Call Stack in Depth
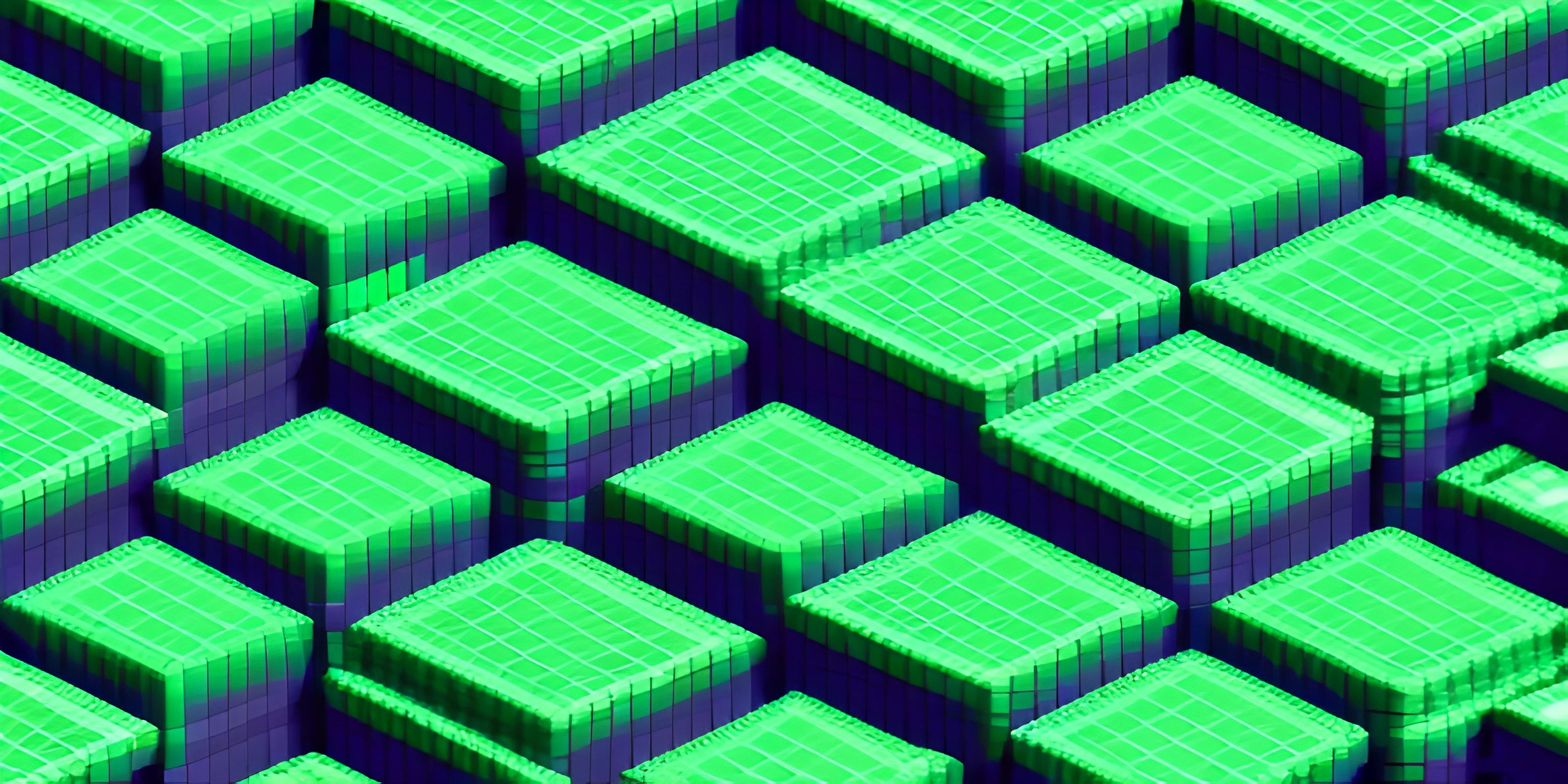
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Mastering the call stack is crucial for any programmer, as it plays a vital role in function calls and memory management. So buckle up, and let's dive into the world of call stacks!
What is a Call Stack?
In a nutshell, the call stack is a data structure that keeps track of function calls and their corresponding return addresses. When a function is called, its execution context (variables, parameters, and return address) is saved on the call stack. And when the function returns, the top-most context is popped off the stack, and execution resumes at the saved return address. It's like a stack of plates, where you can only add or remove a plate from the top.
Why is the Call Stack Important?
The call stack plays a crucial role in program execution and memory management for several reasons:
-
Managing Function Calls: The call stack ensures that each function returns control to the correct point in the program after completing its execution.
-
Preserving Execution Context: The call stack saves the context of each function call, allowing local variables and parameters to be used and discarded as needed.
-
Detecting Recursion: The call stack helps detect and handle recursion, which occurs when a function calls itself directly or indirectly.
-
Error Handling: The call stack is essential for error handling and debugging, as it provides a trace of function calls leading up to an error or crash.
Anatomy of a Call Stack
Let's break down the different components of a call stack:
-
Stack Frame: A stack frame represents an individual function call and contains information such as local variables, parameters, and return address. Each new function call creates a new stack frame, which is pushed onto the call stack.
-
Stack Pointer: The stack pointer is a register that points to the top of the call stack. It's used to keep track of the current stack frame and helps manage adding and removing frames.
-
Base Pointer: The base pointer, also known as the frame pointer, is another register that points to the beginning of the current stack frame. It's used to access local variables and parameters within the stack frame.
-
Heap and Stack Memory: Call stack memory is separate from the heap memory. The heap is used for dynamic memory allocation, while the stack is used for storing function call information.
Call Stack Limitations and Solutions
The call stack is not without its limitations, such as:
-
Stack Overflow: A stack overflow occurs when the call stack exceeds its allocated memory. This can happen due to excessive recursion or an overly deep nesting of function calls. To avoid stack overflows, be cautious with recursion and consider using tail recursion or iterative solutions when possible.
-
Memory Limitations: The call stack has a limited amount of memory, which can be a constraint for programs with complex or large data structures. In these cases, consider using heap memory for data storage instead.
Now that you've learned about the call stack and its importance, you're one step closer to becoming a programming wizard. With a solid grasp of the call stack, you'll be better equipped to debug your code, manage memory, and write efficient programs. Happy coding!
FAQ
What is a call stack and why is it important?
A call stack is a data structure used by the programming language to keep track of function calls and their respective execution contexts. It plays a crucial role in memory management and function execution. When a function is called, its details (such as arguments and return address) are pushed onto the call stack. Once the function has finished executing, its details are popped off the stack, and control is returned to the calling function. This ensures proper execution flow and efficient memory usage.
How does the call stack work with recursive functions?
In the case of recursive functions, the call stack keeps track of each nested function call. Every time the function is called recursively, a new stack frame is pushed onto the call stack with the function's details. This continues until the base case is reached. As the base case starts returning values, the stack frames are popped off one by one, and the values are returned to the calling functions. This process continues until the initial function call is complete.
Can the call stack overflow? What happens in such cases?
Yes, the call stack can overflow if there are too many function calls or the function call depth is too large. This usually happens when a function is called recursively without a proper base case. When the call stack overflows, the program crashes, and an error message is generated, such as "stack overflow" or "maximum call stack size exceeded."
How can I prevent a call stack overflow?
To prevent a call stack overflow, make sure that your recursive functions have a proper base case, and the recursion depth is limited. In some cases, you might want to consider using a different approach, such as iteration, to solve the problem without using recursion. Additionally, some languages offer tail call optimization (TCO), which allows the compiler to optimize recursive calls and reduce the stack usage, preventing potential overflows.
How can I visualize the call stack to better understand function execution?
Visualizing the call stack can be helpful for understanding the execution flow of your code. You can use debugging tools provided by your programming language or IDE to step through the code and observe the changes in the call stack. Alternatively, you can use online visualization tools like Python Tutor or create your own visualization by manually adding print statements to your code to track function entry and exit points.