Ruby Introduction
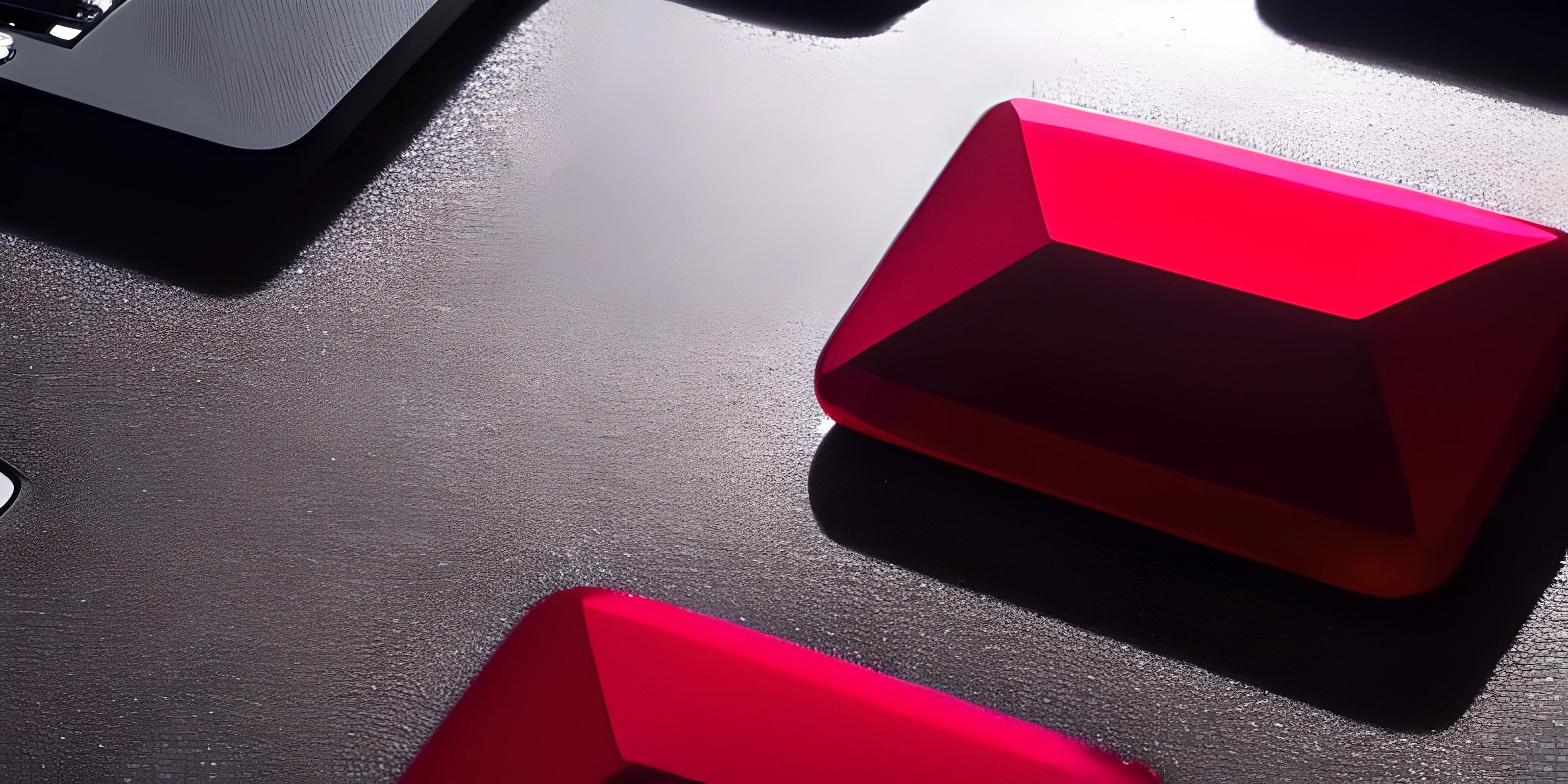
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the world of Ruby, a programming language designed to be fun and easy to use. Ruby is often praised for its elegance and readability, making it a popular choice among developers. In this article, we'll dive into the basics of Ruby and its role in web development.
What is Ruby?
Ruby is a dynamic, open-source, object-oriented programming language. It was created by Yukihiro "Matz" Matsumoto in the mid-1990s with the goal of creating a language that struck a balance between simplicity and power. Ruby's syntax is designed to be easy to read and write, which is why it's often described as a language that was built for developer happiness.
Ruby in Web Development
One of the most common uses for Ruby is in web development. This is largely due to the Ruby on Rails framework, which is a powerful and user-friendly tool for building web applications. Ruby on Rails, often shortened to Rails, allows developers to quickly build web applications using the Model-View-Controller (MVC) architectural pattern.
Rails is known for its emphasis on convention over configuration. This means that it has many built-in defaults and conventions that developers can follow, which can lead to faster development and cleaner code. Some of the key features of Rails include:
- ActiveRecord: An Object-Relational Mapping (ORM) system that simplifies database interactions.
- ActionView: A templating system for rendering views in a web application.
- ActionPack: A collection of components for handling web requests and responses.
Basic Ruby Syntax
Now that we have an idea of what Ruby is and how it's used in web development, let's take a closer look at its syntax. Here are some examples of basic Ruby constructs:
Variables
Variables in Ruby are used to store values. You can declare a variable by simply assigning a value to a name. Here's an example:
name = "Alice" age = 30
Strings
Strings in Ruby are sequences of characters enclosed in double quotes. You can concatenate strings using the +
operator or use string interpolation to insert a variable's value into a string. Here's an example:
greeting = "Hello, #{name}!" puts greeting
Arrays
Arrays in Ruby are ordered collections of items. You can create an array by enclosing a comma-separated list of items in square brackets. Here's an example:
fruits = ["apple", "banana", "cherry"]
You can access the elements of an array using their index, starting from 0. Here's an example:
first_fruit = fruits[0]
Loops
Loops in Ruby are used to perform repetitive tasks. Ruby offers various looping constructs, such as each
, while
, and until
. Here's an example using the each
loop:
fruits.each do |fruit| puts "I love #{fruit}!" end
Getting Started with Ruby
There you have it – a brief introduction to the world of Ruby and its role in web development. If you're interested in learning more about Ruby and diving deeper into the language, check out the official Ruby documentation, or explore various Ruby tutorials and courses available online. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).