Binary Search: An Introduction
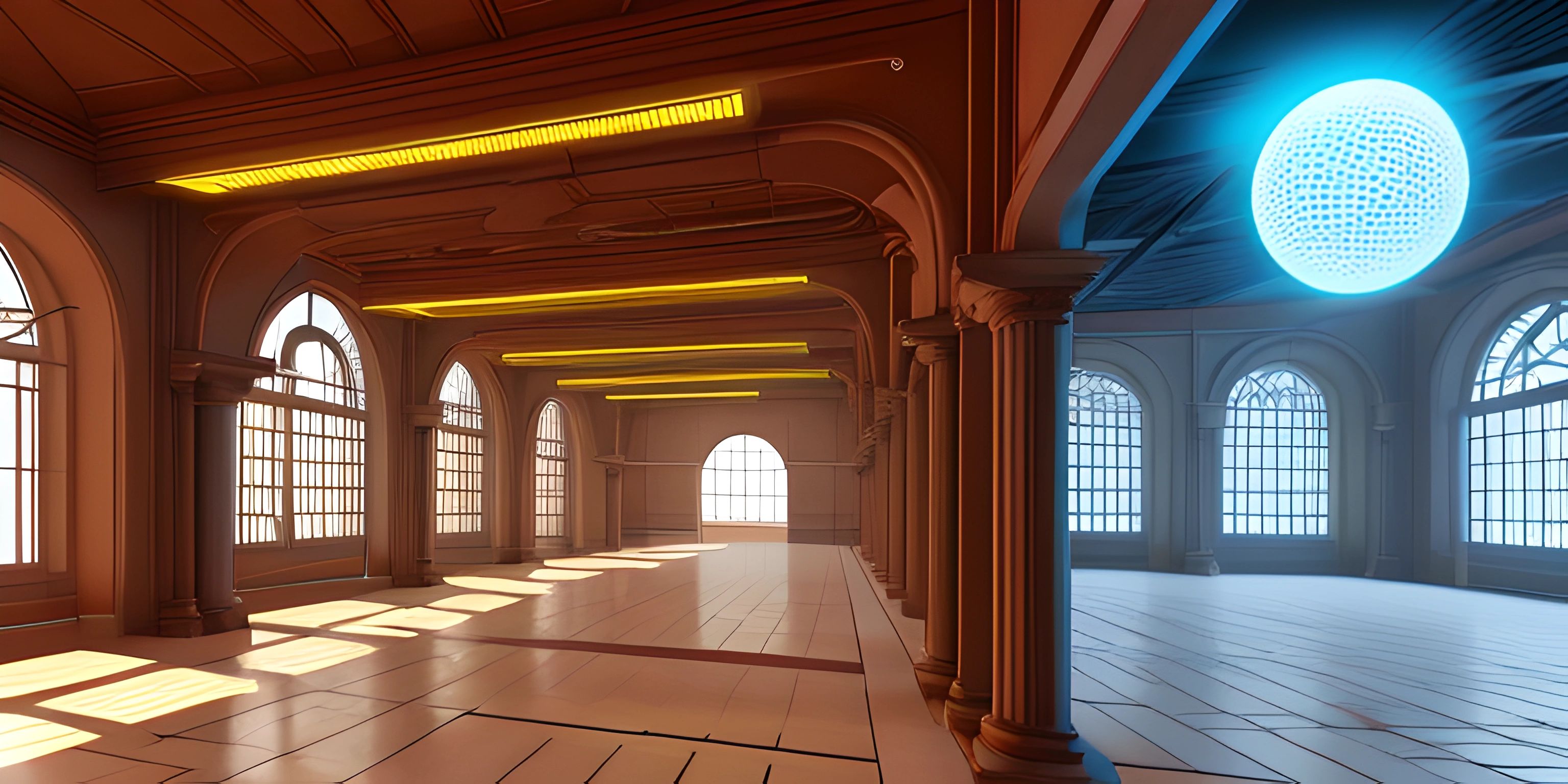
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming is filled with a plethora of algorithms, but one of the most efficient searching techniques is the binary search. It's like looking for a word in a dictionary, but for computers. So, buckle up and let's dive into the world of binary search!
What is Binary Search?
Binary search is an algorithm that efficiently searches for a specific value within a sorted array or list by repeatedly dividing it in half. If the desired value is less than the middle element, it searches in the left half, otherwise in the right half, until it finds the target value or exhausts the search space.
You may have used a similar process when searching for a name in a phone book (remember those?). Start at the middle, and if the name you're looking for is before the middle, you focus on the first half, otherwise, you check the second half. You keep narrowing down the search space until you find the name or realize it's not there.
Binary Search Algorithm
Here's a simple outline of the binary search algorithm:
- Set the lower and upper bounds of the search space (initially, the whole array).
- Calculate the middle index of the search space.
- Compare the middle element with the desired value.
- If the middle element is the desired value, you've found it!
- If the desired value is less than the middle element, update the upper bound to be one less than the middle index.
- If the desired value is greater than the middle element, update the lower bound to be one more than the middle index.
- Repeat steps 2-6 until you find the desired value or the lower bound is greater than the upper bound, meaning the value is not in the array.
Here's an example in Python:
def binary_search(arr, target): lower = 0 upper = len(arr) - 1 while lower <= upper: middle = (lower + upper) // 2 mid_val = arr[middle] if mid_val == target: return middle elif mid_val < target: lower = middle + 1 else: upper = middle - 1 return -1 # Target value not found
Use Cases
Binary search is a powerful algorithm that can be applied to various programming tasks, such as:
- Searching for a specific value in a large, sorted dataset.
- Finding the closest value to a given number in a sorted array.
- Determining the position of a new element to maintain a sorted order in a sorted array.
- Solving problems involving search spaces with monotonic properties, such as finding the square root of a number.
Binary search's efficiency comes from its O(log n) complexity, which means that for every doubling of the input size, it only takes one additional step to search for a value. This makes it an ideal choice when dealing with large datasets or when performance is a priority.
In Summary
Binary search is an efficient searching algorithm that saves time and resources by dividing and conquering sorted arrays or lists. Its wide range of use cases and performance benefits make it a valuable asset in any programmer's toolkit. Now that you're equipped with the knowledge of binary search, you're ready to employ this powerful algorithm in your own projects! Happy searching!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).