Introduction to the Standard Template Library in C++
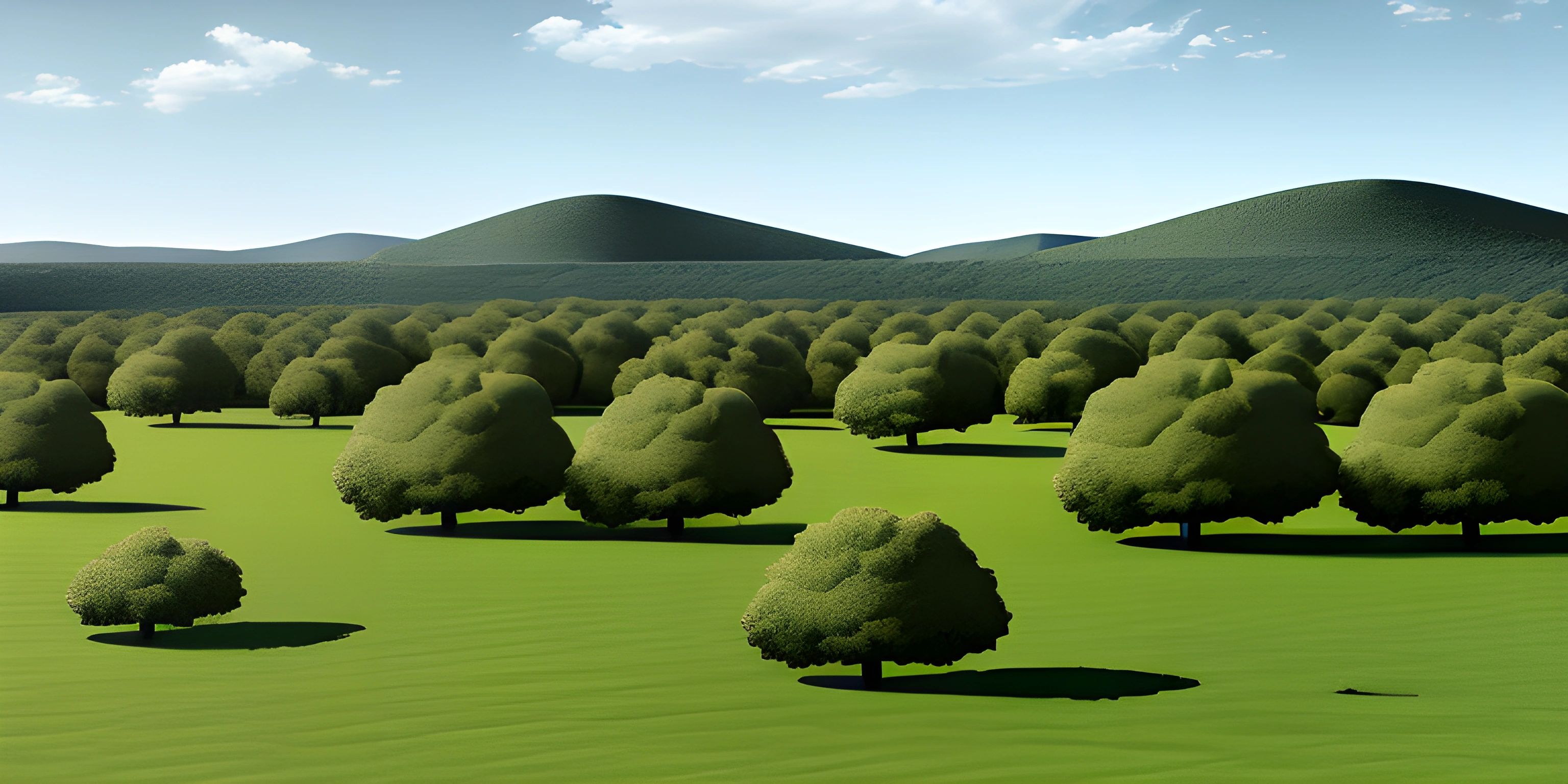
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
You might have heard the phrase "don't reinvent the wheel". In programming, this means leveraging existing solutions instead of writing everything from scratch. C++ developers have a powerful tool called the Standard Template Library (STL) that does just that! Let's dive into what the STL is and how it can make your C++ programming life easier.
What is the Standard Template Library?
The Standard Template Library is a collection of classes and functions designed to kick-start your C++ development by providing ready-made and efficient solutions for common programming tasks. The STL saves you time and effort by offering data structures, algorithms, and iterators that are both generic and reusable.
The STL can be broken down into three main components:
-
Containers: These are data structures that store data. Containers such as
vector
,list
,set
, andmap
help you organize and manage your data more efficiently. -
Algorithms: These are pre-built functions that perform common operations on your data. Examples include searching, sorting, and modifying elements.
-
Iterators: Iterators act as a bridge between containers and algorithms. They allow you to traverse through container elements and manipulate data using algorithms.
Containers
Containers in the STL are objects that store and organize data. They come in different flavors, each with its own advantages and trade-offs. Some popular container types include:
vector
: A dynamic array that automatically resizes and provides fast access to elements.list
: A doubly-linked list that allows for quick insertions and deletions.set
: An ordered collection of unique elements, optimized for searching.map
: An associative array that stores key-value pairs for efficient data retrieval.
To use a container, you must #include
the appropriate header file. For example, to use a vector
, you would include the following line at the beginning of your program:
#include <vector>
Algorithms
Algorithms are functions that perform operations on data stored in containers. They provide a high level of abstraction, allowing you to focus on the task at hand rather than getting bogged down in implementation details.
Some common algorithm operations include:
sort
: Sorts elements in a container.find
: Searches for an element in a container.reverse
: Reverses the order of elements in a container.copy
: Copies elements from one container to another.
To use an algorithm, you must include the <algorithm>
header:
#include <algorithm>
Iterators
Iterators are objects that allow you to traverse and access elements within a container. They provide a common interface for various container types, enabling you to use the same code for different data structures.
Iterators are often used in conjunction with algorithms. For example, when sorting a vector
, you would pass the iterators pointing to the beginning and end of the vector to the sort
algorithm:
#include <vector> #include <algorithm> int main() { std::vector<int> my_vector = {3, 1, 4, 1, 5, 9}; std::sort(my_vector.begin(), my_vector.end()); }
In this example, my_vector.begin()
and my_vector.end()
are iterators that point to the first and one-past-the-last elements of the vector
, respectively.
Conclusion
The Standard Template Library is a powerful and versatile tool for C++ developers. It provides you with pre-built, efficient, and reusable components that can significantly speed up your development process. By understanding and leveraging the STL's containers, algorithms, and iterators, you'll be able to write more efficient and cleaner code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Smiley Face (psst, it's free!).
FAQ
What is the Standard Template Library in C++?
The Standard Template Library (STL) in C++ is a collection of classes and functions that provide ready-made and efficient solutions for common programming tasks. It includes data structures, algorithms, and iterators to help you create efficient and reusable code.
What are the main components of the STL?
The three main components of the STL are containers, algorithms, and iterators. Containers are data structures that store data, algorithms are pre-built functions that perform common operations on data, and iterators are objects that help you traverse and access elements within containers.
How do I use a container from the STL?
To use a container from the STL, you need to #include
the appropriate header file in your program. For example, to use a vector
, you would include the following line at the beginning of your program: #include <vector>
.
How do I use an algorithm from the STL?
To use an algorithm from the STL, you need to include the <algorithm>
header in your program. Then, you can call the algorithm function and pass the required parameters, which often include iterators pointing to the beginning and end of the container you want to work with. For example, to sort a vector
, you would call std::sort(my_vector.begin(), my_vector.end());
.
What are iterators and why are they important in the STL?
Iterators are objects that allow you to traverse and access elements within a container. They provide a common interface for various container types, enabling you to use the same code for different data structures. Iterators are often used in conjunction with algorithms to manipulate and traverse the data within containers.