Introduction to JavaScript
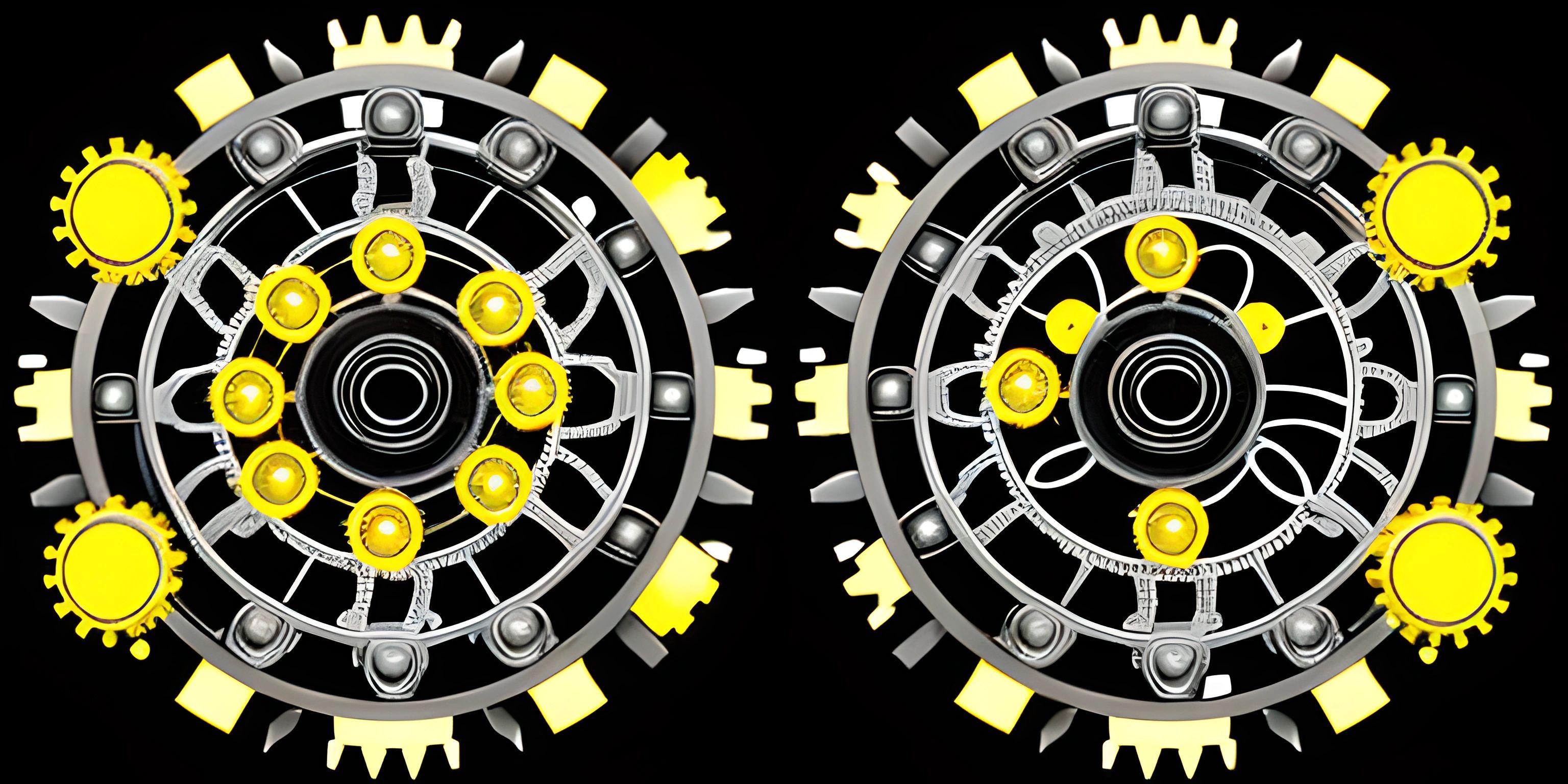
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Get ready to dive into the world of JavaScript, one of the most popular and versatile programming languages in the world! JavaScript is primarily known for its power to breathe life into web pages, making them interactive and dynamic.
What is JavaScript?
JavaScript is a scripting language that is mainly used in web development to enhance HTML and CSS by adding interactivity and dynamic content to web pages. It was created in 1995 by Brendan Eich at Netscape and has since become an essential part of the modern web development trifecta, alongside HTML and CSS.
Unlike compiled languages like C++ or Java, JavaScript is interpreted by the browser, meaning the browser reads and executes the code line-by-line on the fly. This makes JavaScript particularly well-suited for web applications, as it can easily adapt to different browsers and platforms.
Why Use JavaScript?
There are many reasons to embrace JavaScript for your web development projects, but here are a few key benefits:
-
Interactivity: JavaScript allows you to create dynamic and interactive web pages. You can use it to respond to user input, manipulate DOM elements, and even create games and animations.
-
Versatility: JavaScript is not limited to just client-side web development. With the advent of technologies like Node.js, you can use JavaScript for server-side programming and even develop desktop and mobile applications.
-
Community: JavaScript has a massive, vibrant community that is constantly growing and evolving. There are tons of resources, libraries, and frameworks available to help you get started and streamline your development process.
-
Browser Support: JavaScript is supported by all modern web browsers, making it the de facto language for client-side web development.
JavaScript Basics
Now that we've covered what JavaScript is and why you should use it, let's take a look at some fundamental concepts to get you started.
Variables
In JavaScript, variables are used to store data. You can declare a variable using the var
, let
, or const
keyword, followed by the variable name. Here's an example:
var message = "Hello, JavaScript!";
Functions
Functions are blocks of reusable code that can be called with a given set of input parameters. Functions help keep your code organized and modular. Here's an example of a simple function definition and call:
function greet(name) { console.log("Hello, " + name + "!"); } greet("Cratecoder");
Control Structures
JavaScript provides several control structures to help you manage the flow of your code, such as if
statements, for
loops, and while
loops. Here's an example of a basic if
statement:
var age = 18; if (age >= 18) { console.log("You're an adult!"); } else { console.log("You're still a minor."); }
Objects
JavaScript is an object-oriented language, which means that you can use objects to model real-world entities and their interactions. Objects in JavaScript are collections of key-value pairs, also known as properties. Here's an example of an object representing a person:
var person = { name: "Cratecoder", age: 25, greet: function() { console.log("Hello, my name is " + this.name + "."); } }; person.greet();
Conclusion
This introduction to JavaScript has only scratched the surface of this powerful and versatile language. As you continue your journey, you'll discover a vast array of features and capabilities, leading you to create amazing web applications and beyond. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is JavaScript and why is it important in web development?
JavaScript is a powerful and versatile scripting language that plays a crucial role in web development. It allows developers to add interactivity, animations, and dynamic content to websites, making them more engaging and user-friendly. With JavaScript, you can create responsive web applications, handle user input, and manipulate the Document Object Model (DOM) to update the web page content in real-time.
How can I include JavaScript in my web pages?
You can include JavaScript in your web pages in two ways: inline and external. To write inline JavaScript, use the <script>
tag inside your HTML file, like this:
<script> // Your JavaScript code goes here </script>
However, for better organization and maintainability, it's recommended to use external JavaScript files. Create a separate .js
file and link it to your HTML file using the <script>
tag with the src
attribute:
<script src="your-javascript-file.js"></script>
What are some basic JavaScript concepts I should be familiar with?
As a beginner, you should be familiar with the following JavaScript concepts:
- Variables: Containers for storing values, defined using the
let
,const
, orvar
keywords. - Data types: JavaScript has different data types like string, number, boolean, array, and object.
- Functions: Reusable blocks of code that can be called with or without arguments and may return a value.
- Conditional statements:
if
,else if
, andelse
statements that control the flow of your code based on conditions. - Loops:
for
,while
, anddo...while
loops that enable you to execute a block of code repeatedly.
Is JavaScript the same as Java?
No, JavaScript and Java are two distinct programming languages. Although their names are similar, they serve different purposes and have different syntax, features, and use cases. Java is a compiled, statically-typed language mainly used for creating large-scale applications, while JavaScript is an interpreted, dynamically-typed language primarily used for web development.
Can JavaScript be used for server-side programming?
Yes, JavaScript can be used for server-side programming with the help of Node.js, an open-source runtime environment that enables you to run JavaScript on the server side. With Node.js, you can create web servers, APIs, and interact with databases, extending JavaScript's capabilities beyond the browser.