Ruby on Rails Introduction
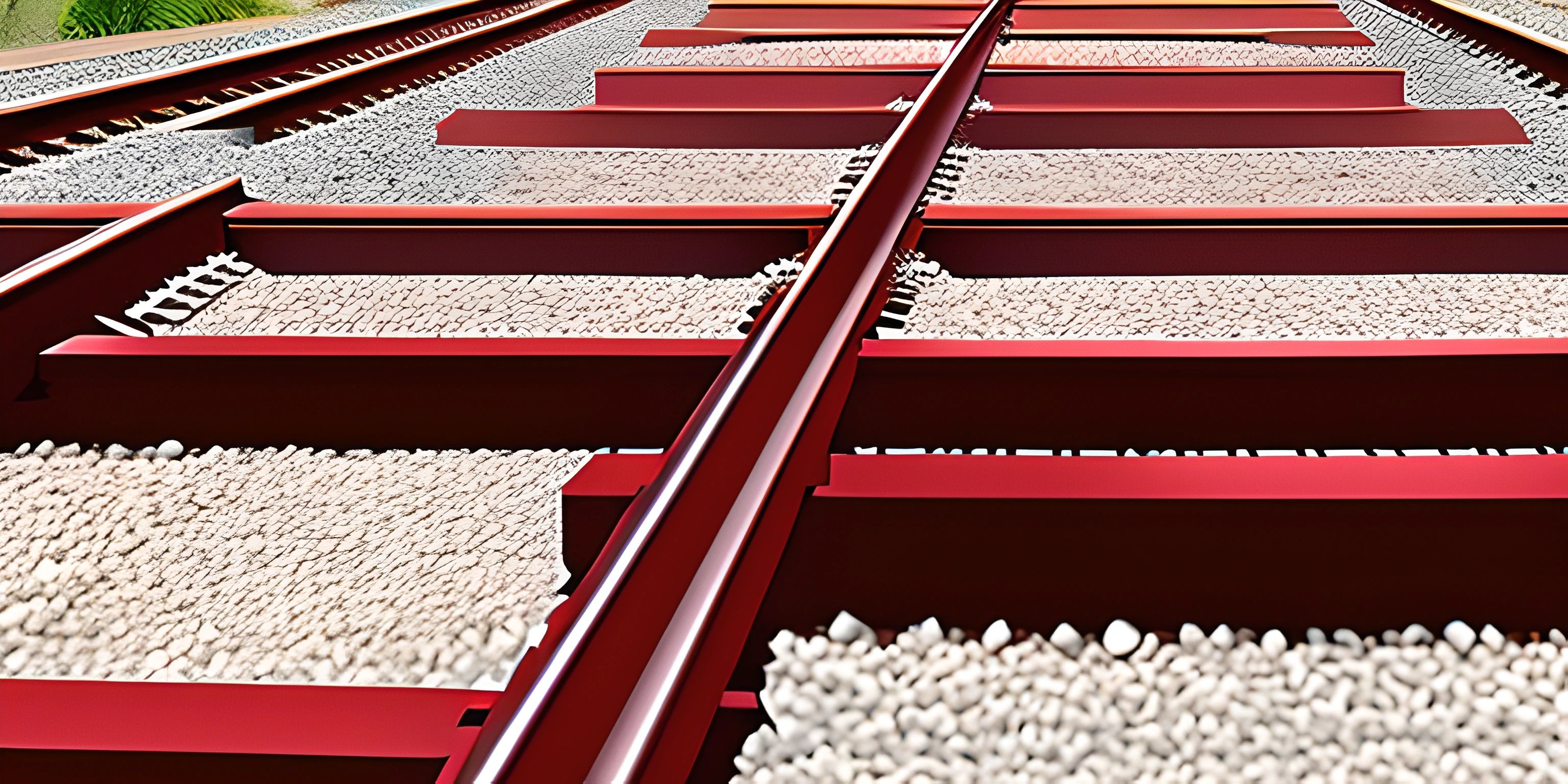
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ruby on Rails, commonly referred to as Rails, is a powerful web application framework built on the Ruby programming language. Its main goal is to simplify the development process by providing developers with a set of conventions and tools that make building web applications faster and more enjoyable.
Rails Philosophy
Rails follows two main principles: Convention over Configuration (CoC) and Don't Repeat Yourself (DRY). These guiding philosophies help streamline the development process and promote the use of best practices throughout a Rails application.
Convention over Configuration
Convention over Configuration means that Rails provides sensible defaults for application settings and behavior. By following these conventions, developers can spend less time configuring their applications and more time focusing on the core functionality. This results in cleaner, more maintainable code.
Don't Repeat Yourself
The DRY principle encourages developers to write code that is reusable and modular. This helps eliminate duplication, making it easier to maintain and update your application over time.
Model-View-Controller (MVC) Architecture
Rails is built on the Model-View-Controller (MVC) architectural pattern. This pattern separates an application into three main components:
- Model: Represents the data and business logic of the application.
- View: Displays the data to the user in a human-readable format.
- Controller: Handles user interactions and processes requests, updating the model and view accordingly.
By adhering to the MVC pattern, Rails applications are organized and easy to navigate, making it simple for developers to understand the flow of data and functionality.
Getting Started with Rails
To begin developing with Rails, you'll need to have Ruby and the Rails gem installed on your machine. Once you've set up your development environment, you can create a new Rails application using the rails new
command. This command generates a boilerplate application with the necessary files and directories to get started.
Rails Generators
One of the key features that sets Rails apart from other web frameworks is its use of generators. Generators are command-line tools that automate the creation of common elements in a Rails application, such as models, controllers, and views. They follow Rails conventions, ensuring that your application is structured correctly and adheres to best practices.
ActiveRecord
ActiveRecord is Rails' Object-Relational Mapping (ORM) library. It provides a simple, intuitive interface for interacting with databases, allowing developers to work with data in their applications without needing to write complex SQL queries. ActiveRecord follows the Active Record pattern, representing database tables as classes and rows as instances of those classes.
Conclusion
Ruby on Rails is an excellent choice for web developers who value efficiency, organization, and adherence to best practices. With its powerful tools and conventions, Rails enables developers to create web applications quickly and maintainably. Whether you're building a simple blog or a complex, data-driven application, Rails has the tools and resources to make your project a success.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Ruby on Rails and why is it popular for web development?
Ruby on Rails, often simply called Rails, is a web application framework built on top of the Ruby programming language. It's popular for web development due to its emphasis on simplicity, convention over configuration, and rapid development. With Rails, developers can build feature-rich web applications more quickly and with less boilerplate code compared to other frameworks.
How does Ruby on Rails follow the principle of convention over configuration?
Convention over configuration is a design principle that Rails embraces to reduce the number of decisions a developer has to make by providing sensible defaults. Rails follows established conventions for things like file structure, naming, and database schema, which allows developers to focus on writing application logic rather than dealing with configuration settings. This leads to more efficient development and easier maintenance.
What is the Model-View-Controller (MVC) architecture in Rails?
Model-View-Controller (MVC) is a design pattern that separates an application's components into three distinct layers:
- Model: Represents the data and business logic of the application.
- View: Handles the presentation and user interface components.
- Controller: Manages the communication between the Model and View. Rails follows the MVC pattern, which helps in organizing code, improving maintainability, and enabling the reuse of components across the application.
Can you give a brief overview of the main components of a Rails application?
Sure! A Rails application typically consists of the following components:
- Models: Represent the application's data and business logic.
- Views: Handle the presentation layer and user interface elements, often using embedded Ruby (ERB) files or other templating languages.
- Controllers: Manage the flow of data between the Models and Views.
- Routes: Define the URL patterns and map them to specific controller actions.
- Configurations: Store various settings and configurations for the application.
- Assets: Include CSS, JavaScript, and image files that are used for the application's styling and interactivity.
- Database: Stores the data for the application, with Rails supporting various databases like PostgreSQL, MySQL, and SQLite.
How can I get started with Ruby on Rails?
To get started with Ruby on Rails, you need to have Ruby and Rails installed on your system. You can follow the official Rails installation guide at Install Rails. Once you have Rails installed, you can create a new Rails application by running the following command in your terminal:
rails new my_app
Replace my_app
with the name of your application. This command will generate a new Rails application with the default directory structure, which you can then run and start developing your web application.