "Jane Doe"; echo greetUser($username); ?>
We've defined a function called greetUser()
that accepts a single parameter, $name
, and returns a greeting string. We then call this function using our $username
variable and echo
the result.
Interacting with Databases
A key feature of PHP is its ability to interact with databases, such as MySQL, to store and retrieve data. This allows you to create web applications that can store user information, manage content, and more.
To work with databases in PHP, you can use the PDO (PHP Data Objects) extension, which provides a consistent and easy-to-use interface for accessing databases.
Here's a simple example of using PDO to connect to a MySQL database and retrieve data:
<?php $dsn = "mysql:host=localhost;dbname=my_database"; $username = "db_user"; $password = "db_password"; try { $connection = new PDO($dsn, $username, $password); $connection->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); $sql = "SELECT * FROM users"; $statement = $connection->prepare($sql); $statement->execute(); $result = $statement->fetchAll(PDO::FETCH_ASSOC); foreach ($result as $row) { echo "Name: " . $row["name"] . "<br>"; echo "Email: " . $row["email"] . "<br>"; echo "<hr>"; } } catch (PDOException $error) { echo "Error: " . $error->getMessage(); } $connection = null; ?>
In this example, we're connecting to a MySQL database, executing a simple SQL query to fetch all records from the "users" table, and then displaying the name and email of each user.
Conclusion
PHP is a powerful and versatile language for web development, offering dynamic content generation, database interaction, and much more. By mastering the basics of PHP, you'll be well on your way to creating engaging and interactive web applications for your users. So, go ahead and explore the vast world of PHP and bring your web development skills to new heights.
FAQ
What is PHP and why is it important for web development?
PHP, or Hypertext Preprocessor, is a widely-used open-source scripting language designed specifically for web development. It allows developers to create dynamic and interactive web pages by embedding server-side scripts directly within HTML. PHP plays a crucial role in web development because it provides an efficient and flexible way to build web applications and manage databases.
Can you give a brief history of PHP and how it has evolved?
PHP was originally created by Rasmus Lerdorf in 1994 as a set of Perl scripts called "Personal Home Page Tools." Over time, PHP evolved into a full-fledged scripting language, with PHP/FI (Form Interpreter) as its first iteration. In 1997, PHP 3 was released, introducing a new language parser and many new features. PHP 4, released in 2000, introduced the Zend Engine, which improved performance and added object-oriented programming capabilities. PHP 5, released in 2004, further enhanced its object-oriented programming features and introduced improved database connectivity. The current major version, PHP 7, was released in 2015, offering significant performance improvements and modern language features.
How do I start using PHP in web development?
To start using PHP in web development, you need to have a server with PHP installed and configured. You can either use a web hosting service that supports PHP or set up a local development environment using software like XAMPP or MAMP. Once your server is ready, create a file with a ".php" extension and write your PHP code within PHP tags <?php
and ?>
embedded in your HTML file. When you access the file through your web server, the PHP code will be executed, and the resulting output will be displayed in the browser.
Can you provide a simple example of PHP code?
Sure! Here's a basic example of PHP code that displays a "Hello, World!" message:
<?php echo "Hello, World!"; ?>
In this example, the echo
statement is used to output the text "Hello, World!" to the web page. When the PHP code is executed, the server replaces the PHP block with the output generated by the echo
statement.
How does PHP interact with databases?
PHP can interact with various databases, such as MySQL, PostgreSQL, SQLite, and more, to store, retrieve, and manipulate data. PHP provides several functions and extensions to connect to databases, execute queries, and manage database records. The most popular way to interact with databases in PHP is through the MySQLi and PDO (PHP Data Objects) extensions, which provide a consistent and secure way to access databases and prevent SQL injection attacks. Here's a simple example of connecting to a MySQL database using PDO:
<?php $dsn = "mysql:host=localhost;dbname=mydb"; $username = "username"; $password = "password"; try { $connection = new PDO($dsn, $username, $password); echo "Connected successfully!"; } catch (PDOException $e) { echo "Connection failed: " . $e->getMessage(); } ?>