Rust Traits Introduction
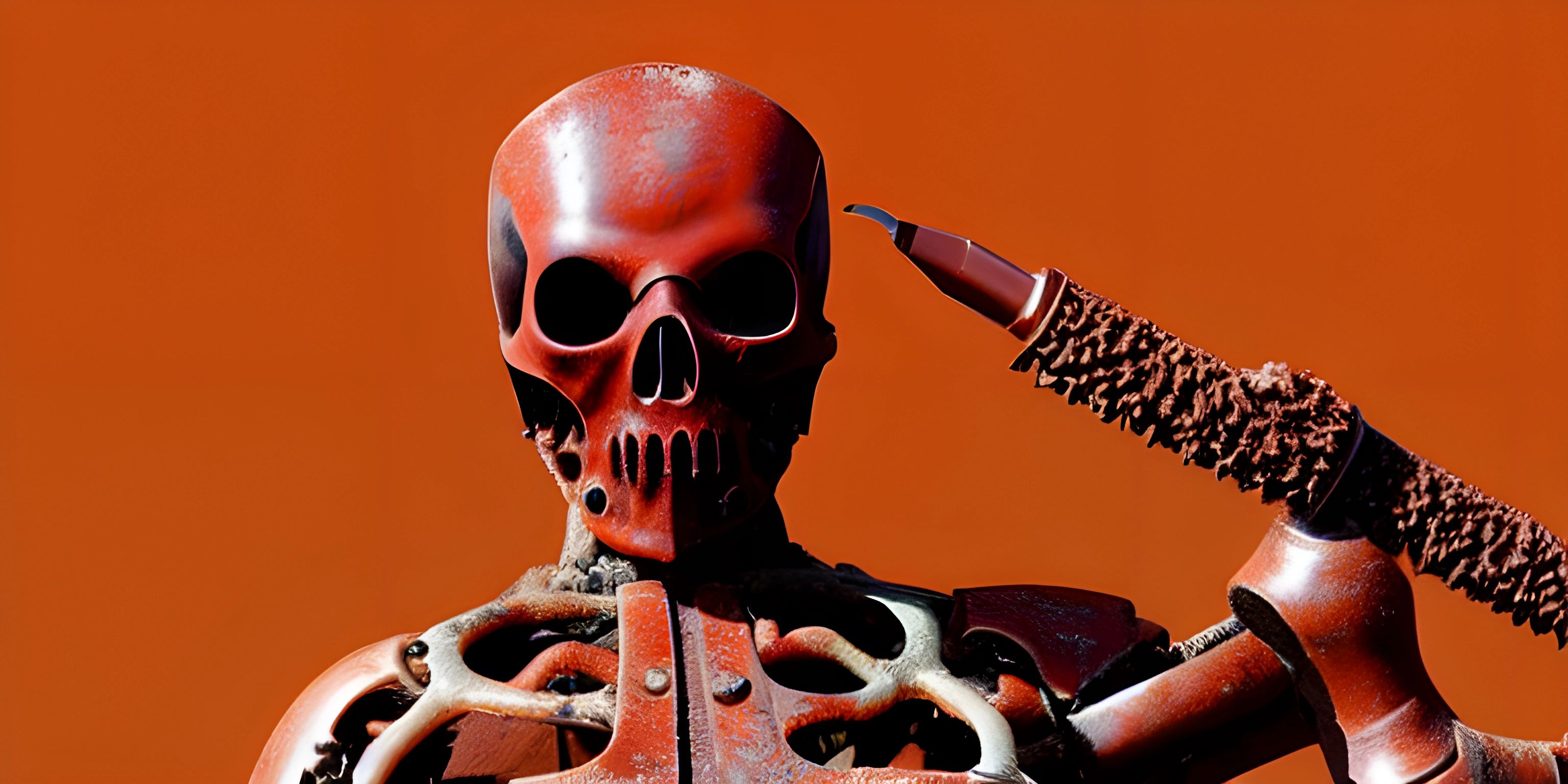
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Traits in Rust are a powerful way to define shared behavior between different types. They allow you to express what a type can do without specifying how it should do it. In this article, we'll explore the basics of Rust traits, how to implement them, and how they can be used to improve code organization and reusability.
What are Rust Traits?
Traits are a fundamental concept in Rust's type system. They serve as a way to define interfaces or contracts that types must adhere to, ensuring consistency and readability in your code. Simply put, a trait is a collection of methods that can be implemented by any type.
Imagine you're building a game with various characters that can perform different actions. Although each character may perform these actions differently, you want to ensure that they all have a consistent set of actions. This is where Rust traits come in handy.
To define a trait, we use the trait
keyword:
trait Character { fn attack(&self); fn defend(&self); }
Here, we've defined a Character
trait with two methods: attack
and defend
. Now any type that implements this trait will need to provide an implementation for these methods.
Implementing Traits
To implement a trait for a type, we use the impl
keyword followed by the trait name and the type name. Let's create a Knight
and Archer
struct and implement the Character
trait for both of them:
struct Knight { name: String, } struct Archer { name: String, } impl Character for Knight { fn attack(&self) { println!("{} swings their sword!", self.name); } fn defend(&self) { println!("{} raises their shield!", self.name); } } impl Character for Archer { fn attack(&self) { println!("{} shoots an arrow!", self.name); } fn defend(&self) { println!("{} dodges the attack!", self.name); } }
Now both Knight
and Archer
implement the Character
trait and provide their own implementation for the attack
and defend
methods.
Trait Bounds
Rust allows you to specify trait bounds on generic functions or structs, ensuring that the types used adhere to the specified traits. This allows you to write more flexible and reusable code.
For example, let's create a function that allows any Character
to perform a combo attack:
fn perform_combo<T: Character>(character: &T) { character.attack(); character.defend(); character.attack(); } let knight = Knight { name: String::from("Lancelot") }; let archer = Archer { name: String::from("Robin") }; perform_combo(&knight); // Lancelot swings their sword! Lancelot raises their shield! Lancelot swings their sword! perform_combo(&archer); // Robin shoots an arrow! Robin dodges the attack! Robin shoots an arrow!
In the function definition, we used the syntax <T: Character>
to indicate that T
must implement the Character
trait.
Summary
Rust traits provide a powerful way to define shared behavior between different types. They help improve code organization and reusability by allowing you to specify interfaces that types must adhere to. In this article, we've covered the basics of defining traits, implementing them for different types, and using trait bounds for more flexible and reusable code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What are Rust traits?
Rust traits are a way to define shared behavior between different types. They serve as interfaces or contracts that types must adhere to, ensuring consistency and readability in your code. Traits are a collection of methods that can be implemented by any type.
How do you implement a trait for a type?
To implement a trait for a type, use the impl
keyword followed by the trait name and the type name. Then, provide an implementation for each method specified in the trait.
What are trait bounds?
Trait bounds are constraints placed on generic functions or structs, ensuring that the types used adhere to the specified traits. This allows you to write more flexible and reusable code by ensuring that only types compatible with a certain trait can be used in certain contexts.