Recursion in Programming
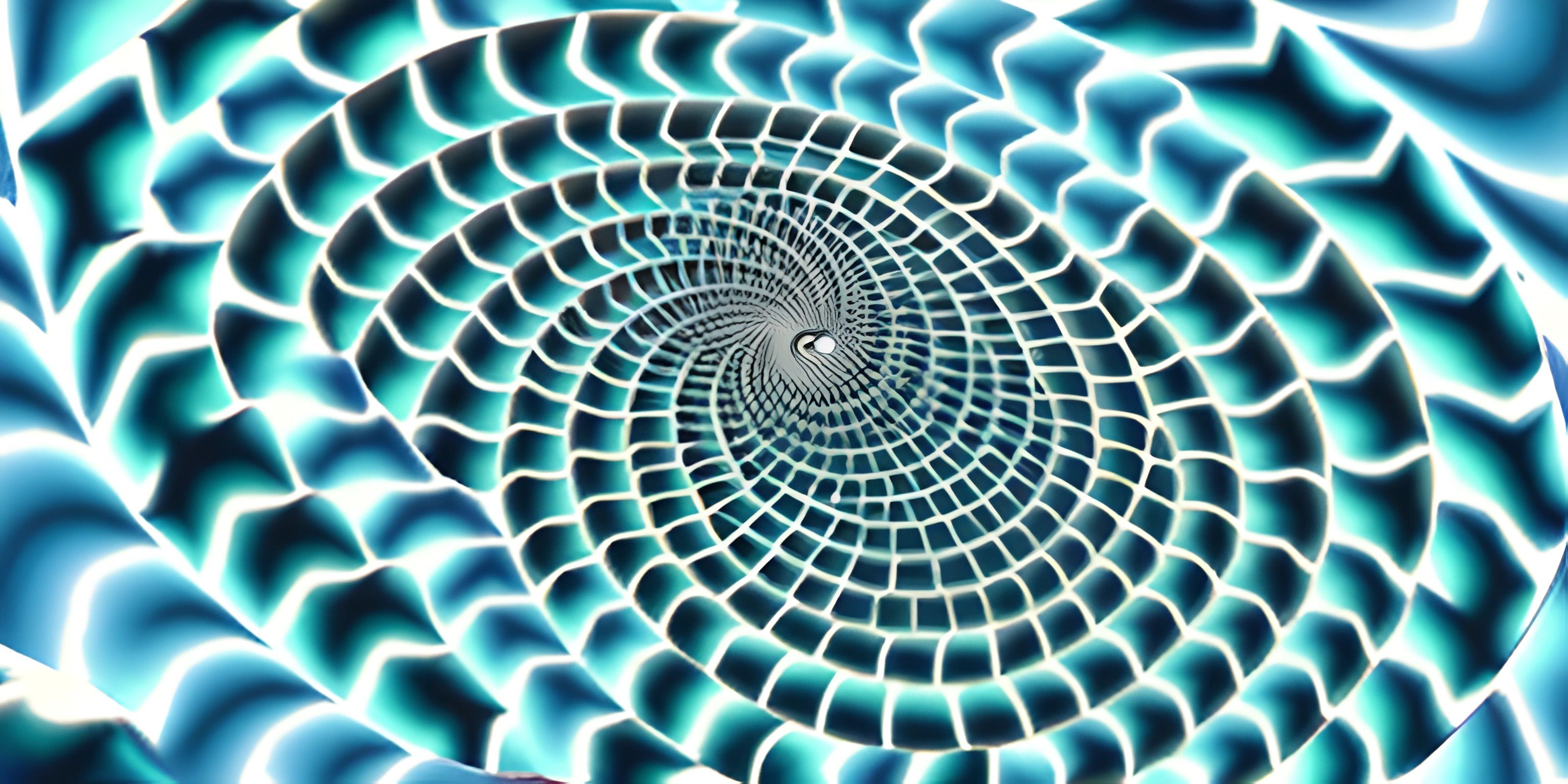
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Recursion is a powerful concept in programming, allowing us to break down complex problems into simpler, more manageable ones. It's like a Russian nesting doll, where each level peels away to reveal another smaller doll inside, until we reach the tiniest doll at the center.
What is Recursion?
Recursion is a technique in which a function calls itself, either directly or indirectly, to solve a problem. The function continues to call itself until it reaches a base case or a condition that stops the recursion. To understand recursion, let's first explore its key components: the recursive call and the base case.
Recursive Call
A recursive call is when a function calls itself within its own definition. This helps to break down the problem into smaller, more manageable sub-problems. Let's take a look at a simple example in Python:
def countdown(n): if n <= 0: print("Blast off!") else: print(n) countdown(n - 1) countdown(3)
In this example, the countdown
function calls itself with the argument n - 1
. This continues until n
reaches 0, at which point the function stops calling itself and prints "Blast off!".
Base Case
The base case is a condition that stops the recursion from continuing indefinitely. It's like the smallest Russian nesting doll – once we reach it, we can start putting the other dolls back together. In the countdown
example, the base case is when n <= 0
. Without a base case, the function would call itself infinitely, causing a stack overflow error.
When to Use Recursion
Recursion can be used to solve a variety of problems, especially when the problem can be divided into smaller, similar sub-problems. Some common examples include calculating factorials, traversing data structures like trees and graphs, and implementing search algorithms like binary search and depth-first search.
However, recursion isn't always the best solution. It can lead to inefficiencies in both time and space complexity, as well as potential stack overflow errors for deep recursion. In some cases, using an iterative approach or dynamic programming may be more suitable.
Best Practices for Recursion
To effectively use recursion in your programming, keep the following best practices in mind:
- Clearly define the base case(s): Ensure that your recursive function has one or more base cases to prevent infinite recursion and stack overflow errors.
- Simplify the problem: Break the problem down into smaller, similar sub-problems that can be solved more easily through recursion.
- Be mindful of performance: Recursion can lead to inefficient solutions in terms of time and space complexity. Consider optimizing your solution with techniques like memoization or switching to an iterative approach if necessary.
In conclusion, recursion is a powerful tool in programming that can help you tackle complex problems by breaking them down into smaller, more manageable pieces. With a solid understanding of recursion, its components, and best practices, you'll be well-equipped to harness its power in your programs. Happy nesting!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is recursion in programming?
Recursion in programming is a technique where a function calls itself directly or indirectly in order to solve a problem. It breaks down the problem into smaller, more manageable subproblems, and solves them by repeatedly applying the same function until a base case (the simplest form of the problem) is reached.
Can you provide an example of a simple recursive function?
Sure! Here's a basic example of a recursive function in Python that calculates the factorial of a given number n
:
def factorial(n): if n == 0: return 1 else: return n * factorial(n - 1)
This function takes an integer n
as its input and returns the factorial of n
. It uses recursion by calling itself with the argument n - 1
until it reaches the base case, when n
is 0.
When should recursion be used in programming?
Recursion is best used when the problem can be broken down into smaller, similar subproblems. It's particularly helpful for solving problems that involve tree or graph traversal, such as searching for a specific value in a tree or calculating the shortest path between two nodes in a graph. However, recursion isn't always the most efficient solution, as it may lead to a large number of function calls and increased memory usage. In such cases, an iterative approach might be more suitable.
Are there any drawbacks to using recursion in programming?
While recursion can make code more elegant and easier to understand, it can also have some drawbacks:
- Performance: Recursive functions can be slower and require more memory compared to iterative solutions, as each function call adds a new frame to the call stack, consuming memory and processing resources.
- Stack overflow: If the recursion is too deep or infinite, it might lead to a stack overflow error, crashing the program.
- Limited language support: Some programming languages may not support or optimize recursion well, making it less efficient in those cases.
How can I prevent stack overflow in recursive functions?
To prevent stack overflow in recursive functions, you can take the following measures:
- Ensure you have a well-defined base case that stops the recursion when reached.
- Use tail recursion, where the recursive call is the last operation in the function, allowing some languages or compilers to optimize the function and prevent stack overflow.
- Consider converting your recursive function to an iterative one, using loops and data structures like stacks or queues to manage the problem-solving process without consuming call stack memory.