Understanding Static Typing in Programming Languages
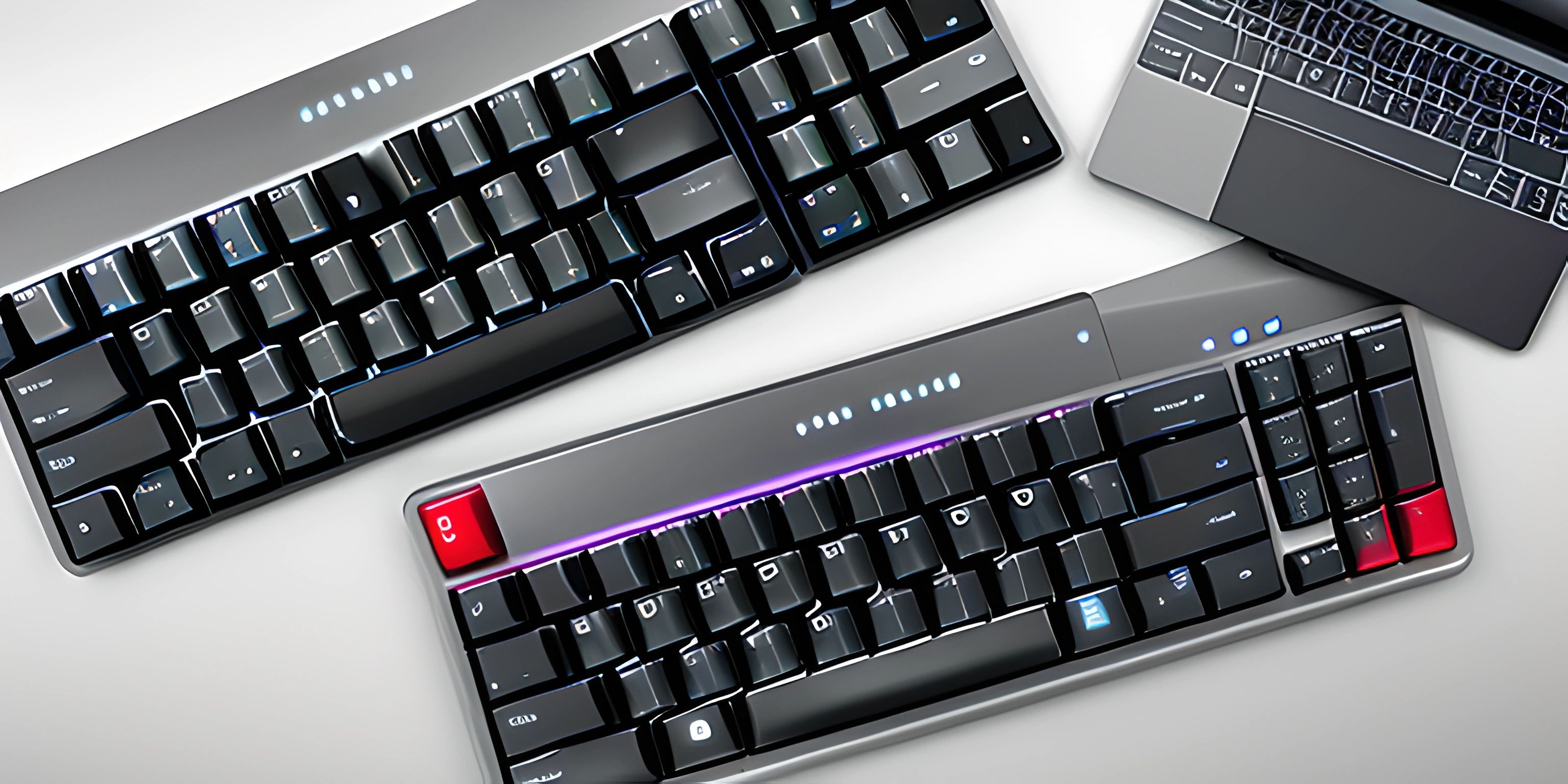
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Just like spoken languages, programming languages have different ways of expressing and managing data. One significant aspect is the way they handle types, which are the categories of values that can be stored and manipulated. In this article, we'll explore static typing, how it compares to dynamic typing, and the advantages it can bring to your code.
What is Static Typing?
Static typing is a property of programming languages where the type of a variable is determined at compile-time. This means that, before a program is executed, the compiler checks if the types of variables and expressions are consistent. If there's a type mismatch, it results in a compilation error, preventing the program from running.
Languages such as C, C++, Java, and Rust are examples of statically-typed languages. In these languages, you typically declare the type of a variable explicitly, like this (in C++):
int myNumber = 42; std::string myText = "Hello, world!";
Static Typing vs Dynamic Typing
The counterpart to static typing is dynamic typing. In dynamically-typed languages, the type of a variable is determined at runtime, and variables can change their type during the execution of the program. Examples of dynamically-typed languages include JavaScript, Python, and Ruby.
Here's a comparison of variable declaration in Python (dynamically-typed) and Java (statically-typed):
# Python (dynamic typing) myNumber = 42 myText = "Hello, world!"
// Java (static typing) int myNumber = 42; String myText = "Hello, world!";
An important difference is that, in dynamically-typed languages, type-related errors are usually detected at runtime, which can lead to unexpected behavior or crashes.
Advantages of Static Typing
There are several benefits to using a statically-typed language:
- Error detection: Since types are checked at compile-time, many errors can be caught early, before the program is executed. This can save time and effort during development and testing.
- Readability: Explicitly declaring types can make the code more readable, as it's clear what kind of data is expected in each variable or function.
- Performance: Statically-typed languages can be more efficient at runtime, as the compiler can optimize the code based on the known types, without the need for runtime type-checks.
However, statically-typed languages may require more verbose code, and some developers prefer the flexibility and conciseness of dynamically-typed languages. Ultimately, the choice depends on your project and personal preferences.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Advanced Data Types (psst, it's free!).
FAQ
What is static typing?
Static typing is a property of programming languages where the type of a variable is determined at compile-time, and type checking is performed before the program is executed.
How does static typing differ from dynamic typing?
In static typing, variable types are determined at compile-time, while in dynamic typing, variable types are determined at runtime. This means that static typing can catch type-related errors early in the development process, while dynamic typing may result in unexpected behavior or crashes during runtime.
What are some advantages of static typing?
Advantages of static typing include early error detection, improved readability of code, and better performance due to compile-time optimizations.