Introduction to Vuex
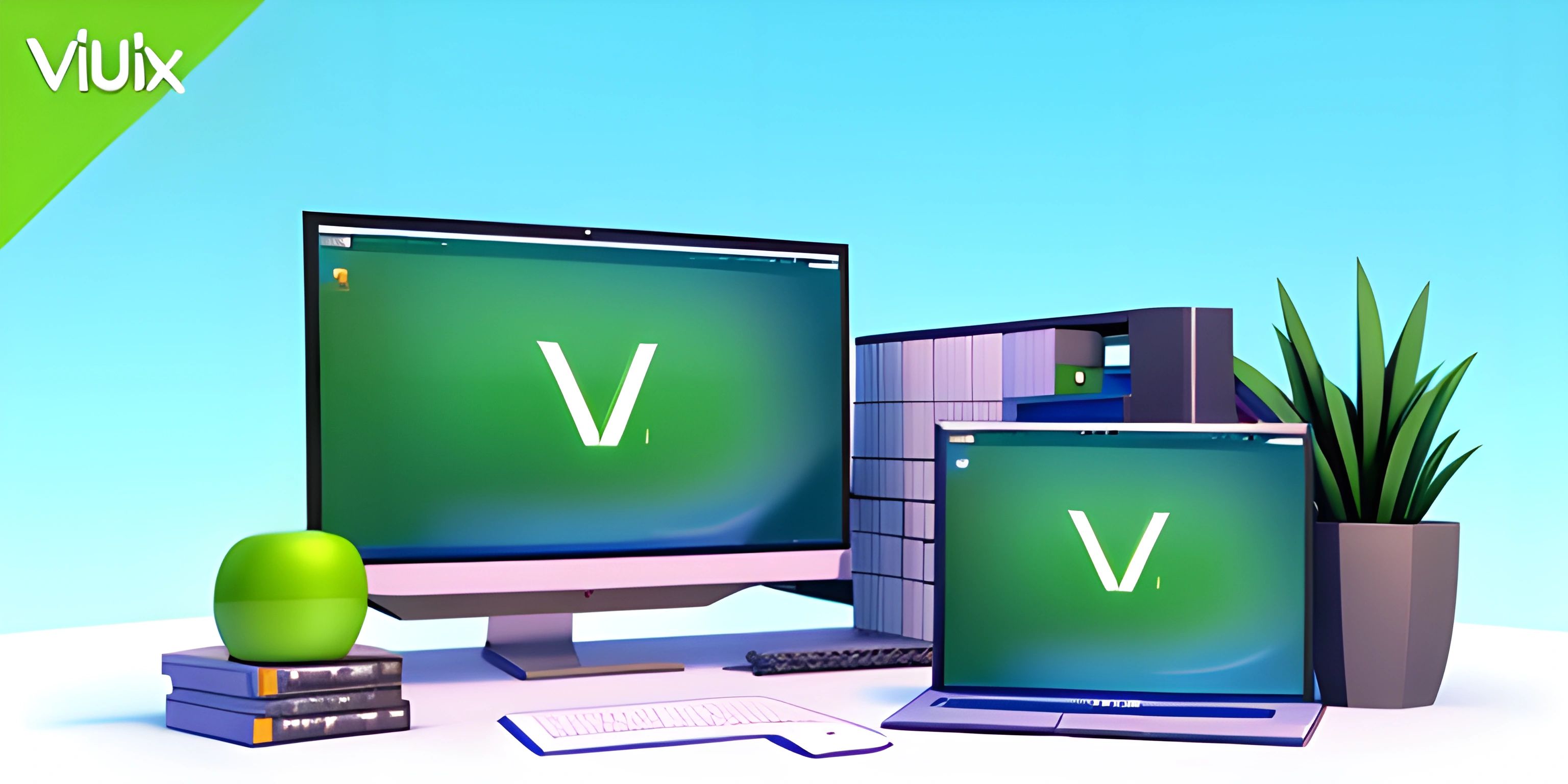
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Managing application state can be a complex task, especially in large-scale applications. That's where Vuex comes in. Vuex is a centralized state management library for Vue.js applications, which helps you build maintainable, scalable, and testable applications.
What is Vuex?
Vuex is a state management pattern and a library for managing state in Vue.js applications. It serves as a single source of truth for your application's data, making it easier to manage, debug, and understand the flow of data.
Core Concepts
There are four main concepts in Vuex:
- State: The single source of truth that stores the data for your application.
- Getters: Computed properties that are derived from the state and used to access state data.
- Mutations: Functions that directly modify the state. Mutations are synchronous and should be the only way to change the state.
- Actions: Functions that can perform asynchronous operations and commit mutations to change the state.
Installation
To get started with Vuex, you first need to install it using npm or yarn:
npm install vuex
or
yarn add vuex
Then, you need to import Vuex and create a store:
import Vue from "vue"; import Vuex from "vuex"; Vue.use(Vuex); const store = new Vuex.Store({ state: { count: 0 }, mutations: { increment(state) { state.count++; } } });
Now, you can use the store in your Vue application:
import Vue from "vue"; import App from "./App.vue"; import store from "./store"; new Vue({ store, render: (h) => h(App) }).$mount("#app");
Using Vuex in Components
You can access the store's state and commit mutations from your Vue components:
<template> <div> <p>The count is: {{ count }}</p> <button @click="increment">Increment</button> </div> </template> <script> export default { computed: { count() { return this.$store.state.count; } }, methods: { increment() { this.$store.commit("increment"); } } }; </script>
Benefits of Vuex
Using Vuex in your Vue applications has several benefits:
- Centralized State Management: Vuex provides a single source of truth for your application's state, making it easier to manage and understand.
- Debugging: With Vuex, you can track every state change, making it easier to debug and trace the flow of data in your application.
- Scalability: Vuex is designed to handle large-scale applications, providing a solid foundation for managing complex state.
- Testing: Vuex makes it easier to test your components, as you can mock the store and test how your components interact with it.
Overall, Vuex is a powerful tool for managing state in Vue.js applications, helping you build maintainable, scalable, and testable applications.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Vuex?
Vuex is a centralized state management library for Vue.js applications, which helps you build maintainable, scalable, and testable applications. It serves as a single source of truth for your application's data, making it easier to manage, debug, and understand the flow of data.
What are the core concepts of Vuex?
There are four main concepts in Vuex: 1) State, which stores the data for your application; 2) Getters, computed properties that access state data; 3) Mutations, functions that directly modify the state; and 4) Actions, functions that perform asynchronous operations and commit mutations to change the state.
How do you install and use Vuex in a Vue application?
To install Vuex, use npm or yarn to add it to your project (npm install vuex
or yarn add vuex
). Then, import Vuex, create a store, and use that store in your Vue application. Finally, access the store's state and commit mutations from your Vue components.