Understanding Tail Recursion
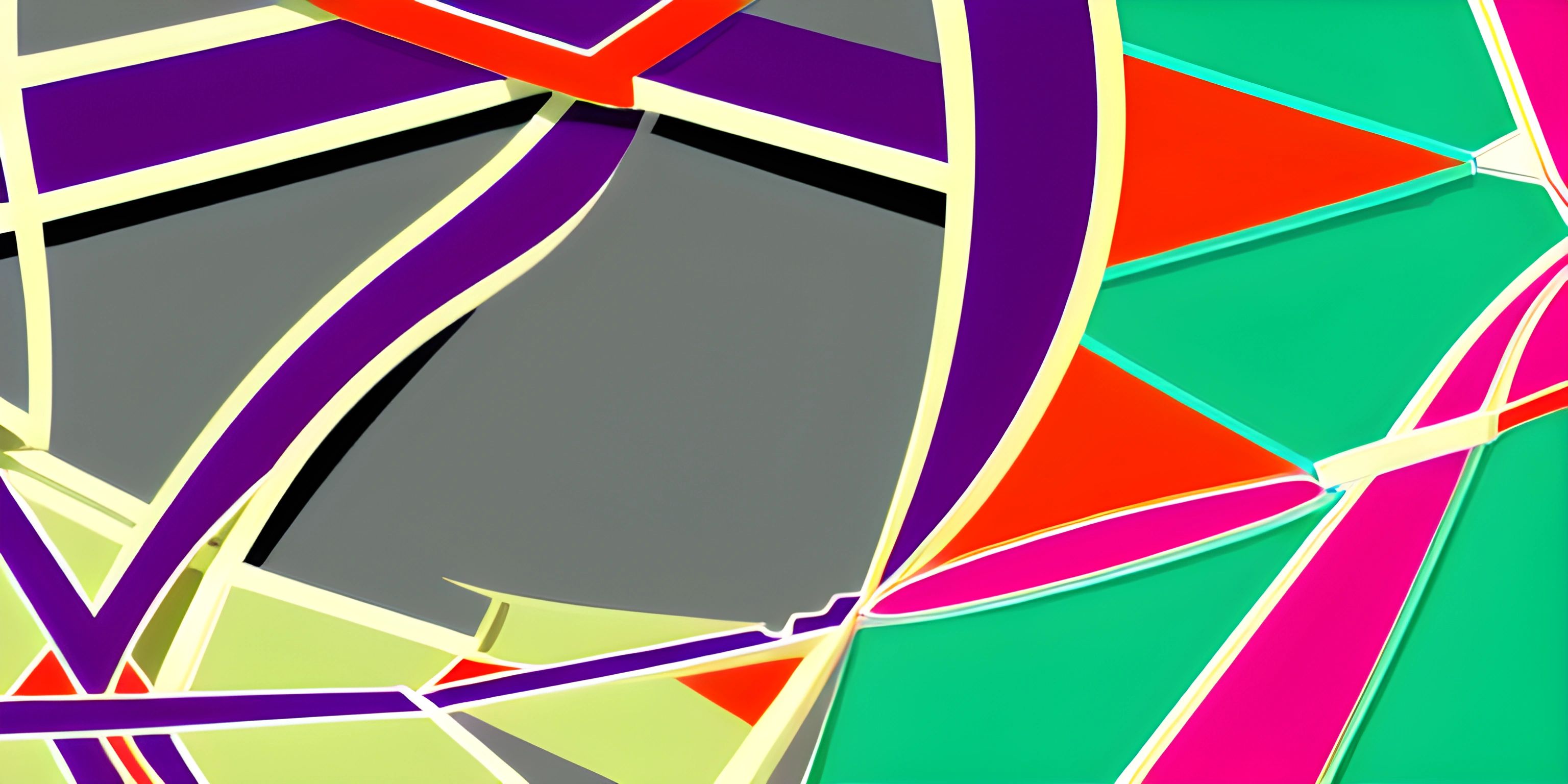
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the labyrinths of programming concepts, recursion is a beast that often rears its head, sometimes to the sheer delight of programmers, but often to their utter confusion. Today, we're going to shine a light on one of recursion's most efficient and elegant forms: tail recursion.
What is Tail Recursion?
Imagine standing on the edge of a fractal, a mathematical shape that repeats itself infinitely. You hop from one level of the fractal to the next, journeying deeper and deeper into its structure. This is a bit like recursion, except recursion has a safety net - a base case that eventually stops you from falling further. Now, imagine if each hop you took didn't make you carry the weight of all the previous steps. That, my friends, is tail recursion.
In traditional recursion, each recursive function call carries with it a bit of memory — a breadcrumb trail leading back to the original call. This is because after executing the recursive call, there might be other operations that the function needs to perform. In tail recursion, however, the recursive call is the last operation. This means it can "forget" about the rest of the calls, because there's nothing left to do.
Let's take a look at a simple example using Python.
def factorial(n): if n == 0: return 1 else: return n * factorial(n - 1)
This is a classic example of a recursive function - it calculates the factorial of a number. However, it's not tail recursive. Why? Because after calling factorial(n - 1)
, it still needs to multiply the result by n
.
Let's refactor this function to make it tail recursive.
def factorial(n, acc=1): if n == 0: return acc else: return factorial(n - 1, n * acc)
In this tail recursive version, as soon as we hit our base case (n == 0
), we immediately return our result (acc
). There's no more computation left to do after our recursive call. This means that Python (or any language that supports tail recursion optimization) can optimize this function to use constant stack space, instead of linear.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is tail recursion?
Tail recursion is a form of recursion where the recursive call is the last operation in the function. This allows the function to be optimized to use constant stack space, instead of linear.
How does tail recursion differ from regular recursion?
In regular recursion, each recursive call carries with it some memory of the previous calls, because there might be other operations that need to be performed after the recursive call. In tail recursion, the recursive call is the last operation, so it can "forget" about the rest of the calls.
What are the advantages of tail recursion?
The main advantage of tail recursion is efficiency. Because the recursive call is the last operation, the function can be optimized to use constant stack space, instead of linear. This can result in significant performance improvements for deep recursion.
How can I write a tail recursive function?
To write a tail recursive function, ensure that the recursive call is the last operation in your function. You might need to use an accumulator parameter to store the result of the computation up to the current point.
Can all recursive functions be converted to tail recursive functions?
In theory, yes, all recursive functions can be converted to tail recursive functions. However, the conversion might not always be straightforward and could potentially make the function more complex or difficult to understand. Always consider the trade-offs before deciding to convert a recursive function to a tail recursive one.