Recursion Explained
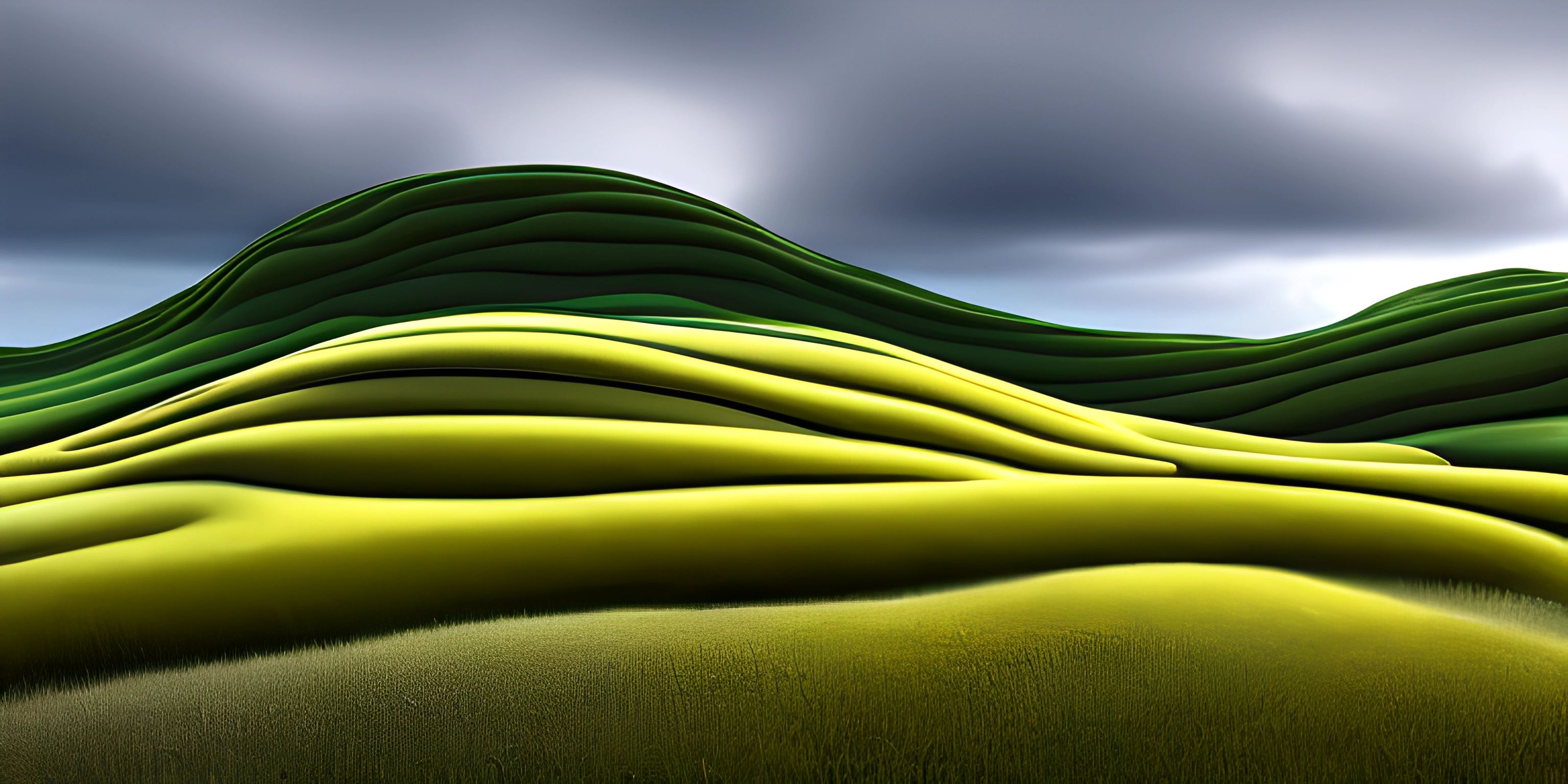
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Recursion is a powerful programming technique that lets a function call itself in order to solve a problem. It might sound like a mind-bending concept at first, but once you grasp the basics, you'll be able to tackle complex problems with ease. So, let's dive into the world of recursion and see how it works.
Recursion Basics
In a nutshell, recursion is when a function calls itself in order to break down a larger problem into smaller, more manageable pieces. This process continues until a base case is reached, at which point the function stops calling itself and starts returning values.
Here's a classic example to help illustrate the idea. Imagine you want to find the factorial of a number (written as n!
). The factorial is the product of all positive integers less than or equal to n
. For example, 5! = 5 * 4 * 3 * 2 * 1 = 120
. We can solve this problem recursively:
def factorial(n): if n == 1: return 1 else: return n * factorial(n - 1)
In this example, the function factorial
calculates the factorial of a number n
. The base case is n == 1
, where the function returns 1
. Otherwise, the function calls itself with the argument n - 1
and multiplies the result by n
. This process continues until the base case is reached.
Advantages and Disadvantages
Recursion has several pros and cons, and understanding them will help you decide when it's appropriate to use.
Advantages
- Simplicity: Recursive solutions can often be much simpler and more elegant than their iterative counterparts.
- Problem-solving: Recursion is a natural way to break down complex problems into smaller, more manageable pieces.
Disadvantages
- Overhead: Recursive functions can have a higher overhead due to the extra function calls, which can lead to increased memory usage and slower performance.
- Stack overflow: Deeply recursive functions can cause a stack overflow, which occurs when the call stack (a data structure used to store information about active function calls) reaches its maximum size.
Tips for Using Recursion
- Identify the base case: Determine the simplest version of the problem, which can be solved without further recursion.
- Formulate the recursive step: Figure out how to break the problem down into smaller pieces and how the function should call itself.
- Think about efficiency: Be mindful of the potential overhead and risks of using recursion. Consider using memoization or converting the recursive function to an iterative one if necessary.
Common Recursive Algorithms
Here are a few examples of popular recursive algorithms:
- Binary search: A fast search algorithm that works on sorted arrays by repeatedly dividing the search interval in half.
- Fibonacci sequence: A sequence of numbers where each number is the sum of the two preceding ones, starting from 0 and 1.
- Tree traversal: Algorithms for visiting all the nodes in a tree data structure in a specific order.
Now you're equipped with the knowledge of recursion, its pros and cons, and some tips for using it effectively. With practice, recursion can become an invaluable tool in your programming arsenal. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is recursion in programming?
Recursion is a programming technique where a function calls itself to solve a problem. It breaks down a complex problem into smaller, manageable subproblems. This method is particularly useful for tasks that have a naturally recursive structure, such as traversing a file system, generating permutations, or solving mathematical problems like the Fibonacci sequence and factorials.
How does a recursive function work?
A recursive function works by having a base case and a recursive case. The base case is the simplest version of the problem, which can be solved directly without any further recursion. The recursive case is where the function calls itself to solve a smaller subproblem. The function continues to call itself until it reaches the base case, and then the solutions are combined to solve the original problem. Here's an example of a recursive function to calculate the factorial of a number:
def factorial(n): if n == 0: return 1 # This is the base case else: return n * factorial(n-1) # This is the recursive case
What are some common applications of recursion in programming?
Recursion is often used in problems that have a naturally recursive structure, such as:
- Traversing a file system, where each folder can contain subfolders and files.
- Generating permutations and combinations.
- Solving mathematical problems like the Fibonacci sequence, factorials, and Tower of Hanoi.
- Tree and graph traversals, such as depth-first search and breadth-first search.
- Divide-and-conquer algorithms, like merge sort and quick sort.
What are the advantages and disadvantages of using recursion?
Advantages of recursion:
- Recursive solutions are often more elegant and easier to understand compared to iterative ones.
- It can simplify complex problems by breaking them down into smaller subproblems. Disadvantages of recursion:
- Recursive function calls can lead to a large number of function calls, consuming more memory and affecting performance.
- Debugging recursive functions can be more difficult due to multiple function calls and the call stack.
How can I optimize my recursive functions for better performance?
To optimize recursive functions, you can use techniques such as memoization or dynamic programming. Memoization involves storing the results of expensive function calls and returning the cached result when the same inputs are encountered again. This can significantly reduce the number of redundant calculations and improve the performance of your recursive functions. Here's an example of a memoized Fibonacci function:
def memoize(f): memo = {} def helper(x): if x not in memo: memo[x] = f(x) return memo[x] return helper def fibonacci(n): if n == 0: return 0 elif n == 1: return 1 else: return fibonacci(n-1) + fibonacci(n-2) fibonacci = memoize(fibonacci)